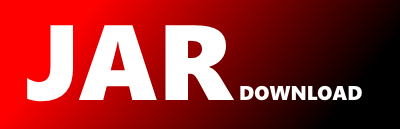
com.pulumi.aws.iot.kotlin.inputs.IndexingConfigurationThingIndexingConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iot.kotlin.inputs
import com.pulumi.aws.iot.inputs.IndexingConfigurationThingIndexingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property customFields Contains custom field names and their data type. See below.
* @property deviceDefenderIndexingMode Device Defender indexing mode. Valid values: `VIOLATIONS`, `OFF`. Default: `OFF`.
* @property filter Required if `named_shadow_indexing_mode` is `ON`. Enables to add named shadows filtered by `filter` to fleet indexing configuration.
* @property managedFields Contains fields that are indexed and whose types are already known by the Fleet Indexing service. See below.
* @property namedShadowIndexingMode [Named shadow](https://docs.aws.amazon.com/iot/latest/developerguide/iot-device-shadows.html) indexing mode. Valid values: `ON`, `OFF`. Default: `OFF`.
* @property thingConnectivityIndexingMode Thing connectivity indexing mode. Valid values: `STATUS`, `OFF`. Default: `OFF`.
* @property thingIndexingMode Thing indexing mode. Valid values: `REGISTRY`, `REGISTRY_AND_SHADOW`, `OFF`.
*/
public data class IndexingConfigurationThingIndexingConfigurationArgs(
public val customFields: Output>? = null,
public val deviceDefenderIndexingMode: Output? = null,
public val filter: Output? = null,
public val managedFields: Output>? = null,
public val namedShadowIndexingMode: Output? = null,
public val thingConnectivityIndexingMode: Output? = null,
public val thingIndexingMode: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.iot.inputs.IndexingConfigurationThingIndexingConfigurationArgs =
com.pulumi.aws.iot.inputs.IndexingConfigurationThingIndexingConfigurationArgs.builder()
.customFields(
customFields?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.deviceDefenderIndexingMode(deviceDefenderIndexingMode?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.managedFields(
managedFields?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.namedShadowIndexingMode(namedShadowIndexingMode?.applyValue({ args0 -> args0 }))
.thingConnectivityIndexingMode(thingConnectivityIndexingMode?.applyValue({ args0 -> args0 }))
.thingIndexingMode(thingIndexingMode.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [IndexingConfigurationThingIndexingConfigurationArgs].
*/
@PulumiTagMarker
public class IndexingConfigurationThingIndexingConfigurationArgsBuilder internal constructor() {
private var customFields:
Output>? = null
private var deviceDefenderIndexingMode: Output? = null
private var filter: Output? = null
private var managedFields:
Output>? = null
private var namedShadowIndexingMode: Output? = null
private var thingConnectivityIndexingMode: Output? = null
private var thingIndexingMode: Output? = null
/**
* @param value Contains custom field names and their data type. See below.
*/
@JvmName("dhdaxnphtensxeae")
public suspend fun customFields(`value`: Output>) {
this.customFields = value
}
@JvmName("evlegigvjxkugqbe")
public suspend fun customFields(vararg values: Output) {
this.customFields = Output.all(values.asList())
}
/**
* @param values Contains custom field names and their data type. See below.
*/
@JvmName("sqtookjdhubwtxkj")
public suspend fun customFields(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy