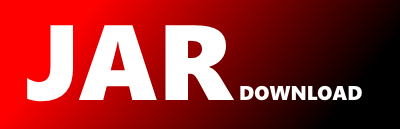
com.pulumi.aws.iot.kotlin.TopicRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iot.kotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchAlarm
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchLog
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchMetric
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleDynamodb
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleDynamodbv2
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleElasticsearch
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorAction
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleFirehose
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleHttp
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleIotAnalytic
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleIotEvent
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleKafka
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleKinesis
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleLambda
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleRepublish
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleS3
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleSns
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleSqs
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleStepFunction
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleTimestream
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchAlarm.Companion.toKotlin as topicRuleCloudwatchAlarmToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchLog.Companion.toKotlin as topicRuleCloudwatchLogToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleCloudwatchMetric.Companion.toKotlin as topicRuleCloudwatchMetricToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleDynamodb.Companion.toKotlin as topicRuleDynamodbToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleDynamodbv2.Companion.toKotlin as topicRuleDynamodbv2ToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleElasticsearch.Companion.toKotlin as topicRuleElasticsearchToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorAction.Companion.toKotlin as topicRuleErrorActionToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleFirehose.Companion.toKotlin as topicRuleFirehoseToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleHttp.Companion.toKotlin as topicRuleHttpToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleIotAnalytic.Companion.toKotlin as topicRuleIotAnalyticToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleIotEvent.Companion.toKotlin as topicRuleIotEventToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleKafka.Companion.toKotlin as topicRuleKafkaToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleKinesis.Companion.toKotlin as topicRuleKinesisToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleLambda.Companion.toKotlin as topicRuleLambdaToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleRepublish.Companion.toKotlin as topicRuleRepublishToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleS3.Companion.toKotlin as topicRuleS3ToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleSns.Companion.toKotlin as topicRuleSnsToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleSqs.Companion.toKotlin as topicRuleSqsToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleStepFunction.Companion.toKotlin as topicRuleStepFunctionToKotlin
import com.pulumi.aws.iot.kotlin.outputs.TopicRuleTimestream.Companion.toKotlin as topicRuleTimestreamToKotlin
/**
* Builder for [TopicRule].
*/
@PulumiTagMarker
public class TopicRuleResourceBuilder internal constructor() {
public var name: String? = null
public var args: TopicRuleArgs = TopicRuleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TopicRuleArgsBuilder.() -> Unit) {
val builder = TopicRuleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): TopicRule {
val builtJavaResource = com.pulumi.aws.iot.TopicRule(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return TopicRule(builtJavaResource)
}
}
/**
* Creates and manages an AWS IoT topic rule.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const mytopic = new aws.sns.Topic("mytopic", {name: "mytopic"});
* const myerrortopic = new aws.sns.Topic("myerrortopic", {name: "myerrortopic"});
* const rule = new aws.iot.TopicRule("rule", {
* name: "MyRule",
* description: "Example rule",
* enabled: true,
* sql: "SELECT * FROM 'topic/test'",
* sqlVersion: "2016-03-23",
* sns: [{
* messageFormat: "RAW",
* roleArn: role.arn,
* targetArn: mytopic.arn,
* }],
* errorAction: {
* sns: {
* messageFormat: "RAW",
* roleArn: role.arn,
* targetArn: myerrortopic.arn,
* },
* },
* });
* const assumeRole = aws.iam.getPolicyDocument({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "Service",
* identifiers: ["iot.amazonaws.com"],
* }],
* actions: ["sts:AssumeRole"],
* }],
* });
* const myrole = new aws.iam.Role("myrole", {
* name: "myrole",
* assumeRolePolicy: assumeRole.then(assumeRole => assumeRole.json),
* });
* const mypolicy = mytopic.arn.apply(arn => aws.iam.getPolicyDocumentOutput({
* statements: [{
* effect: "Allow",
* actions: ["sns:Publish"],
* resources: [arn],
* }],
* }));
* const mypolicyRolePolicy = new aws.iam.RolePolicy("mypolicy", {
* name: "mypolicy",
* role: myrole.id,
* policy: mypolicy.apply(mypolicy => mypolicy.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* mytopic = aws.sns.Topic("mytopic", name="mytopic")
* myerrortopic = aws.sns.Topic("myerrortopic", name="myerrortopic")
* rule = aws.iot.TopicRule("rule",
* name="MyRule",
* description="Example rule",
* enabled=True,
* sql="SELECT * FROM 'topic/test'",
* sql_version="2016-03-23",
* sns=[{
* "message_format": "RAW",
* "role_arn": role["arn"],
* "target_arn": mytopic.arn,
* }],
* error_action={
* "sns": {
* "message_format": "RAW",
* "role_arn": role["arn"],
* "target_arn": myerrortopic.arn,
* },
* })
* assume_role = aws.iam.get_policy_document(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "Service",
* "identifiers": ["iot.amazonaws.com"],
* }],
* "actions": ["sts:AssumeRole"],
* }])
* myrole = aws.iam.Role("myrole",
* name="myrole",
* assume_role_policy=assume_role.json)
* mypolicy = mytopic.arn.apply(lambda arn: aws.iam.get_policy_document_output(statements=[{
* "effect": "Allow",
* "actions": ["sns:Publish"],
* "resources": [arn],
* }]))
* mypolicy_role_policy = aws.iam.RolePolicy("mypolicy",
* name="mypolicy",
* role=myrole.id,
* policy=mypolicy.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var mytopic = new Aws.Sns.Topic("mytopic", new()
* {
* Name = "mytopic",
* });
* var myerrortopic = new Aws.Sns.Topic("myerrortopic", new()
* {
* Name = "myerrortopic",
* });
* var rule = new Aws.Iot.TopicRule("rule", new()
* {
* Name = "MyRule",
* Description = "Example rule",
* Enabled = true,
* Sql = "SELECT * FROM 'topic/test'",
* SqlVersion = "2016-03-23",
* Sns = new[]
* {
* new Aws.Iot.Inputs.TopicRuleSnsArgs
* {
* MessageFormat = "RAW",
* RoleArn = role.Arn,
* TargetArn = mytopic.Arn,
* },
* },
* ErrorAction = new Aws.Iot.Inputs.TopicRuleErrorActionArgs
* {
* Sns = new Aws.Iot.Inputs.TopicRuleErrorActionSnsArgs
* {
* MessageFormat = "RAW",
* RoleArn = role.Arn,
* TargetArn = myerrortopic.Arn,
* },
* },
* });
* var assumeRole = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "Service",
* Identifiers = new[]
* {
* "iot.amazonaws.com",
* },
* },
* },
* Actions = new[]
* {
* "sts:AssumeRole",
* },
* },
* },
* });
* var myrole = new Aws.Iam.Role("myrole", new()
* {
* Name = "myrole",
* AssumeRolePolicy = assumeRole.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* var mypolicy = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Actions = new[]
* {
* "sns:Publish",
* },
* Resources = new[]
* {
* mytopic.Arn,
* },
* },
* },
* });
* var mypolicyRolePolicy = new Aws.Iam.RolePolicy("mypolicy", new()
* {
* Name = "mypolicy",
* Role = myrole.Id,
* Policy = mypolicy.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iot"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/sns"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* mytopic, err := sns.NewTopic(ctx, "mytopic", &sns.TopicArgs{
* Name: pulumi.String("mytopic"),
* })
* if err != nil {
* return err
* }
* myerrortopic, err := sns.NewTopic(ctx, "myerrortopic", &sns.TopicArgs{
* Name: pulumi.String("myerrortopic"),
* })
* if err != nil {
* return err
* }
* _, err = iot.NewTopicRule(ctx, "rule", &iot.TopicRuleArgs{
* Name: pulumi.String("MyRule"),
* Description: pulumi.String("Example rule"),
* Enabled: pulumi.Bool(true),
* Sql: pulumi.String("SELECT * FROM 'topic/test'"),
* SqlVersion: pulumi.String("2016-03-23"),
* Sns: iot.TopicRuleSnsArray{
* &iot.TopicRuleSnsArgs{
* MessageFormat: pulumi.String("RAW"),
* RoleArn: pulumi.Any(role.Arn),
* TargetArn: mytopic.Arn,
* },
* },
* ErrorAction: &iot.TopicRuleErrorActionArgs{
* Sns: &iot.TopicRuleErrorActionSnsArgs{
* MessageFormat: pulumi.String("RAW"),
* RoleArn: pulumi.Any(role.Arn),
* TargetArn: myerrortopic.Arn,
* },
* },
* })
* if err != nil {
* return err
* }
* assumeRole, err := iam.GetPolicyDocument(ctx, &iam.GetPolicyDocumentArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: pulumi.StringRef("Allow"),
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "Service",
* Identifiers: []string{
* "iot.amazonaws.com",
* },
* },
* },
* Actions: []string{
* "sts:AssumeRole",
* },
* },
* },
* }, nil);
* if err != nil {
* return err
* }
* myrole, err := iam.NewRole(ctx, "myrole", &iam.RoleArgs{
* Name: pulumi.String("myrole"),
* AssumeRolePolicy: pulumi.String(assumeRole.Json),
* })
* if err != nil {
* return err
* }
* mypolicy := mytopic.Arn.ApplyT(func(arn string) (iam.GetPolicyDocumentResult, error) {
* return iam.GetPolicyDocumentResult(interface{}(iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: "Allow",
* Actions: []string{
* "sns:Publish",
* },
* Resources: interface{}{
* arn,
* },
* },
* },
* }, nil))), nil
* }).(iam.GetPolicyDocumentResultOutput)
* _, err = iam.NewRolePolicy(ctx, "mypolicy", &iam.RolePolicyArgs{
* Name: pulumi.String("mypolicy"),
* Role: myrole.ID(),
* Policy: pulumi.String(mypolicy.ApplyT(func(mypolicy iam.GetPolicyDocumentResult) (*string, error) {
* return &mypolicy.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.sns.Topic;
* import com.pulumi.aws.sns.TopicArgs;
* import com.pulumi.aws.iot.TopicRule;
* import com.pulumi.aws.iot.TopicRuleArgs;
* import com.pulumi.aws.iot.inputs.TopicRuleSnsArgs;
* import com.pulumi.aws.iot.inputs.TopicRuleErrorActionArgs;
* import com.pulumi.aws.iot.inputs.TopicRuleErrorActionSnsArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.iam.RolePolicy;
* import com.pulumi.aws.iam.RolePolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var mytopic = new Topic("mytopic", TopicArgs.builder()
* .name("mytopic")
* .build());
* var myerrortopic = new Topic("myerrortopic", TopicArgs.builder()
* .name("myerrortopic")
* .build());
* var rule = new TopicRule("rule", TopicRuleArgs.builder()
* .name("MyRule")
* .description("Example rule")
* .enabled(true)
* .sql("SELECT * FROM 'topic/test'")
* .sqlVersion("2016-03-23")
* .sns(TopicRuleSnsArgs.builder()
* .messageFormat("RAW")
* .roleArn(role.arn())
* .targetArn(mytopic.arn())
* .build())
* .errorAction(TopicRuleErrorActionArgs.builder()
* .sns(TopicRuleErrorActionSnsArgs.builder()
* .messageFormat("RAW")
* .roleArn(role.arn())
* .targetArn(myerrortopic.arn())
* .build())
* .build())
* .build());
* final var assumeRole = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("Service")
* .identifiers("iot.amazonaws.com")
* .build())
* .actions("sts:AssumeRole")
* .build())
* .build());
* var myrole = new Role("myrole", RoleArgs.builder()
* .name("myrole")
* .assumeRolePolicy(assumeRole.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json()))
* .build());
* final var mypolicy = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .actions("sns:Publish")
* .resources(mytopic.arn())
* .build())
* .build());
* var mypolicyRolePolicy = new RolePolicy("mypolicyRolePolicy", RolePolicyArgs.builder()
* .name("mypolicy")
* .role(myrole.id())
* .policy(mypolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult).applyValue(mypolicy -> mypolicy.applyValue(getPolicyDocumentResult -> getPolicyDocumentResult.json())))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* rule:
* type: aws:iot:TopicRule
* properties:
* name: MyRule
* description: Example rule
* enabled: true
* sql: SELECT * FROM 'topic/test'
* sqlVersion: 2016-03-23
* sns:
* - messageFormat: RAW
* roleArn: ${role.arn}
* targetArn: ${mytopic.arn}
* errorAction:
* sns:
* messageFormat: RAW
* roleArn: ${role.arn}
* targetArn: ${myerrortopic.arn}
* mytopic:
* type: aws:sns:Topic
* properties:
* name: mytopic
* myerrortopic:
* type: aws:sns:Topic
* properties:
* name: myerrortopic
* myrole:
* type: aws:iam:Role
* properties:
* name: myrole
* assumeRolePolicy: ${assumeRole.json}
* mypolicyRolePolicy:
* type: aws:iam:RolePolicy
* name: mypolicy
* properties:
* name: mypolicy
* role: ${myrole.id}
* policy: ${mypolicy.json}
* variables:
* assumeRole:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: Service
* identifiers:
* - iot.amazonaws.com
* actions:
* - sts:AssumeRole
* mypolicy:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* actions:
* - sns:Publish
* resources:
* - ${mytopic.arn}
* ```
*
* ## Import
* Using `pulumi import`, import IoT Topic Rules using the `name`. For example:
* ```sh
* $ pulumi import aws:iot/topicRule:TopicRule rule
* ```
*/
public class TopicRule internal constructor(
override val javaResource: com.pulumi.aws.iot.TopicRule,
) : KotlinCustomResource(javaResource, TopicRuleMapper) {
/**
* The ARN of the topic rule
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
public val cloudwatchAlarms: Output>?
get() = javaResource.cloudwatchAlarms().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleCloudwatchAlarmToKotlin(args0)
})
})
}).orElse(null)
})
public val cloudwatchLogs: Output>?
get() = javaResource.cloudwatchLogs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleCloudwatchLogToKotlin(args0)
})
})
}).orElse(null)
})
public val cloudwatchMetrics: Output>?
get() = javaResource.cloudwatchMetrics().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleCloudwatchMetricToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The description of the rule.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
public val dynamodbs: Output>?
get() = javaResource.dynamodbs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleDynamodbToKotlin(args0) })
})
}).orElse(null)
})
public val dynamodbv2s: Output>?
get() = javaResource.dynamodbv2s().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleDynamodbv2ToKotlin(args0) })
})
}).orElse(null)
})
public val elasticsearch: Output>?
get() = javaResource.elasticsearch().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleElasticsearchToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies whether the rule is enabled.
*/
public val enabled: Output
get() = javaResource.enabled().applyValue({ args0 -> args0 })
/**
* Configuration block with error action to be associated with the rule. See the documentation for `cloudwatch_alarm`, `cloudwatch_logs`, `cloudwatch_metric`, `dynamodb`, `dynamodbv2`, `elasticsearch`, `firehose`, `http`, `iot_analytics`, `iot_events`, `kafka`, `kinesis`, `lambda`, `republish`, `s3`, `sns`, `sqs`, `step_functions`, `timestream` configuration blocks for further configuration details.
*/
public val errorAction: Output?
get() = javaResource.errorAction().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleErrorActionToKotlin(args0)
})
}).orElse(null)
})
public val firehoses: Output>?
get() = javaResource.firehoses().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleFirehoseToKotlin(args0) })
})
}).orElse(null)
})
public val https: Output>?
get() = javaResource.https().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleHttpToKotlin(args0) })
})
}).orElse(null)
})
public val iotAnalytics: Output>?
get() = javaResource.iotAnalytics().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleIotAnalyticToKotlin(args0) })
})
}).orElse(null)
})
public val iotEvents: Output>?
get() = javaResource.iotEvents().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleIotEventToKotlin(args0) })
})
}).orElse(null)
})
public val kafkas: Output>?
get() = javaResource.kafkas().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleKafkaToKotlin(args0) })
})
}).orElse(null)
})
public val kineses: Output>?
get() = javaResource.kineses().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleKinesisToKotlin(args0) })
})
}).orElse(null)
})
public val lambdas: Output>?
get() = javaResource.lambdas().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleLambdaToKotlin(args0) })
})
}).orElse(null)
})
/**
* The name of the rule.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val republishes: Output>?
get() = javaResource.republishes().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleRepublishToKotlin(args0) })
})
}).orElse(null)
})
public val s3: Output>?
get() = javaResource.s3().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleS3ToKotlin(args0) })
})
}).orElse(null)
})
public val sns: Output>?
get() = javaResource.sns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleSnsToKotlin(args0) })
})
}).orElse(null)
})
/**
* The SQL statement used to query the topic. For more information, see AWS IoT SQL Reference (http://docs.aws.amazon.com/iot/latest/developerguide/iot-rules.html#aws-iot-sql-reference) in the AWS IoT Developer Guide.
*/
public val sql: Output
get() = javaResource.sql().applyValue({ args0 -> args0 })
/**
* The version of the SQL rules engine to use when evaluating the rule.
*/
public val sqlVersion: Output
get() = javaResource.sqlVersion().applyValue({ args0 -> args0 })
public val sqs: Output>?
get() = javaResource.sqs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> topicRuleSqsToKotlin(args0) })
})
}).orElse(null)
})
public val stepFunctions: Output>?
get() = javaResource.stepFunctions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
topicRuleStepFunctionToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy