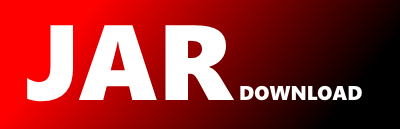
com.pulumi.aws.imagebuilder.kotlin.inputs.ImageRecipeBlockDeviceMappingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.imagebuilder.kotlin.inputs
import com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property deviceName Name of the device. For example, `/dev/sda` or `/dev/xvdb`.
* @property ebs Configuration block with Elastic Block Storage (EBS) block device mapping settings. Detailed below.
* @property noDevice Set to `true` to remove a mapping from the parent image.
* @property virtualName Virtual device name. For example, `ephemeral0`. Instance store volumes are numbered starting from 0.
*/
public data class ImageRecipeBlockDeviceMappingArgs(
public val deviceName: Output? = null,
public val ebs: Output? = null,
public val noDevice: Output? = null,
public val virtualName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingArgs =
com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingArgs.builder()
.deviceName(deviceName?.applyValue({ args0 -> args0 }))
.ebs(ebs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.noDevice(noDevice?.applyValue({ args0 -> args0 }))
.virtualName(virtualName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ImageRecipeBlockDeviceMappingArgs].
*/
@PulumiTagMarker
public class ImageRecipeBlockDeviceMappingArgsBuilder internal constructor() {
private var deviceName: Output? = null
private var ebs: Output? = null
private var noDevice: Output? = null
private var virtualName: Output? = null
/**
* @param value Name of the device. For example, `/dev/sda` or `/dev/xvdb`.
*/
@JvmName("xpfpxynquaemmmkd")
public suspend fun deviceName(`value`: Output) {
this.deviceName = value
}
/**
* @param value Configuration block with Elastic Block Storage (EBS) block device mapping settings. Detailed below.
*/
@JvmName("sidagwsjocfbtujo")
public suspend fun ebs(`value`: Output) {
this.ebs = value
}
/**
* @param value Set to `true` to remove a mapping from the parent image.
*/
@JvmName("wjkayhwaxjkovspy")
public suspend fun noDevice(`value`: Output) {
this.noDevice = value
}
/**
* @param value Virtual device name. For example, `ephemeral0`. Instance store volumes are numbered starting from 0.
*/
@JvmName("ufmlbeftpmeijnhr")
public suspend fun virtualName(`value`: Output) {
this.virtualName = value
}
/**
* @param value Name of the device. For example, `/dev/sda` or `/dev/xvdb`.
*/
@JvmName("mfmqnvjumxjdyvvv")
public suspend fun deviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deviceName = mapped
}
/**
* @param value Configuration block with Elastic Block Storage (EBS) block device mapping settings. Detailed below.
*/
@JvmName("jtwiglruvdgislgh")
public suspend fun ebs(`value`: ImageRecipeBlockDeviceMappingEbsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ebs = mapped
}
/**
* @param argument Configuration block with Elastic Block Storage (EBS) block device mapping settings. Detailed below.
*/
@JvmName("nkpavcelewyrexbw")
public suspend fun ebs(argument: suspend ImageRecipeBlockDeviceMappingEbsArgsBuilder.() -> Unit) {
val toBeMapped = ImageRecipeBlockDeviceMappingEbsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ebs = mapped
}
/**
* @param value Set to `true` to remove a mapping from the parent image.
*/
@JvmName("ynfeyqucwkfbktnd")
public suspend fun noDevice(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.noDevice = mapped
}
/**
* @param value Virtual device name. For example, `ephemeral0`. Instance store volumes are numbered starting from 0.
*/
@JvmName("oebclersgjwjukmj")
public suspend fun virtualName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.virtualName = mapped
}
internal fun build(): ImageRecipeBlockDeviceMappingArgs = ImageRecipeBlockDeviceMappingArgs(
deviceName = deviceName,
ebs = ebs,
noDevice = noDevice,
virtualName = virtualName,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy