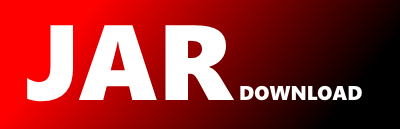
com.pulumi.aws.imagebuilder.kotlin.InfrastructureConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.imagebuilder.kotlin
import com.pulumi.aws.imagebuilder.InfrastructureConfigurationArgs.builder
import com.pulumi.aws.imagebuilder.kotlin.inputs.InfrastructureConfigurationInstanceMetadataOptionsArgs
import com.pulumi.aws.imagebuilder.kotlin.inputs.InfrastructureConfigurationInstanceMetadataOptionsArgsBuilder
import com.pulumi.aws.imagebuilder.kotlin.inputs.InfrastructureConfigurationLoggingArgs
import com.pulumi.aws.imagebuilder.kotlin.inputs.InfrastructureConfigurationLoggingArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages an Image Builder Infrastructure Configuration.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.imagebuilder.InfrastructureConfiguration("example", {
* description: "example description",
* instanceProfileName: exampleAwsIamInstanceProfile.name,
* instanceTypes: [
* "t2.nano",
* "t3.micro",
* ],
* keyPair: exampleAwsKeyPair.keyName,
* name: "example",
* securityGroupIds: [exampleAwsSecurityGroup.id],
* snsTopicArn: exampleAwsSnsTopic.arn,
* subnetId: main.id,
* terminateInstanceOnFailure: true,
* logging: {
* s3Logs: {
* s3BucketName: exampleAwsS3Bucket.bucket,
* s3KeyPrefix: "logs",
* },
* },
* tags: {
* foo: "bar",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.imagebuilder.InfrastructureConfiguration("example",
* description="example description",
* instance_profile_name=example_aws_iam_instance_profile["name"],
* instance_types=[
* "t2.nano",
* "t3.micro",
* ],
* key_pair=example_aws_key_pair["keyName"],
* name="example",
* security_group_ids=[example_aws_security_group["id"]],
* sns_topic_arn=example_aws_sns_topic["arn"],
* subnet_id=main["id"],
* terminate_instance_on_failure=True,
* logging={
* "s3_logs": {
* "s3_bucket_name": example_aws_s3_bucket["bucket"],
* "s3_key_prefix": "logs",
* },
* },
* tags={
* "foo": "bar",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.ImageBuilder.InfrastructureConfiguration("example", new()
* {
* Description = "example description",
* InstanceProfileName = exampleAwsIamInstanceProfile.Name,
* InstanceTypes = new[]
* {
* "t2.nano",
* "t3.micro",
* },
* KeyPair = exampleAwsKeyPair.KeyName,
* Name = "example",
* SecurityGroupIds = new[]
* {
* exampleAwsSecurityGroup.Id,
* },
* SnsTopicArn = exampleAwsSnsTopic.Arn,
* SubnetId = main.Id,
* TerminateInstanceOnFailure = true,
* Logging = new Aws.ImageBuilder.Inputs.InfrastructureConfigurationLoggingArgs
* {
* S3Logs = new Aws.ImageBuilder.Inputs.InfrastructureConfigurationLoggingS3LogsArgs
* {
* S3BucketName = exampleAwsS3Bucket.Bucket,
* S3KeyPrefix = "logs",
* },
* },
* Tags =
* {
* { "foo", "bar" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/imagebuilder"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := imagebuilder.NewInfrastructureConfiguration(ctx, "example", &imagebuilder.InfrastructureConfigurationArgs{
* Description: pulumi.String("example description"),
* InstanceProfileName: pulumi.Any(exampleAwsIamInstanceProfile.Name),
* InstanceTypes: pulumi.StringArray{
* pulumi.String("t2.nano"),
* pulumi.String("t3.micro"),
* },
* KeyPair: pulumi.Any(exampleAwsKeyPair.KeyName),
* Name: pulumi.String("example"),
* SecurityGroupIds: pulumi.StringArray{
* exampleAwsSecurityGroup.Id,
* },
* SnsTopicArn: pulumi.Any(exampleAwsSnsTopic.Arn),
* SubnetId: pulumi.Any(main.Id),
* TerminateInstanceOnFailure: pulumi.Bool(true),
* Logging: &imagebuilder.InfrastructureConfigurationLoggingArgs{
* S3Logs: &imagebuilder.InfrastructureConfigurationLoggingS3LogsArgs{
* S3BucketName: pulumi.Any(exampleAwsS3Bucket.Bucket),
* S3KeyPrefix: pulumi.String("logs"),
* },
* },
* Tags: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.imagebuilder.InfrastructureConfiguration;
* import com.pulumi.aws.imagebuilder.InfrastructureConfigurationArgs;
* import com.pulumi.aws.imagebuilder.inputs.InfrastructureConfigurationLoggingArgs;
* import com.pulumi.aws.imagebuilder.inputs.InfrastructureConfigurationLoggingS3LogsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new InfrastructureConfiguration("example", InfrastructureConfigurationArgs.builder()
* .description("example description")
* .instanceProfileName(exampleAwsIamInstanceProfile.name())
* .instanceTypes(
* "t2.nano",
* "t3.micro")
* .keyPair(exampleAwsKeyPair.keyName())
* .name("example")
* .securityGroupIds(exampleAwsSecurityGroup.id())
* .snsTopicArn(exampleAwsSnsTopic.arn())
* .subnetId(main.id())
* .terminateInstanceOnFailure(true)
* .logging(InfrastructureConfigurationLoggingArgs.builder()
* .s3Logs(InfrastructureConfigurationLoggingS3LogsArgs.builder()
* .s3BucketName(exampleAwsS3Bucket.bucket())
* .s3KeyPrefix("logs")
* .build())
* .build())
* .tags(Map.of("foo", "bar"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:imagebuilder:InfrastructureConfiguration
* properties:
* description: example description
* instanceProfileName: ${exampleAwsIamInstanceProfile.name}
* instanceTypes:
* - t2.nano
* - t3.micro
* keyPair: ${exampleAwsKeyPair.keyName}
* name: example
* securityGroupIds:
* - ${exampleAwsSecurityGroup.id}
* snsTopicArn: ${exampleAwsSnsTopic.arn}
* subnetId: ${main.id}
* terminateInstanceOnFailure: true
* logging:
* s3Logs:
* s3BucketName: ${exampleAwsS3Bucket.bucket}
* s3KeyPrefix: logs
* tags:
* foo: bar
* ```
*
* ## Import
* Using `pulumi import`, import `aws_imagebuilder_infrastructure_configuration` using the Amazon Resource Name (ARN). For example:
* ```sh
* $ pulumi import aws:imagebuilder/infrastructureConfiguration:InfrastructureConfiguration example arn:aws:imagebuilder:us-east-1:123456789012:infrastructure-configuration/example
* ```
* @property description Description for the configuration.
* @property instanceMetadataOptions Configuration block with instance metadata options for the HTTP requests that pipeline builds use to launch EC2 build and test instances. Detailed below.
* @property instanceProfileName Name of IAM Instance Profile.
* @property instanceTypes Set of EC2 Instance Types.
* @property keyPair Name of EC2 Key Pair.
* @property logging Configuration block with logging settings. Detailed below.
* @property name Name for the configuration.
* The following arguments are optional:
* @property resourceTags Key-value map of resource tags to assign to infrastructure created by the configuration.
* @property securityGroupIds Set of EC2 Security Group identifiers.
* @property snsTopicArn Amazon Resource Name (ARN) of SNS Topic.
* @property subnetId EC2 Subnet identifier. Also requires `security_group_ids` argument.
* @property tags Key-value map of resource tags to assign to the configuration. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
* @property terminateInstanceOnFailure Enable if the instance should be terminated when the pipeline fails. Defaults to `false`.
*/
public data class InfrastructureConfigurationArgs(
public val description: Output? = null,
public val instanceMetadataOptions: Output? = null,
public val instanceProfileName: Output? = null,
public val instanceTypes: Output>? = null,
public val keyPair: Output? = null,
public val logging: Output? = null,
public val name: Output? = null,
public val resourceTags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy