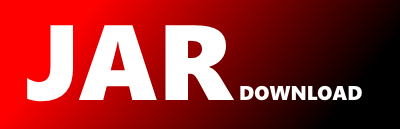
com.pulumi.aws.identitystore.kotlin.User.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.identitystore.kotlin
import com.pulumi.aws.identitystore.kotlin.outputs.UserAddresses
import com.pulumi.aws.identitystore.kotlin.outputs.UserEmails
import com.pulumi.aws.identitystore.kotlin.outputs.UserExternalId
import com.pulumi.aws.identitystore.kotlin.outputs.UserName
import com.pulumi.aws.identitystore.kotlin.outputs.UserPhoneNumbers
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.aws.identitystore.kotlin.outputs.UserAddresses.Companion.toKotlin as userAddressesToKotlin
import com.pulumi.aws.identitystore.kotlin.outputs.UserEmails.Companion.toKotlin as userEmailsToKotlin
import com.pulumi.aws.identitystore.kotlin.outputs.UserExternalId.Companion.toKotlin as userExternalIdToKotlin
import com.pulumi.aws.identitystore.kotlin.outputs.UserName.Companion.toKotlin as userNameToKotlin
import com.pulumi.aws.identitystore.kotlin.outputs.UserPhoneNumbers.Companion.toKotlin as userPhoneNumbersToKotlin
/**
* Builder for [User].
*/
@PulumiTagMarker
public class UserResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserArgs = UserArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserArgsBuilder.() -> Unit) {
val builder = UserArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): User {
val builtJavaResource = com.pulumi.aws.identitystore.User(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return User(builtJavaResource)
}
}
/**
* This resource manages a User resource within an Identity Store.
* > **Note:** If you use an external identity provider or Active Directory as your identity source,
* use this resource with caution. IAM Identity Center does not support outbound synchronization,
* so your identity source does not automatically update with the changes that you make to
* users using this resource.
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.identitystore.User("example", {
* identityStoreId: exampleAwsSsoadminInstances.identityStoreIds[0],
* displayName: "John Doe",
* userName: "johndoe",
* name: {
* givenName: "John",
* familyName: "Doe",
* },
* emails: {
* value: "[email protected]",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.identitystore.User("example",
* identity_store_id=example_aws_ssoadmin_instances["identityStoreIds"],
* display_name="John Doe",
* user_name="johndoe",
* name={
* "given_name": "John",
* "family_name": "Doe",
* },
* emails={
* "value": "[email protected]",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.IdentityStore.User("example", new()
* {
* IdentityStoreId = exampleAwsSsoadminInstances.IdentityStoreIds[0],
* DisplayName = "John Doe",
* UserName = "johndoe",
* Name = new Aws.IdentityStore.Inputs.UserNameArgs
* {
* GivenName = "John",
* FamilyName = "Doe",
* },
* Emails = new Aws.IdentityStore.Inputs.UserEmailsArgs
* {
* Value = "[email protected]",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/identitystore"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := identitystore.NewUser(ctx, "example", &identitystore.UserArgs{
* IdentityStoreId: pulumi.Any(exampleAwsSsoadminInstances.IdentityStoreIds[0]),
* DisplayName: pulumi.String("John Doe"),
* UserName: pulumi.String("johndoe"),
* Name: &identitystore.UserNameArgs{
* GivenName: pulumi.String("John"),
* FamilyName: pulumi.String("Doe"),
* },
* Emails: &identitystore.UserEmailsArgs{
* Value: pulumi.String("[email protected]"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.identitystore.User;
* import com.pulumi.aws.identitystore.UserArgs;
* import com.pulumi.aws.identitystore.inputs.UserNameArgs;
* import com.pulumi.aws.identitystore.inputs.UserEmailsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new User("example", UserArgs.builder()
* .identityStoreId(exampleAwsSsoadminInstances.identityStoreIds()[0])
* .displayName("John Doe")
* .userName("johndoe")
* .name(UserNameArgs.builder()
* .givenName("John")
* .familyName("Doe")
* .build())
* .emails(UserEmailsArgs.builder()
* .value("[email protected]")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:identitystore:User
* properties:
* identityStoreId: ${exampleAwsSsoadminInstances.identityStoreIds[0]}
* displayName: John Doe
* userName: johndoe
* name:
* givenName: John
* familyName: Doe
* emails:
* value: [email protected]
* ```
*
* ## Import
* Using `pulumi import`, import an Identity Store User using the combination `identity_store_id/user_id`. For example:
* ```sh
* $ pulumi import aws:identitystore/user:User example d-9c6705e95c/065212b4-9061-703b-5876-13a517ae2a7c
* ```
*/
public class User internal constructor(
override val javaResource: com.pulumi.aws.identitystore.User,
) : KotlinCustomResource(javaResource, UserMapper) {
/**
* Details about the user's address. At most 1 address is allowed. Detailed below.
*/
public val addresses: Output?
get() = javaResource.addresses().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
userAddressesToKotlin(args0)
})
}).orElse(null)
})
/**
* The name that is typically displayed when the user is referenced.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Details about the user's email. At most 1 email is allowed. Detailed below.
*/
public val emails: Output?
get() = javaResource.emails().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
userEmailsToKotlin(args0)
})
}).orElse(null)
})
/**
* A list of identifiers issued to this resource by an external identity provider.
*/
public val externalIds: Output>
get() = javaResource.externalIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
userExternalIdToKotlin(args0)
})
})
})
/**
* The globally unique identifier for the identity store that this user is in.
*/
public val identityStoreId: Output
get() = javaResource.identityStoreId().applyValue({ args0 -> args0 })
/**
* The user's geographical region or location.
*/
public val locale: Output?
get() = javaResource.locale().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Details about the user's full name. Detailed below.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0.let({ args0 -> userNameToKotlin(args0) }) })
/**
* An alternate name for the user.
*/
public val nickname: Output?
get() = javaResource.nickname().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Details about the user's phone number. At most 1 phone number is allowed. Detailed below.
*/
public val phoneNumbers: Output?
get() = javaResource.phoneNumbers().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
userPhoneNumbersToKotlin(args0)
})
}).orElse(null)
})
/**
* The preferred language of the user.
*/
public val preferredLanguage: Output?
get() = javaResource.preferredLanguage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* An URL that may be associated with the user.
*/
public val profileUrl: Output?
get() = javaResource.profileUrl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The user's time zone.
*/
public val timezone: Output?
get() = javaResource.timezone().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The user's title.
*/
public val title: Output?
get() = javaResource.title().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The identifier for this user in the identity store.
*/
public val userId: Output
get() = javaResource.userId().applyValue({ args0 -> args0 })
/**
* A unique string used to identify the user. This value can consist of letters, accented characters, symbols, numbers, and punctuation. This value is specified at the time the user is created and stored as an attribute of the user object in the identity store. The limit is 128 characters.
* The following arguments are optional:
*/
public val userName: Output
get() = javaResource.userName().applyValue({ args0 -> args0 })
/**
* The user type.
*/
public val userType: Output?
get() = javaResource.userType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object UserMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.aws.identitystore.User::class == javaResource::class
override fun map(javaResource: Resource): User = User(
javaResource as
com.pulumi.aws.identitystore.User,
)
}
/**
* @see [User].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [User].
*/
public suspend fun user(name: String, block: suspend UserResourceBuilder.() -> Unit): User {
val builder = UserResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [User].
* @param name The _unique_ name of the resulting resource.
*/
public fun user(name: String): User {
val builder = UserResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy