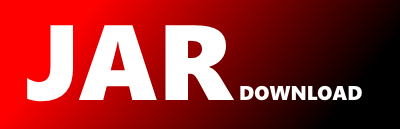
com.pulumi.aws.kendra.kotlin.inputs.DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kendra.kotlin.inputs
import com.pulumi.aws.kendra.inputs.DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property targetDocumentAttributeKey The identifier of the target document attribute or metadata field. For example, 'Department' could be an identifier for the target attribute or metadata field that includes the department names associated with the documents.
* @property targetDocumentAttributeValue The target value you want to create for the target attribute. For example, 'Finance' could be the target value for the target attribute key 'Department'. See target_document_attribute_value.
* @property targetDocumentAttributeValueDeletion `TRUE` to delete the existing target value for your specified target attribute key. You cannot create a target value and set this to `TRUE`. To create a target value (`TargetDocumentAttributeValue`), set this to `FALSE`.
*/
public data class DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs(
public val targetDocumentAttributeKey: Output? = null,
public val targetDocumentAttributeValue: Output? =
null,
public val targetDocumentAttributeValueDeletion: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kendra.inputs.DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs =
com.pulumi.aws.kendra.inputs.DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs.builder()
.targetDocumentAttributeKey(targetDocumentAttributeKey?.applyValue({ args0 -> args0 }))
.targetDocumentAttributeValue(
targetDocumentAttributeValue?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.targetDocumentAttributeValueDeletion(
targetDocumentAttributeValueDeletion?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs].
*/
@PulumiTagMarker
public class DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgsBuilder
internal constructor() {
private var targetDocumentAttributeKey: Output? = null
private var targetDocumentAttributeValue:
Output? =
null
private var targetDocumentAttributeValueDeletion: Output? = null
/**
* @param value The identifier of the target document attribute or metadata field. For example, 'Department' could be an identifier for the target attribute or metadata field that includes the department names associated with the documents.
*/
@JvmName("yonlcrskpmqvukio")
public suspend fun targetDocumentAttributeKey(`value`: Output) {
this.targetDocumentAttributeKey = value
}
/**
* @param value The target value you want to create for the target attribute. For example, 'Finance' could be the target value for the target attribute key 'Department'. See target_document_attribute_value.
*/
@JvmName("ctixrsrojkwntphl")
public suspend fun targetDocumentAttributeValue(`value`: Output) {
this.targetDocumentAttributeValue = value
}
/**
* @param value `TRUE` to delete the existing target value for your specified target attribute key. You cannot create a target value and set this to `TRUE`. To create a target value (`TargetDocumentAttributeValue`), set this to `FALSE`.
*/
@JvmName("giqqernphvaocmyq")
public suspend fun targetDocumentAttributeValueDeletion(`value`: Output) {
this.targetDocumentAttributeValueDeletion = value
}
/**
* @param value The identifier of the target document attribute or metadata field. For example, 'Department' could be an identifier for the target attribute or metadata field that includes the department names associated with the documents.
*/
@JvmName("ydpigmmwopfwlmoq")
public suspend fun targetDocumentAttributeKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetDocumentAttributeKey = mapped
}
/**
* @param value The target value you want to create for the target attribute. For example, 'Finance' could be the target value for the target attribute key 'Department'. See target_document_attribute_value.
*/
@JvmName("mvgkwamqrdrlaxof")
public suspend fun targetDocumentAttributeValue(`value`: DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetTargetDocumentAttributeValueArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetDocumentAttributeValue = mapped
}
/**
* @param argument The target value you want to create for the target attribute. For example, 'Finance' could be the target value for the target attribute key 'Department'. See target_document_attribute_value.
*/
@JvmName("bxpimbcbpkpcmmlt")
public suspend fun targetDocumentAttributeValue(argument: suspend DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetTargetDocumentAttributeValueArgsBuilder.() -> Unit) {
val toBeMapped =
DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetTargetDocumentAttributeValueArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetDocumentAttributeValue = mapped
}
/**
* @param value `TRUE` to delete the existing target value for your specified target attribute key. You cannot create a target value and set this to `TRUE`. To create a target value (`TargetDocumentAttributeValue`), set this to `FALSE`.
*/
@JvmName("wqwnutnxnbjunmxb")
public suspend fun targetDocumentAttributeValueDeletion(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetDocumentAttributeValueDeletion = mapped
}
internal fun build(): DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs = DataSourceCustomDocumentEnrichmentConfigurationInlineConfigurationTargetArgs(
targetDocumentAttributeKey = targetDocumentAttributeKey,
targetDocumentAttributeValue = targetDocumentAttributeValue,
targetDocumentAttributeValueDeletion = targetDocumentAttributeValueDeletion,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy