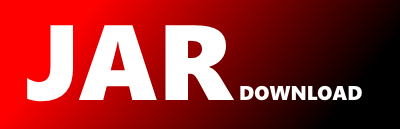
com.pulumi.aws.identitystore.kotlin.inputs.GetGroupPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.identitystore.kotlin.inputs
import com.pulumi.aws.identitystore.inputs.GetGroupPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getGroup.
* @property alternateIdentifier A unique identifier for the group that is not the primary identifier. Conflicts with `group_id` and `filter`. Detailed below.
* @property filter Configuration block for filtering by a unique attribute of the group. Detailed below.
* @property groupId The identifier for a group in the Identity Store.
* > Exactly one of the above arguments must be provided. Passing both `filter` and `group_id` is allowed for backwards compatibility.
* @property identityStoreId Identity Store ID associated with the Single Sign-On Instance.
* The following arguments are optional:
*/
public data class GetGroupPlainArgs(
public val alternateIdentifier: GetGroupAlternateIdentifier? = null,
@Deprecated(
message = """
Use the alternate_identifier attribute instead.
""",
)
public val filter: GetGroupFilter? = null,
public val groupId: String? = null,
public val identityStoreId: String,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.identitystore.inputs.GetGroupPlainArgs =
com.pulumi.aws.identitystore.inputs.GetGroupPlainArgs.builder()
.alternateIdentifier(alternateIdentifier?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.filter(filter?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.groupId(groupId?.let({ args0 -> args0 }))
.identityStoreId(identityStoreId.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetGroupPlainArgs].
*/
@PulumiTagMarker
public class GetGroupPlainArgsBuilder internal constructor() {
private var alternateIdentifier: GetGroupAlternateIdentifier? = null
private var filter: GetGroupFilter? = null
private var groupId: String? = null
private var identityStoreId: String? = null
/**
* @param value A unique identifier for the group that is not the primary identifier. Conflicts with `group_id` and `filter`. Detailed below.
*/
@JvmName("stkaatbinwhkcncp")
public suspend fun alternateIdentifier(`value`: GetGroupAlternateIdentifier?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.alternateIdentifier = mapped
}
/**
* @param argument A unique identifier for the group that is not the primary identifier. Conflicts with `group_id` and `filter`. Detailed below.
*/
@JvmName("ufxqhlpjfrtioobl")
public suspend fun alternateIdentifier(argument: suspend GetGroupAlternateIdentifierBuilder.() -> Unit) {
val toBeMapped = GetGroupAlternateIdentifierBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.alternateIdentifier = mapped
}
/**
* @param value Configuration block for filtering by a unique attribute of the group. Detailed below.
*/
@Deprecated(
message = """
Use the alternate_identifier attribute instead.
""",
)
@JvmName("cxsowkwhyldauyof")
public suspend fun filter(`value`: GetGroupFilter?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.filter = mapped
}
/**
* @param argument Configuration block for filtering by a unique attribute of the group. Detailed below.
*/
@Deprecated(
message = """
Use the alternate_identifier attribute instead.
""",
)
@JvmName("jhnqxkicljgknubd")
public suspend fun filter(argument: suspend GetGroupFilterBuilder.() -> Unit) {
val toBeMapped = GetGroupFilterBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.filter = mapped
}
/**
* @param value The identifier for a group in the Identity Store.
* > Exactly one of the above arguments must be provided. Passing both `filter` and `group_id` is allowed for backwards compatibility.
*/
@JvmName("drptctrnxmfhthqp")
public suspend fun groupId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.groupId = mapped
}
/**
* @param value Identity Store ID associated with the Single Sign-On Instance.
* The following arguments are optional:
*/
@JvmName("uhekuhuuvvileemn")
public suspend fun identityStoreId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.identityStoreId = mapped
}
internal fun build(): GetGroupPlainArgs = GetGroupPlainArgs(
alternateIdentifier = alternateIdentifier,
filter = filter,
groupId = groupId,
identityStoreId = identityStoreId ?: throw PulumiNullFieldException("identityStoreId"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy