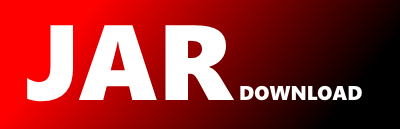
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorAction.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.iot.kotlin.outputs
import kotlin.Suppress
/**
*
* @property cloudwatchAlarm
* @property cloudwatchLogs
* @property cloudwatchMetric
* @property dynamodb
* @property dynamodbv2
* @property elasticsearch
* @property firehose
* @property http
* @property iotAnalytics
* @property iotEvents
* @property kafka
* @property kinesis
* @property lambda
* @property republish
* @property s3
* @property sns
* @property sqs
* @property stepFunctions
* @property timestream
*/
public data class TopicRuleErrorAction(
public val cloudwatchAlarm: TopicRuleErrorActionCloudwatchAlarm? = null,
public val cloudwatchLogs: TopicRuleErrorActionCloudwatchLogs? = null,
public val cloudwatchMetric: TopicRuleErrorActionCloudwatchMetric? = null,
public val dynamodb: TopicRuleErrorActionDynamodb? = null,
public val dynamodbv2: TopicRuleErrorActionDynamodbv2? = null,
public val elasticsearch: TopicRuleErrorActionElasticsearch? = null,
public val firehose: TopicRuleErrorActionFirehose? = null,
public val http: TopicRuleErrorActionHttp? = null,
public val iotAnalytics: TopicRuleErrorActionIotAnalytics? = null,
public val iotEvents: TopicRuleErrorActionIotEvents? = null,
public val kafka: TopicRuleErrorActionKafka? = null,
public val kinesis: TopicRuleErrorActionKinesis? = null,
public val lambda: TopicRuleErrorActionLambda? = null,
public val republish: TopicRuleErrorActionRepublish? = null,
public val s3: TopicRuleErrorActionS3? = null,
public val sns: TopicRuleErrorActionSns? = null,
public val sqs: TopicRuleErrorActionSqs? = null,
public val stepFunctions: TopicRuleErrorActionStepFunctions? = null,
public val timestream: TopicRuleErrorActionTimestream? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.iot.outputs.TopicRuleErrorAction): TopicRuleErrorAction = TopicRuleErrorAction(
cloudwatchAlarm = javaType.cloudwatchAlarm().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionCloudwatchAlarm.Companion.toKotlin(args0)
})
}).orElse(null),
cloudwatchLogs = javaType.cloudwatchLogs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionCloudwatchLogs.Companion.toKotlin(args0)
})
}).orElse(null),
cloudwatchMetric = javaType.cloudwatchMetric().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionCloudwatchMetric.Companion.toKotlin(args0)
})
}).orElse(null),
dynamodb = javaType.dynamodb().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionDynamodb.Companion.toKotlin(args0)
})
}).orElse(null),
dynamodbv2 = javaType.dynamodbv2().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionDynamodbv2.Companion.toKotlin(args0)
})
}).orElse(null),
elasticsearch = javaType.elasticsearch().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionElasticsearch.Companion.toKotlin(args0)
})
}).orElse(null),
firehose = javaType.firehose().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionFirehose.Companion.toKotlin(args0)
})
}).orElse(null),
http = javaType.http().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionHttp.Companion.toKotlin(args0)
})
}).orElse(null),
iotAnalytics = javaType.iotAnalytics().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionIotAnalytics.Companion.toKotlin(args0)
})
}).orElse(null),
iotEvents = javaType.iotEvents().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionIotEvents.Companion.toKotlin(args0)
})
}).orElse(null),
kafka = javaType.kafka().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionKafka.Companion.toKotlin(args0)
})
}).orElse(null),
kinesis = javaType.kinesis().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionKinesis.Companion.toKotlin(args0)
})
}).orElse(null),
lambda = javaType.lambda().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionLambda.Companion.toKotlin(args0)
})
}).orElse(null),
republish = javaType.republish().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionRepublish.Companion.toKotlin(args0)
})
}).orElse(null),
s3 = javaType.s3().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionS3.Companion.toKotlin(args0)
})
}).orElse(null),
sns = javaType.sns().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionSns.Companion.toKotlin(args0)
})
}).orElse(null),
sqs = javaType.sqs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionSqs.Companion.toKotlin(args0)
})
}).orElse(null),
stepFunctions = javaType.stepFunctions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionStepFunctions.Companion.toKotlin(args0)
})
}).orElse(null),
timestream = javaType.timestream().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.iot.kotlin.outputs.TopicRuleErrorActionTimestream.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy