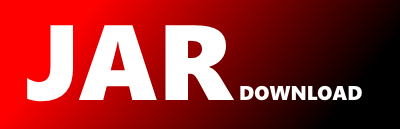
com.pulumi.aws.imagebuilder.kotlin.ImageRecipe.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.imagebuilder.kotlin
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeBlockDeviceMapping
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeComponent
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeSystemsManagerAgent
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeBlockDeviceMapping.Companion.toKotlin as imageRecipeBlockDeviceMappingToKotlin
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeComponent.Companion.toKotlin as imageRecipeComponentToKotlin
import com.pulumi.aws.imagebuilder.kotlin.outputs.ImageRecipeSystemsManagerAgent.Companion.toKotlin as imageRecipeSystemsManagerAgentToKotlin
/**
* Builder for [ImageRecipe].
*/
@PulumiTagMarker
public class ImageRecipeResourceBuilder internal constructor() {
public var name: String? = null
public var args: ImageRecipeArgs = ImageRecipeArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ImageRecipeArgsBuilder.() -> Unit) {
val builder = ImageRecipeArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ImageRecipe {
val builtJavaResource = com.pulumi.aws.imagebuilder.ImageRecipe(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ImageRecipe(builtJavaResource)
}
}
/**
* Manages an Image Builder Image Recipe.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.imagebuilder.ImageRecipe("example", {
* blockDeviceMappings: [{
* deviceName: "/dev/xvdb",
* ebs: {
* deleteOnTermination: "true",
* volumeSize: 100,
* volumeType: "gp2",
* },
* }],
* components: [{
* componentArn: exampleAwsImagebuilderComponent.arn,
* parameters: [
* {
* name: "Parameter1",
* value: "Value1",
* },
* {
* name: "Parameter2",
* value: "Value2",
* },
* ],
* }],
* name: "example",
* parentImage: `arn:${current.partition}:imagebuilder:${currentAwsRegion.name}:aws:image/amazon-linux-2-x86/x.x.x`,
* version: "1.0.0",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.imagebuilder.ImageRecipe("example",
* block_device_mappings=[{
* "device_name": "/dev/xvdb",
* "ebs": {
* "delete_on_termination": "true",
* "volume_size": 100,
* "volume_type": "gp2",
* },
* }],
* components=[{
* "component_arn": example_aws_imagebuilder_component["arn"],
* "parameters": [
* {
* "name": "Parameter1",
* "value": "Value1",
* },
* {
* "name": "Parameter2",
* "value": "Value2",
* },
* ],
* }],
* name="example",
* parent_image=f"arn:{current['partition']}:imagebuilder:{current_aws_region['name']}:aws:image/amazon-linux-2-x86/x.x.x",
* version="1.0.0")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ImageBuilder.ImageRecipe("example", new()
* {
* BlockDeviceMappings = new[]
* {
* new Aws.ImageBuilder.Inputs.ImageRecipeBlockDeviceMappingArgs
* {
* DeviceName = "/dev/xvdb",
* Ebs = new Aws.ImageBuilder.Inputs.ImageRecipeBlockDeviceMappingEbsArgs
* {
* DeleteOnTermination = "true",
* VolumeSize = 100,
* VolumeType = "gp2",
* },
* },
* },
* Components = new[]
* {
* new Aws.ImageBuilder.Inputs.ImageRecipeComponentArgs
* {
* ComponentArn = exampleAwsImagebuilderComponent.Arn,
* Parameters = new[]
* {
* new Aws.ImageBuilder.Inputs.ImageRecipeComponentParameterArgs
* {
* Name = "Parameter1",
* Value = "Value1",
* },
* new Aws.ImageBuilder.Inputs.ImageRecipeComponentParameterArgs
* {
* Name = "Parameter2",
* Value = "Value2",
* },
* },
* },
* },
* Name = "example",
* ParentImage = $"arn:{current.Partition}:imagebuilder:{currentAwsRegion.Name}:aws:image/amazon-linux-2-x86/x.x.x",
* Version = "1.0.0",
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/imagebuilder"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := imagebuilder.NewImageRecipe(ctx, "example", &imagebuilder.ImageRecipeArgs{
* BlockDeviceMappings: imagebuilder.ImageRecipeBlockDeviceMappingArray{
* &imagebuilder.ImageRecipeBlockDeviceMappingArgs{
* DeviceName: pulumi.String("/dev/xvdb"),
* Ebs: &imagebuilder.ImageRecipeBlockDeviceMappingEbsArgs{
* DeleteOnTermination: pulumi.String("true"),
* VolumeSize: pulumi.Int(100),
* VolumeType: pulumi.String("gp2"),
* },
* },
* },
* Components: imagebuilder.ImageRecipeComponentArray{
* &imagebuilder.ImageRecipeComponentArgs{
* ComponentArn: pulumi.Any(exampleAwsImagebuilderComponent.Arn),
* Parameters: imagebuilder.ImageRecipeComponentParameterArray{
* &imagebuilder.ImageRecipeComponentParameterArgs{
* Name: pulumi.String("Parameter1"),
* Value: pulumi.String("Value1"),
* },
* &imagebuilder.ImageRecipeComponentParameterArgs{
* Name: pulumi.String("Parameter2"),
* Value: pulumi.String("Value2"),
* },
* },
* },
* },
* Name: pulumi.String("example"),
* ParentImage: pulumi.Sprintf("arn:%v:imagebuilder:%v:aws:image/amazon-linux-2-x86/x.x.x", current.Partition, currentAwsRegion.Name),
* Version: pulumi.String("1.0.0"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.imagebuilder.ImageRecipe;
* import com.pulumi.aws.imagebuilder.ImageRecipeArgs;
* import com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingArgs;
* import com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingEbsArgs;
* import com.pulumi.aws.imagebuilder.inputs.ImageRecipeComponentArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ImageRecipe("example", ImageRecipeArgs.builder()
* .blockDeviceMappings(ImageRecipeBlockDeviceMappingArgs.builder()
* .deviceName("/dev/xvdb")
* .ebs(ImageRecipeBlockDeviceMappingEbsArgs.builder()
* .deleteOnTermination(true)
* .volumeSize(100)
* .volumeType("gp2")
* .build())
* .build())
* .components(ImageRecipeComponentArgs.builder()
* .componentArn(exampleAwsImagebuilderComponent.arn())
* .parameters(
* ImageRecipeComponentParameterArgs.builder()
* .name("Parameter1")
* .value("Value1")
* .build(),
* ImageRecipeComponentParameterArgs.builder()
* .name("Parameter2")
* .value("Value2")
* .build())
* .build())
* .name("example")
* .parentImage(String.format("arn:%s:imagebuilder:%s:aws:image/amazon-linux-2-x86/x.x.x", current.partition(),currentAwsRegion.name()))
* .version("1.0.0")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:imagebuilder:ImageRecipe
* properties:
* blockDeviceMappings:
* - deviceName: /dev/xvdb
* ebs:
* deleteOnTermination: true
* volumeSize: 100
* volumeType: gp2
* components:
* - componentArn: ${exampleAwsImagebuilderComponent.arn}
* parameters:
* - name: Parameter1
* value: Value1
* - name: Parameter2
* value: Value2
* name: example
* parentImage: arn:${current.partition}:imagebuilder:${currentAwsRegion.name}:aws:image/amazon-linux-2-x86/x.x.x
* version: 1.0.0
* ```
*
* ## Import
* Using `pulumi import`, import `aws_imagebuilder_image_recipe` resources using the Amazon Resource Name (ARN). For example:
* ```sh
* $ pulumi import aws:imagebuilder/imageRecipe:ImageRecipe example arn:aws:imagebuilder:us-east-1:123456789012:image-recipe/example/1.0.0
* ```
*/
public class ImageRecipe internal constructor(
override val javaResource: com.pulumi.aws.imagebuilder.ImageRecipe,
) : KotlinCustomResource(javaResource, ImageRecipeMapper) {
/**
* Amazon Resource Name (ARN) of the image recipe.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Configuration block(s) with block device mappings for the image recipe. Detailed below.
*/
public val blockDeviceMappings: Output>?
get() = javaResource.blockDeviceMappings().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
imageRecipeBlockDeviceMappingToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Ordered configuration block(s) with components for the image recipe. Detailed below.
*/
public val components: Output>
get() = javaResource.components().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
imageRecipeComponentToKotlin(args0)
})
})
})
/**
* Date the image recipe was created.
*/
public val dateCreated: Output
get() = javaResource.dateCreated().applyValue({ args0 -> args0 })
/**
* Description of the image recipe.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Name of the image recipe.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Owner of the image recipe.
*/
public val owner: Output
get() = javaResource.owner().applyValue({ args0 -> args0 })
/**
* The image recipe uses this image as a base from which to build your customized image. The value can be the base image ARN or an AMI ID.
*/
public val parentImage: Output
get() = javaResource.parentImage().applyValue({ args0 -> args0 })
/**
* Platform of the image recipe.
*/
public val platform: Output
get() = javaResource.platform().applyValue({ args0 -> args0 })
/**
* Configuration block for the Systems Manager Agent installed by default by Image Builder. Detailed below.
*/
public val systemsManagerAgent: Output
get() = javaResource.systemsManagerAgent().applyValue({ args0 ->
args0.let({ args0 ->
imageRecipeSystemsManagerAgentToKotlin(args0)
})
})
/**
* Key-value map of resource tags for the image recipe. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy