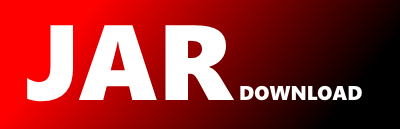
com.pulumi.aws.iam.kotlin.inputs.GetPrincipalPolicySimulationPlainArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iam.kotlin.inputs
import com.pulumi.aws.iam.inputs.GetPrincipalPolicySimulationPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getPrincipalPolicySimulation.
* @property actionNames A set of IAM action names to run simulations for. Each entry in this set adds an additional hypothetical request to the simulation.
* Action names consist of a service prefix and an action verb separated by a colon, such as `s3:GetObject`. Refer to [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html) to see the full set of possible IAM action names across all AWS services.
* @property additionalPoliciesJsons A set of additional principal policy documents to include in the simulation. The simulator will behave as if each of these policies were associated with the object specified in `policy_source_arn`, allowing you to test the effect of hypothetical policies not yet created.
* @property callerArn The ARN of an user that will appear as the "caller" of the simulated requests. If you do not specify `caller_arn` then the simulation will use the `policy_source_arn` instead, if it contains a user ARN.
* @property contexts Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
* @property permissionsBoundaryPoliciesJsons A set of [permissions boundary policy documents](https://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies_boundaries.html) to include in the simulation.
* @property policySourceArn The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM user, group, or role whose policies will be included in the simulation.
* You must closely match the form of the real service request you are simulating in order to achieve a realistic result. You can use the following additional arguments to specify other characteristics of the simulated requests:
* @property resourceArns A set of ARNs of resources to include in the simulation.
* This argument is important for actions that have either required or optional resource types listed in [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html), and you must provide ARNs that identify AWS objects of the appropriate types for the chosen actions.
* The policy simulator only automatically loads policies associated with the `policy_source_arn`, so if your given resources have their own resource-level policy then you'll also need to provide that explicitly using the `resource_policy_json` argument to achieve a realistic simulation.
* @property resourceHandlingOption Specifies a special simulation type to run. Some EC2 actions require special simulation behaviors and a particular set of resource ARNs to achieve a realistic result.
* For more details, see the `ResourceHandlingOption` request parameter for [the underlying `iam:SimulatePrincipalPolicy` action](https://docs.aws.amazon.com/IAM/latest/APIReference/API_SimulatePrincipalPolicy.html).
* @property resourceOwnerAccountId An AWS account ID to use for any resource ARN in `resource_arns` that doesn't include its own AWS account ID. If unspecified, the simulator will use the account ID from the `caller_arn` argument as a placeholder.
* @property resourcePolicyJson An IAM policy document representing the resource-level policy of all of the resources specified in `resource_arns`.
* The policy simulator cannot automatically load policies that are associated with individual resources, as described in the documentation for `resource_arns` above.
*/
public data class GetPrincipalPolicySimulationPlainArgs(
public val actionNames: List,
public val additionalPoliciesJsons: List? = null,
public val callerArn: String? = null,
public val contexts: List? = null,
public val permissionsBoundaryPoliciesJsons: List? = null,
public val policySourceArn: String,
public val resourceArns: List? = null,
public val resourceHandlingOption: String? = null,
public val resourceOwnerAccountId: String? = null,
public val resourcePolicyJson: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.iam.inputs.GetPrincipalPolicySimulationPlainArgs =
com.pulumi.aws.iam.inputs.GetPrincipalPolicySimulationPlainArgs.builder()
.actionNames(actionNames.let({ args0 -> args0.map({ args0 -> args0 }) }))
.additionalPoliciesJsons(additionalPoliciesJsons?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.callerArn(callerArn?.let({ args0 -> args0 }))
.contexts(contexts?.let({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.permissionsBoundaryPoliciesJsons(
permissionsBoundaryPoliciesJsons?.let({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.policySourceArn(policySourceArn.let({ args0 -> args0 }))
.resourceArns(resourceArns?.let({ args0 -> args0.map({ args0 -> args0 }) }))
.resourceHandlingOption(resourceHandlingOption?.let({ args0 -> args0 }))
.resourceOwnerAccountId(resourceOwnerAccountId?.let({ args0 -> args0 }))
.resourcePolicyJson(resourcePolicyJson?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetPrincipalPolicySimulationPlainArgs].
*/
@PulumiTagMarker
public class GetPrincipalPolicySimulationPlainArgsBuilder internal constructor() {
private var actionNames: List? = null
private var additionalPoliciesJsons: List? = null
private var callerArn: String? = null
private var contexts: List? = null
private var permissionsBoundaryPoliciesJsons: List? = null
private var policySourceArn: String? = null
private var resourceArns: List? = null
private var resourceHandlingOption: String? = null
private var resourceOwnerAccountId: String? = null
private var resourcePolicyJson: String? = null
/**
* @param value A set of IAM action names to run simulations for. Each entry in this set adds an additional hypothetical request to the simulation.
* Action names consist of a service prefix and an action verb separated by a colon, such as `s3:GetObject`. Refer to [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html) to see the full set of possible IAM action names across all AWS services.
*/
@JvmName("smqvvarfitgwxxuh")
public suspend fun actionNames(`value`: List) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.actionNames = mapped
}
/**
* @param values A set of IAM action names to run simulations for. Each entry in this set adds an additional hypothetical request to the simulation.
* Action names consist of a service prefix and an action verb separated by a colon, such as `s3:GetObject`. Refer to [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html) to see the full set of possible IAM action names across all AWS services.
*/
@JvmName("botstnqlyxyrsmxm")
public suspend fun actionNames(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.actionNames = mapped
}
/**
* @param value A set of additional principal policy documents to include in the simulation. The simulator will behave as if each of these policies were associated with the object specified in `policy_source_arn`, allowing you to test the effect of hypothetical policies not yet created.
*/
@JvmName("hjwwdnwqkpmotwfj")
public suspend fun additionalPoliciesJsons(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.additionalPoliciesJsons = mapped
}
/**
* @param values A set of additional principal policy documents to include in the simulation. The simulator will behave as if each of these policies were associated with the object specified in `policy_source_arn`, allowing you to test the effect of hypothetical policies not yet created.
*/
@JvmName("iltssarsamvcqvpt")
public suspend fun additionalPoliciesJsons(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.additionalPoliciesJsons = mapped
}
/**
* @param value The ARN of an user that will appear as the "caller" of the simulated requests. If you do not specify `caller_arn` then the simulation will use the `policy_source_arn` instead, if it contains a user ARN.
*/
@JvmName("dkwlnaictqtydffd")
public suspend fun callerArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.callerArn = mapped
}
/**
* @param value Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
*/
@JvmName("ttmfrsyclruuiqka")
public suspend fun contexts(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.contexts = mapped
}
/**
* @param argument Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
*/
@JvmName("jjgbeagdfdwatqki")
public suspend fun contexts(argument: List Unit>) {
val toBeMapped = argument.toList().map {
GetPrincipalPolicySimulationContextBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.contexts = mapped
}
/**
* @param argument Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
*/
@JvmName("xyidvugmxtxbboiv")
public suspend fun contexts(vararg argument: suspend GetPrincipalPolicySimulationContextBuilder.() -> Unit) {
val toBeMapped = argument.toList().map {
GetPrincipalPolicySimulationContextBuilder().applySuspend { it() }.build()
}
val mapped = toBeMapped
this.contexts = mapped
}
/**
* @param argument Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
*/
@JvmName("xyqbitfdnvfqiudv")
public suspend fun contexts(argument: suspend GetPrincipalPolicySimulationContextBuilder.() -> Unit) {
val toBeMapped = listOf(
GetPrincipalPolicySimulationContextBuilder().applySuspend {
argument()
}.build(),
)
val mapped = toBeMapped
this.contexts = mapped
}
/**
* @param values Each `context` block defines an entry in the table of additional context keys in the simulated request.
* IAM uses context keys for both custom conditions and for interpolating dynamic request-specific values into policy values. If you use policies that include those features then you will need to provide suitable example values for those keys to achieve a realistic simulation.
*/
@JvmName("eatajemyuumgifxr")
public suspend fun contexts(vararg values: GetPrincipalPolicySimulationContext) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.contexts = mapped
}
/**
* @param value A set of [permissions boundary policy documents](https://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies_boundaries.html) to include in the simulation.
*/
@JvmName("qedqdkirywsqnlgr")
public suspend fun permissionsBoundaryPoliciesJsons(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.permissionsBoundaryPoliciesJsons = mapped
}
/**
* @param values A set of [permissions boundary policy documents](https://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies_boundaries.html) to include in the simulation.
*/
@JvmName("ioauwsutwbkiotvg")
public suspend fun permissionsBoundaryPoliciesJsons(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.permissionsBoundaryPoliciesJsons = mapped
}
/**
* @param value The [ARN](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) of the IAM user, group, or role whose policies will be included in the simulation.
* You must closely match the form of the real service request you are simulating in order to achieve a realistic result. You can use the following additional arguments to specify other characteristics of the simulated requests:
*/
@JvmName("mbontgnghclokwam")
public suspend fun policySourceArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.policySourceArn = mapped
}
/**
* @param value A set of ARNs of resources to include in the simulation.
* This argument is important for actions that have either required or optional resource types listed in [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html), and you must provide ARNs that identify AWS objects of the appropriate types for the chosen actions.
* The policy simulator only automatically loads policies associated with the `policy_source_arn`, so if your given resources have their own resource-level policy then you'll also need to provide that explicitly using the `resource_policy_json` argument to achieve a realistic simulation.
*/
@JvmName("vxjaappodyikmwwh")
public suspend fun resourceArns(`value`: List?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resourceArns = mapped
}
/**
* @param values A set of ARNs of resources to include in the simulation.
* This argument is important for actions that have either required or optional resource types listed in [Actions, resources, and condition keys for AWS services](https://docs.aws.amazon.com/service-authorization/latest/reference/reference_policies_actions-resources-contextkeys.html), and you must provide ARNs that identify AWS objects of the appropriate types for the chosen actions.
* The policy simulator only automatically loads policies associated with the `policy_source_arn`, so if your given resources have their own resource-level policy then you'll also need to provide that explicitly using the `resource_policy_json` argument to achieve a realistic simulation.
*/
@JvmName("ykkuuljfpqbhxoqb")
public suspend fun resourceArns(vararg values: String) {
val toBeMapped = values.toList()
val mapped = toBeMapped.let({ args0 -> args0 })
this.resourceArns = mapped
}
/**
* @param value Specifies a special simulation type to run. Some EC2 actions require special simulation behaviors and a particular set of resource ARNs to achieve a realistic result.
* For more details, see the `ResourceHandlingOption` request parameter for [the underlying `iam:SimulatePrincipalPolicy` action](https://docs.aws.amazon.com/IAM/latest/APIReference/API_SimulatePrincipalPolicy.html).
*/
@JvmName("ucbwojmcchqfmkmd")
public suspend fun resourceHandlingOption(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resourceHandlingOption = mapped
}
/**
* @param value An AWS account ID to use for any resource ARN in `resource_arns` that doesn't include its own AWS account ID. If unspecified, the simulator will use the account ID from the `caller_arn` argument as a placeholder.
*/
@JvmName("xstqysrmigeeaqlm")
public suspend fun resourceOwnerAccountId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resourceOwnerAccountId = mapped
}
/**
* @param value An IAM policy document representing the resource-level policy of all of the resources specified in `resource_arns`.
* The policy simulator cannot automatically load policies that are associated with individual resources, as described in the documentation for `resource_arns` above.
*/
@JvmName("pggngqwvpyyojtvm")
public suspend fun resourcePolicyJson(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.resourcePolicyJson = mapped
}
internal fun build(): GetPrincipalPolicySimulationPlainArgs =
GetPrincipalPolicySimulationPlainArgs(
actionNames = actionNames ?: throw PulumiNullFieldException("actionNames"),
additionalPoliciesJsons = additionalPoliciesJsons,
callerArn = callerArn,
contexts = contexts,
permissionsBoundaryPoliciesJsons = permissionsBoundaryPoliciesJsons,
policySourceArn = policySourceArn ?: throw PulumiNullFieldException("policySourceArn"),
resourceArns = resourceArns,
resourceHandlingOption = resourceHandlingOption,
resourceOwnerAccountId = resourceOwnerAccountId,
resourcePolicyJson = resourcePolicyJson,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy