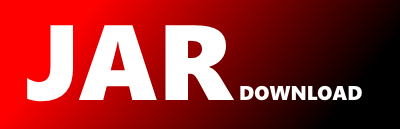
com.pulumi.aws.imagebuilder.kotlin.ContainerRecipeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.imagebuilder.kotlin
import com.pulumi.aws.imagebuilder.ContainerRecipeArgs.builder
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeComponentArgs
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeComponentArgsBuilder
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeInstanceConfigurationArgs
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeInstanceConfigurationArgsBuilder
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeTargetRepositoryArgs
import com.pulumi.aws.imagebuilder.kotlin.inputs.ContainerRecipeTargetRepositoryArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages an Image Builder Container Recipe.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.imagebuilder.ContainerRecipe("example", {
* name: "example",
* version: "1.0.0",
* containerType: "DOCKER",
* parentImage: "arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x",
* targetRepository: {
* repositoryName: exampleAwsEcrRepository.name,
* service: "ECR",
* },
* components: [{
* componentArn: exampleAwsImagebuilderComponent.arn,
* parameters: [
* {
* name: "Parameter1",
* value: "Value1",
* },
* {
* name: "Parameter2",
* value: "Value2",
* },
* ],
* }],
* dockerfileTemplateData: `FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.imagebuilder.ContainerRecipe("example",
* name="example",
* version="1.0.0",
* container_type="DOCKER",
* parent_image="arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x",
* target_repository={
* "repository_name": example_aws_ecr_repository["name"],
* "service": "ECR",
* },
* components=[{
* "component_arn": example_aws_imagebuilder_component["arn"],
* "parameters": [
* {
* "name": "Parameter1",
* "value": "Value1",
* },
* {
* "name": "Parameter2",
* "value": "Value2",
* },
* ],
* }],
* dockerfile_template_data="""FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ImageBuilder.ContainerRecipe("example", new()
* {
* Name = "example",
* Version = "1.0.0",
* ContainerType = "DOCKER",
* ParentImage = "arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x",
* TargetRepository = new Aws.ImageBuilder.Inputs.ContainerRecipeTargetRepositoryArgs
* {
* RepositoryName = exampleAwsEcrRepository.Name,
* Service = "ECR",
* },
* Components = new[]
* {
* new Aws.ImageBuilder.Inputs.ContainerRecipeComponentArgs
* {
* ComponentArn = exampleAwsImagebuilderComponent.Arn,
* Parameters = new[]
* {
* new Aws.ImageBuilder.Inputs.ContainerRecipeComponentParameterArgs
* {
* Name = "Parameter1",
* Value = "Value1",
* },
* new Aws.ImageBuilder.Inputs.ContainerRecipeComponentParameterArgs
* {
* Name = "Parameter2",
* Value = "Value2",
* },
* },
* },
* },
* DockerfileTemplateData = @"FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/imagebuilder"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := imagebuilder.NewContainerRecipe(ctx, "example", &imagebuilder.ContainerRecipeArgs{
* Name: pulumi.String("example"),
* Version: pulumi.String("1.0.0"),
* ContainerType: pulumi.String("DOCKER"),
* ParentImage: pulumi.String("arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x"),
* TargetRepository: &imagebuilder.ContainerRecipeTargetRepositoryArgs{
* RepositoryName: pulumi.Any(exampleAwsEcrRepository.Name),
* Service: pulumi.String("ECR"),
* },
* Components: imagebuilder.ContainerRecipeComponentArray{
* &imagebuilder.ContainerRecipeComponentArgs{
* ComponentArn: pulumi.Any(exampleAwsImagebuilderComponent.Arn),
* Parameters: imagebuilder.ContainerRecipeComponentParameterArray{
* &imagebuilder.ContainerRecipeComponentParameterArgs{
* Name: pulumi.String("Parameter1"),
* Value: pulumi.String("Value1"),
* },
* &imagebuilder.ContainerRecipeComponentParameterArgs{
* Name: pulumi.String("Parameter2"),
* Value: pulumi.String("Value2"),
* },
* },
* },
* },
* DockerfileTemplateData: pulumi.String("FROM {{{ imagebuilder:parentImage }}}\n{{{ imagebuilder:environments }}}\n{{{ imagebuilder:components }}}\n"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.imagebuilder.ContainerRecipe;
* import com.pulumi.aws.imagebuilder.ContainerRecipeArgs;
* import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeTargetRepositoryArgs;
* import com.pulumi.aws.imagebuilder.inputs.ContainerRecipeComponentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ContainerRecipe("example", ContainerRecipeArgs.builder()
* .name("example")
* .version("1.0.0")
* .containerType("DOCKER")
* .parentImage("arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x")
* .targetRepository(ContainerRecipeTargetRepositoryArgs.builder()
* .repositoryName(exampleAwsEcrRepository.name())
* .service("ECR")
* .build())
* .components(ContainerRecipeComponentArgs.builder()
* .componentArn(exampleAwsImagebuilderComponent.arn())
* .parameters(
* ContainerRecipeComponentParameterArgs.builder()
* .name("Parameter1")
* .value("Value1")
* .build(),
* ContainerRecipeComponentParameterArgs.builder()
* .name("Parameter2")
* .value("Value2")
* .build())
* .build())
* .dockerfileTemplateData("""
* FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:imagebuilder:ContainerRecipe
* properties:
* name: example
* version: 1.0.0
* containerType: DOCKER
* parentImage: arn:aws:imagebuilder:eu-central-1:aws:image/amazon-linux-x86-latest/x.x.x
* targetRepository:
* repositoryName: ${exampleAwsEcrRepository.name}
* service: ECR
* components:
* - componentArn: ${exampleAwsImagebuilderComponent.arn}
* parameters:
* - name: Parameter1
* value: Value1
* - name: Parameter2
* value: Value2
* dockerfileTemplateData: |
* FROM {{{ imagebuilder:parentImage }}}
* {{{ imagebuilder:environments }}}
* {{{ imagebuilder:components }}}
* ```
*
* ## Import
* Using `pulumi import`, import `aws_imagebuilder_container_recipe` resources using the Amazon Resource Name (ARN). For example:
* ```sh
* $ pulumi import aws:imagebuilder/containerRecipe:ContainerRecipe example arn:aws:imagebuilder:us-east-1:123456789012:container-recipe/example/1.0.0
* ```
* @property components Ordered configuration block(s) with components for the container recipe. Detailed below.
* @property containerType The type of the container to create. Valid values: `DOCKER`.
* @property description The description of the container recipe.
* @property dockerfileTemplateData The Dockerfile template used to build the image as an inline data blob.
* @property dockerfileTemplateUri The Amazon S3 URI for the Dockerfile that will be used to build the container image.
* @property instanceConfiguration Configuration block used to configure an instance for building and testing container images. Detailed below.
* @property kmsKeyId The KMS key used to encrypt the container image.
* @property name The name of the container recipe.
* @property parentImage The base image for the container recipe.
* @property platformOverride Specifies the operating system platform when you use a custom base image.
* @property tags Key-value map of resource tags for the container recipe. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property targetRepository The destination repository for the container image. Detailed below.
* @property version Version of the container recipe.
* The following attributes are optional:
* @property workingDirectory The working directory to be used during build and test workflows.
*/
public data class ContainerRecipeArgs(
public val components: Output>? = null,
public val containerType: Output? = null,
public val description: Output? = null,
public val dockerfileTemplateData: Output? = null,
public val dockerfileTemplateUri: Output? = null,
public val instanceConfiguration: Output? = null,
public val kmsKeyId: Output? = null,
public val name: Output? = null,
public val parentImage: Output? = null,
public val platformOverride: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy