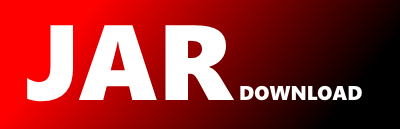
com.pulumi.aws.inspector.kotlin.AssessmentTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.inspector.kotlin
import com.pulumi.aws.inspector.AssessmentTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Provides an Inspector Classic Assessment Target
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const bar = new aws.inspector.ResourceGroup("bar", {tags: {
* Name: "foo",
* Env: "bar",
* }});
* const foo = new aws.inspector.AssessmentTarget("foo", {
* name: "assessment target",
* resourceGroupArn: bar.arn,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* bar = aws.inspector.ResourceGroup("bar", tags={
* "Name": "foo",
* "Env": "bar",
* })
* foo = aws.inspector.AssessmentTarget("foo",
* name="assessment target",
* resource_group_arn=bar.arn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var bar = new Aws.Inspector.ResourceGroup("bar", new()
* {
* Tags =
* {
* { "Name", "foo" },
* { "Env", "bar" },
* },
* });
* var foo = new Aws.Inspector.AssessmentTarget("foo", new()
* {
* Name = "assessment target",
* ResourceGroupArn = bar.Arn,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/inspector"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* bar, err := inspector.NewResourceGroup(ctx, "bar", &inspector.ResourceGroupArgs{
* Tags: pulumi.StringMap{
* "Name": pulumi.String("foo"),
* "Env": pulumi.String("bar"),
* },
* })
* if err != nil {
* return err
* }
* _, err = inspector.NewAssessmentTarget(ctx, "foo", &inspector.AssessmentTargetArgs{
* Name: pulumi.String("assessment target"),
* ResourceGroupArn: bar.Arn,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.inspector.ResourceGroup;
* import com.pulumi.aws.inspector.ResourceGroupArgs;
* import com.pulumi.aws.inspector.AssessmentTarget;
* import com.pulumi.aws.inspector.AssessmentTargetArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var bar = new ResourceGroup("bar", ResourceGroupArgs.builder()
* .tags(Map.ofEntries(
* Map.entry("Name", "foo"),
* Map.entry("Env", "bar")
* ))
* .build());
* var foo = new AssessmentTarget("foo", AssessmentTargetArgs.builder()
* .name("assessment target")
* .resourceGroupArn(bar.arn())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* bar:
* type: aws:inspector:ResourceGroup
* properties:
* tags:
* Name: foo
* Env: bar
* foo:
* type: aws:inspector:AssessmentTarget
* properties:
* name: assessment target
* resourceGroupArn: ${bar.arn}
* ```
*
* ## Import
* Using `pulumi import`, import Inspector Classic Assessment Targets using their Amazon Resource Name (ARN). For example:
* ```sh
* $ pulumi import aws:inspector/assessmentTarget:AssessmentTarget example arn:aws:inspector:us-east-1:123456789012:target/0-xxxxxxx
* ```
* @property name The name of the assessment target.
* @property resourceGroupArn Inspector Resource Group Amazon Resource Name (ARN) stating tags for instance matching. If not specified, all EC2 instances in the current AWS account and region are included in the assessment target.
*/
public data class AssessmentTargetArgs(
public val name: Output? = null,
public val resourceGroupArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.inspector.AssessmentTargetArgs =
com.pulumi.aws.inspector.AssessmentTargetArgs.builder()
.name(name?.applyValue({ args0 -> args0 }))
.resourceGroupArn(resourceGroupArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AssessmentTargetArgs].
*/
@PulumiTagMarker
public class AssessmentTargetArgsBuilder internal constructor() {
private var name: Output? = null
private var resourceGroupArn: Output? = null
/**
* @param value The name of the assessment target.
*/
@JvmName("lufibcnkdkgrmhnj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Inspector Resource Group Amazon Resource Name (ARN) stating tags for instance matching. If not specified, all EC2 instances in the current AWS account and region are included in the assessment target.
*/
@JvmName("wvpgyovigxpasvno")
public suspend fun resourceGroupArn(`value`: Output) {
this.resourceGroupArn = value
}
/**
* @param value The name of the assessment target.
*/
@JvmName("botlypfbskvyydfc")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Inspector Resource Group Amazon Resource Name (ARN) stating tags for instance matching. If not specified, all EC2 instances in the current AWS account and region are included in the assessment target.
*/
@JvmName("jekavucmywdwirbx")
public suspend fun resourceGroupArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupArn = mapped
}
internal fun build(): AssessmentTargetArgs = AssessmentTargetArgs(
name = name,
resourceGroupArn = resourceGroupArn,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy