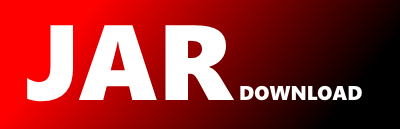
com.pulumi.aws.kinesis.kotlin.inputs.AnalyticsApplicationOutputArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.inputs
import com.pulumi.aws.kinesis.inputs.AnalyticsApplicationOutputArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property id The ARN of the Kinesis Analytics Application.
* @property kinesisFirehose The Kinesis Firehose configuration for the destination stream. Conflicts with `kinesis_stream`.
* See Kinesis Firehose below for more details.
* @property kinesisStream The Kinesis Stream configuration for the destination stream. Conflicts with `kinesis_firehose`.
* See Kinesis Stream below for more details.
* @property lambda The Lambda function destination. See Lambda below for more details.
* @property name The Name of the in-application stream.
* @property schema The Schema format of the data written to the destination. See Destination Schema below for more details.
*/
public data class AnalyticsApplicationOutputArgs(
public val id: Output? = null,
public val kinesisFirehose: Output? = null,
public val kinesisStream: Output? = null,
public val lambda: Output? = null,
public val name: Output,
public val schema: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesis.inputs.AnalyticsApplicationOutputArgs =
com.pulumi.aws.kinesis.inputs.AnalyticsApplicationOutputArgs.builder()
.id(id?.applyValue({ args0 -> args0 }))
.kinesisFirehose(kinesisFirehose?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kinesisStream(kinesisStream?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.lambda(lambda?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.schema(schema.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalyticsApplicationOutputArgs].
*/
@PulumiTagMarker
public class AnalyticsApplicationOutputArgsBuilder internal constructor() {
private var id: Output? = null
private var kinesisFirehose: Output? = null
private var kinesisStream: Output? = null
private var lambda: Output? = null
private var name: Output? = null
private var schema: Output? = null
/**
* @param value The ARN of the Kinesis Analytics Application.
*/
@JvmName("bjxldohfgvjmtqum")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value The Kinesis Firehose configuration for the destination stream. Conflicts with `kinesis_stream`.
* See Kinesis Firehose below for more details.
*/
@JvmName("tysijijxoakwdqgf")
public suspend fun kinesisFirehose(`value`: Output) {
this.kinesisFirehose = value
}
/**
* @param value The Kinesis Stream configuration for the destination stream. Conflicts with `kinesis_firehose`.
* See Kinesis Stream below for more details.
*/
@JvmName("ocfiuxnsfwxajteh")
public suspend fun kinesisStream(`value`: Output) {
this.kinesisStream = value
}
/**
* @param value The Lambda function destination. See Lambda below for more details.
*/
@JvmName("ubgotlbssjpbhgtx")
public suspend fun lambda(`value`: Output) {
this.lambda = value
}
/**
* @param value The Name of the in-application stream.
*/
@JvmName("stsbrheweuxlkefo")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The Schema format of the data written to the destination. See Destination Schema below for more details.
*/
@JvmName("getrxupsybxgwbyl")
public suspend fun schema(`value`: Output) {
this.schema = value
}
/**
* @param value The ARN of the Kinesis Analytics Application.
*/
@JvmName("pevuvhouudiljpwt")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value The Kinesis Firehose configuration for the destination stream. Conflicts with `kinesis_stream`.
* See Kinesis Firehose below for more details.
*/
@JvmName("kkqusqxxkerrxslt")
public suspend fun kinesisFirehose(`value`: AnalyticsApplicationOutputKinesisFirehoseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisFirehose = mapped
}
/**
* @param argument The Kinesis Firehose configuration for the destination stream. Conflicts with `kinesis_stream`.
* See Kinesis Firehose below for more details.
*/
@JvmName("aucskxhysniwbxfe")
public suspend fun kinesisFirehose(argument: suspend AnalyticsApplicationOutputKinesisFirehoseArgsBuilder.() -> Unit) {
val toBeMapped = AnalyticsApplicationOutputKinesisFirehoseArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisFirehose = mapped
}
/**
* @param value The Kinesis Stream configuration for the destination stream. Conflicts with `kinesis_firehose`.
* See Kinesis Stream below for more details.
*/
@JvmName("svxtiqkifxmpdxbb")
public suspend fun kinesisStream(`value`: AnalyticsApplicationOutputKinesisStreamArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisStream = mapped
}
/**
* @param argument The Kinesis Stream configuration for the destination stream. Conflicts with `kinesis_firehose`.
* See Kinesis Stream below for more details.
*/
@JvmName("vdbdmjhqmitqtmsm")
public suspend fun kinesisStream(argument: suspend AnalyticsApplicationOutputKinesisStreamArgsBuilder.() -> Unit) {
val toBeMapped = AnalyticsApplicationOutputKinesisStreamArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisStream = mapped
}
/**
* @param value The Lambda function destination. See Lambda below for more details.
*/
@JvmName("pjwqukrmkgqucuay")
public suspend fun lambda(`value`: AnalyticsApplicationOutputLambdaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lambda = mapped
}
/**
* @param argument The Lambda function destination. See Lambda below for more details.
*/
@JvmName("nkgbbhwuwcdknnog")
public suspend fun lambda(argument: suspend AnalyticsApplicationOutputLambdaArgsBuilder.() -> Unit) {
val toBeMapped = AnalyticsApplicationOutputLambdaArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.lambda = mapped
}
/**
* @param value The Name of the in-application stream.
*/
@JvmName("rlsetgnydqomcegx")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The Schema format of the data written to the destination. See Destination Schema below for more details.
*/
@JvmName("fhrrcixyhicyigau")
public suspend fun schema(`value`: AnalyticsApplicationOutputSchemaArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.schema = mapped
}
/**
* @param argument The Schema format of the data written to the destination. See Destination Schema below for more details.
*/
@JvmName("wcwkgiyebqusssdv")
public suspend fun schema(argument: suspend AnalyticsApplicationOutputSchemaArgsBuilder.() -> Unit) {
val toBeMapped = AnalyticsApplicationOutputSchemaArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.schema = mapped
}
internal fun build(): AnalyticsApplicationOutputArgs = AnalyticsApplicationOutputArgs(
id = id,
kinesisFirehose = kinesisFirehose,
kinesisStream = kinesisStream,
lambda = lambda,
name = name ?: throw PulumiNullFieldException("name"),
schema = schema ?: throw PulumiNullFieldException("schema"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy