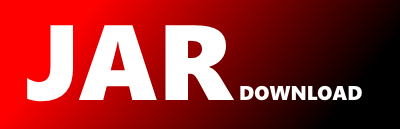
com.pulumi.aws.imagebuilder.kotlin.inputs.ImageRecipeBlockDeviceMappingEbsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.imagebuilder.kotlin.inputs
import com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingEbsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property deleteOnTermination Whether to delete the volume on termination. Defaults to unset, which is the value inherited from the parent image.
* @property encrypted Whether to encrypt the volume. Defaults to unset, which is the value inherited from the parent image.
* @property iops Number of Input/Output (I/O) operations per second to provision for an `io1` or `io2` volume.
* @property kmsKeyId Amazon Resource Name (ARN) of the Key Management Service (KMS) Key for encryption.
* @property snapshotId Identifier of the EC2 Volume Snapshot.
* @property throughput For GP3 volumes only. The throughput in MiB/s that the volume supports.
* @property volumeSize Size of the volume, in GiB.
* @property volumeType Type of the volume. For example, `gp2` or `io2`.
*/
public data class ImageRecipeBlockDeviceMappingEbsArgs(
public val deleteOnTermination: Output? = null,
public val encrypted: Output? = null,
public val iops: Output? = null,
public val kmsKeyId: Output? = null,
public val snapshotId: Output? = null,
public val throughput: Output? = null,
public val volumeSize: Output? = null,
public val volumeType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingEbsArgs =
com.pulumi.aws.imagebuilder.inputs.ImageRecipeBlockDeviceMappingEbsArgs.builder()
.deleteOnTermination(deleteOnTermination?.applyValue({ args0 -> args0 }))
.encrypted(encrypted?.applyValue({ args0 -> args0 }))
.iops(iops?.applyValue({ args0 -> args0 }))
.kmsKeyId(kmsKeyId?.applyValue({ args0 -> args0 }))
.snapshotId(snapshotId?.applyValue({ args0 -> args0 }))
.throughput(throughput?.applyValue({ args0 -> args0 }))
.volumeSize(volumeSize?.applyValue({ args0 -> args0 }))
.volumeType(volumeType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ImageRecipeBlockDeviceMappingEbsArgs].
*/
@PulumiTagMarker
public class ImageRecipeBlockDeviceMappingEbsArgsBuilder internal constructor() {
private var deleteOnTermination: Output? = null
private var encrypted: Output? = null
private var iops: Output? = null
private var kmsKeyId: Output? = null
private var snapshotId: Output? = null
private var throughput: Output? = null
private var volumeSize: Output? = null
private var volumeType: Output? = null
/**
* @param value Whether to delete the volume on termination. Defaults to unset, which is the value inherited from the parent image.
*/
@JvmName("dcnduhjeilbqnbdx")
public suspend fun deleteOnTermination(`value`: Output) {
this.deleteOnTermination = value
}
/**
* @param value Whether to encrypt the volume. Defaults to unset, which is the value inherited from the parent image.
*/
@JvmName("pdabndcxibtubiwb")
public suspend fun encrypted(`value`: Output) {
this.encrypted = value
}
/**
* @param value Number of Input/Output (I/O) operations per second to provision for an `io1` or `io2` volume.
*/
@JvmName("gotteioocvtjvosg")
public suspend fun iops(`value`: Output) {
this.iops = value
}
/**
* @param value Amazon Resource Name (ARN) of the Key Management Service (KMS) Key for encryption.
*/
@JvmName("ceeudgsrwqdlyooh")
public suspend fun kmsKeyId(`value`: Output) {
this.kmsKeyId = value
}
/**
* @param value Identifier of the EC2 Volume Snapshot.
*/
@JvmName("yggphdajccachtrr")
public suspend fun snapshotId(`value`: Output) {
this.snapshotId = value
}
/**
* @param value For GP3 volumes only. The throughput in MiB/s that the volume supports.
*/
@JvmName("jffnnqiavvxxacyw")
public suspend fun throughput(`value`: Output) {
this.throughput = value
}
/**
* @param value Size of the volume, in GiB.
*/
@JvmName("wracmhmwnhogxidj")
public suspend fun volumeSize(`value`: Output) {
this.volumeSize = value
}
/**
* @param value Type of the volume. For example, `gp2` or `io2`.
*/
@JvmName("xybxgxgaklggvcja")
public suspend fun volumeType(`value`: Output) {
this.volumeType = value
}
/**
* @param value Whether to delete the volume on termination. Defaults to unset, which is the value inherited from the parent image.
*/
@JvmName("wbqaiknnulvkjerl")
public suspend fun deleteOnTermination(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteOnTermination = mapped
}
/**
* @param value Whether to encrypt the volume. Defaults to unset, which is the value inherited from the parent image.
*/
@JvmName("yvdmwtoqlibcogmr")
public suspend fun encrypted(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encrypted = mapped
}
/**
* @param value Number of Input/Output (I/O) operations per second to provision for an `io1` or `io2` volume.
*/
@JvmName("ukxpenihahequxhc")
public suspend fun iops(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iops = mapped
}
/**
* @param value Amazon Resource Name (ARN) of the Key Management Service (KMS) Key for encryption.
*/
@JvmName("qsapfbebgxdscqtt")
public suspend fun kmsKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyId = mapped
}
/**
* @param value Identifier of the EC2 Volume Snapshot.
*/
@JvmName("mfpintwxirpanjqs")
public suspend fun snapshotId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotId = mapped
}
/**
* @param value For GP3 volumes only. The throughput in MiB/s that the volume supports.
*/
@JvmName("wufaieyfrqgtwwno")
public suspend fun throughput(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.throughput = mapped
}
/**
* @param value Size of the volume, in GiB.
*/
@JvmName("fufpxuwbstxmvmjb")
public suspend fun volumeSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeSize = mapped
}
/**
* @param value Type of the volume. For example, `gp2` or `io2`.
*/
@JvmName("uhukspaosxsoakdk")
public suspend fun volumeType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.volumeType = mapped
}
internal fun build(): ImageRecipeBlockDeviceMappingEbsArgs = ImageRecipeBlockDeviceMappingEbsArgs(
deleteOnTermination = deleteOnTermination,
encrypted = encrypted,
iops = iops,
kmsKeyId = kmsKeyId,
snapshotId = snapshotId,
throughput = throughput,
volumeSize = volumeSize,
volumeType = volumeType,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy