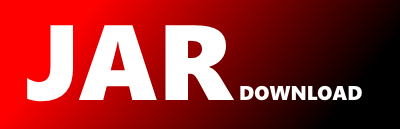
com.pulumi.aws.iot.kotlin.inputs.TopicRuleDynamodbArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iot.kotlin.inputs
import com.pulumi.aws.iot.inputs.TopicRuleDynamodbArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property hashKeyField The hash key name.
* @property hashKeyType The hash key type. Valid values are "STRING" or "NUMBER".
* @property hashKeyValue The hash key value.
* @property operation The operation. Valid values are "INSERT", "UPDATE", or "DELETE".
* @property payloadField The action payload.
* @property rangeKeyField The range key name.
* @property rangeKeyType The range key type. Valid values are "STRING" or "NUMBER".
* @property rangeKeyValue The range key value.
* @property roleArn The ARN of the IAM role that grants access to the DynamoDB table.
* @property tableName The name of the DynamoDB table.
*/
public data class TopicRuleDynamodbArgs(
public val hashKeyField: Output,
public val hashKeyType: Output? = null,
public val hashKeyValue: Output,
public val operation: Output? = null,
public val payloadField: Output? = null,
public val rangeKeyField: Output? = null,
public val rangeKeyType: Output? = null,
public val rangeKeyValue: Output? = null,
public val roleArn: Output,
public val tableName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.iot.inputs.TopicRuleDynamodbArgs =
com.pulumi.aws.iot.inputs.TopicRuleDynamodbArgs.builder()
.hashKeyField(hashKeyField.applyValue({ args0 -> args0 }))
.hashKeyType(hashKeyType?.applyValue({ args0 -> args0 }))
.hashKeyValue(hashKeyValue.applyValue({ args0 -> args0 }))
.operation(operation?.applyValue({ args0 -> args0 }))
.payloadField(payloadField?.applyValue({ args0 -> args0 }))
.rangeKeyField(rangeKeyField?.applyValue({ args0 -> args0 }))
.rangeKeyType(rangeKeyType?.applyValue({ args0 -> args0 }))
.rangeKeyValue(rangeKeyValue?.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.tableName(tableName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TopicRuleDynamodbArgs].
*/
@PulumiTagMarker
public class TopicRuleDynamodbArgsBuilder internal constructor() {
private var hashKeyField: Output? = null
private var hashKeyType: Output? = null
private var hashKeyValue: Output? = null
private var operation: Output? = null
private var payloadField: Output? = null
private var rangeKeyField: Output? = null
private var rangeKeyType: Output? = null
private var rangeKeyValue: Output? = null
private var roleArn: Output? = null
private var tableName: Output? = null
/**
* @param value The hash key name.
*/
@JvmName("jmjxhaodecwjjoeo")
public suspend fun hashKeyField(`value`: Output) {
this.hashKeyField = value
}
/**
* @param value The hash key type. Valid values are "STRING" or "NUMBER".
*/
@JvmName("ytnovmsoxekasmot")
public suspend fun hashKeyType(`value`: Output) {
this.hashKeyType = value
}
/**
* @param value The hash key value.
*/
@JvmName("bopyaimjegvsxsxc")
public suspend fun hashKeyValue(`value`: Output) {
this.hashKeyValue = value
}
/**
* @param value The operation. Valid values are "INSERT", "UPDATE", or "DELETE".
*/
@JvmName("ycwbhgaynyyxhxuw")
public suspend fun operation(`value`: Output) {
this.operation = value
}
/**
* @param value The action payload.
*/
@JvmName("hhydgrpebumpkrnk")
public suspend fun payloadField(`value`: Output) {
this.payloadField = value
}
/**
* @param value The range key name.
*/
@JvmName("jfufkctkgnhpadrt")
public suspend fun rangeKeyField(`value`: Output) {
this.rangeKeyField = value
}
/**
* @param value The range key type. Valid values are "STRING" or "NUMBER".
*/
@JvmName("aeognyimvophieyk")
public suspend fun rangeKeyType(`value`: Output) {
this.rangeKeyType = value
}
/**
* @param value The range key value.
*/
@JvmName("nrgaerkdcooexqyr")
public suspend fun rangeKeyValue(`value`: Output) {
this.rangeKeyValue = value
}
/**
* @param value The ARN of the IAM role that grants access to the DynamoDB table.
*/
@JvmName("xyxcfxpjuuqulkfy")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The name of the DynamoDB table.
*/
@JvmName("stlbxnssshphwoyg")
public suspend fun tableName(`value`: Output) {
this.tableName = value
}
/**
* @param value The hash key name.
*/
@JvmName("kxkakwtrpgrgcxkq")
public suspend fun hashKeyField(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hashKeyField = mapped
}
/**
* @param value The hash key type. Valid values are "STRING" or "NUMBER".
*/
@JvmName("tlgwbwrohcpscuhl")
public suspend fun hashKeyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hashKeyType = mapped
}
/**
* @param value The hash key value.
*/
@JvmName("rpqcswkoxoegnxus")
public suspend fun hashKeyValue(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.hashKeyValue = mapped
}
/**
* @param value The operation. Valid values are "INSERT", "UPDATE", or "DELETE".
*/
@JvmName("judaxaavkfsyytra")
public suspend fun operation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operation = mapped
}
/**
* @param value The action payload.
*/
@JvmName("ofowaeoavagcdvck")
public suspend fun payloadField(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.payloadField = mapped
}
/**
* @param value The range key name.
*/
@JvmName("hsskvcmaychxhxnb")
public suspend fun rangeKeyField(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyField = mapped
}
/**
* @param value The range key type. Valid values are "STRING" or "NUMBER".
*/
@JvmName("hyidmawettvytgdr")
public suspend fun rangeKeyType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyType = mapped
}
/**
* @param value The range key value.
*/
@JvmName("pbnkdnokbrwgtgfl")
public suspend fun rangeKeyValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rangeKeyValue = mapped
}
/**
* @param value The ARN of the IAM role that grants access to the DynamoDB table.
*/
@JvmName("eeegcmvvumsrimrp")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The name of the DynamoDB table.
*/
@JvmName("jsnayjhgouykjxjv")
public suspend fun tableName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.tableName = mapped
}
internal fun build(): TopicRuleDynamodbArgs = TopicRuleDynamodbArgs(
hashKeyField = hashKeyField ?: throw PulumiNullFieldException("hashKeyField"),
hashKeyType = hashKeyType,
hashKeyValue = hashKeyValue ?: throw PulumiNullFieldException("hashKeyValue"),
operation = operation,
payloadField = payloadField,
rangeKeyField = rangeKeyField,
rangeKeyType = rangeKeyType,
rangeKeyValue = rangeKeyValue,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
tableName = tableName ?: throw PulumiNullFieldException("tableName"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy