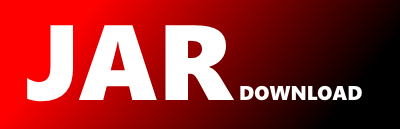
com.pulumi.aws.kendra.kotlin.inputs.IndexDocumentMetadataConfigurationUpdateArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kendra.kotlin.inputs
import com.pulumi.aws.kendra.inputs.IndexDocumentMetadataConfigurationUpdateArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property name The name of the index field. Minimum length of 1. Maximum length of 30.
* @property relevance A block that provides manual tuning parameters to determine how the field affects the search results. Detailed below
* @property search A block that provides information about how the field is used during a search. Documented below. Detailed below
* @property type The data type of the index field. Valid values are `STRING_VALUE`, `STRING_LIST_VALUE`, `LONG_VALUE`, `DATE_VALUE`.
*/
public data class IndexDocumentMetadataConfigurationUpdateArgs(
public val name: Output,
public val relevance: Output? = null,
public val search: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kendra.inputs.IndexDocumentMetadataConfigurationUpdateArgs =
com.pulumi.aws.kendra.inputs.IndexDocumentMetadataConfigurationUpdateArgs.builder()
.name(name.applyValue({ args0 -> args0 }))
.relevance(relevance?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.search(search?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [IndexDocumentMetadataConfigurationUpdateArgs].
*/
@PulumiTagMarker
public class IndexDocumentMetadataConfigurationUpdateArgsBuilder internal constructor() {
private var name: Output? = null
private var relevance: Output? = null
private var search: Output? = null
private var type: Output? = null
/**
* @param value The name of the index field. Minimum length of 1. Maximum length of 30.
*/
@JvmName("glnsmbahcgurjgit")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A block that provides manual tuning parameters to determine how the field affects the search results. Detailed below
*/
@JvmName("sgicbftfbmlfpotj")
public suspend fun relevance(`value`: Output) {
this.relevance = value
}
/**
* @param value A block that provides information about how the field is used during a search. Documented below. Detailed below
*/
@JvmName("nxqterelbqlijgrn")
public suspend fun search(`value`: Output) {
this.search = value
}
/**
* @param value The data type of the index field. Valid values are `STRING_VALUE`, `STRING_LIST_VALUE`, `LONG_VALUE`, `DATE_VALUE`.
*/
@JvmName("edmahyegpjmlrifb")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The name of the index field. Minimum length of 1. Maximum length of 30.
*/
@JvmName("etxnyfjidwbmkhkd")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value A block that provides manual tuning parameters to determine how the field affects the search results. Detailed below
*/
@JvmName("pwblckhauiiameor")
public suspend fun relevance(`value`: IndexDocumentMetadataConfigurationUpdateRelevanceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.relevance = mapped
}
/**
* @param argument A block that provides manual tuning parameters to determine how the field affects the search results. Detailed below
*/
@JvmName("mktggarugcmqkute")
public suspend fun relevance(argument: suspend IndexDocumentMetadataConfigurationUpdateRelevanceArgsBuilder.() -> Unit) {
val toBeMapped = IndexDocumentMetadataConfigurationUpdateRelevanceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.relevance = mapped
}
/**
* @param value A block that provides information about how the field is used during a search. Documented below. Detailed below
*/
@JvmName("fqrsqaevdtxbfake")
public suspend fun search(`value`: IndexDocumentMetadataConfigurationUpdateSearchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.search = mapped
}
/**
* @param argument A block that provides information about how the field is used during a search. Documented below. Detailed below
*/
@JvmName("spavolrhiclymtrl")
public suspend fun search(argument: suspend IndexDocumentMetadataConfigurationUpdateSearchArgsBuilder.() -> Unit) {
val toBeMapped = IndexDocumentMetadataConfigurationUpdateSearchArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.search = mapped
}
/**
* @param value The data type of the index field. Valid values are `STRING_VALUE`, `STRING_LIST_VALUE`, `LONG_VALUE`, `DATE_VALUE`.
*/
@JvmName("ouoehjpibhbcmwsr")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): IndexDocumentMetadataConfigurationUpdateArgs =
IndexDocumentMetadataConfigurationUpdateArgs(
name = name ?: throw PulumiNullFieldException("name"),
relevance = relevance,
search = search,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy