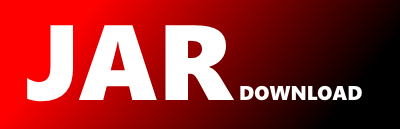
com.pulumi.aws.kinesis.kotlin.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.inputs
import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property enabled Defaults to `true`. Set it to `false` if you want to disable format conversion while preserving the configuration details.
* @property inputFormatConfiguration Specifies the deserializer that you want Kinesis Data Firehose to use to convert the format of your data from JSON. See `input_format_configuration` block below for details.
* @property outputFormatConfiguration Specifies the serializer that you want Kinesis Data Firehose to use to convert the format of your data to the Parquet or ORC format. See `output_format_configuration` block below for details.
* @property schemaConfiguration Specifies the AWS Glue Data Catalog table that contains the column information. See `schema_configuration` block below for details.
*/
public data class
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs(
public val enabled: Output? = null,
public val inputFormatConfiguration: Output,
public val outputFormatConfiguration: Output,
public val schemaConfiguration: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs =
com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs.builder()
.enabled(enabled?.applyValue({ args0 -> args0 }))
.inputFormatConfiguration(
inputFormatConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.outputFormatConfiguration(
outputFormatConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.schemaConfiguration(
schemaConfiguration.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs].
*/
@PulumiTagMarker
public class
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgsBuilder
internal constructor() {
private var enabled: Output? = null
private var inputFormatConfiguration:
Output? =
null
private var outputFormatConfiguration:
Output? =
null
private var schemaConfiguration:
Output? =
null
/**
* @param value Defaults to `true`. Set it to `false` if you want to disable format conversion while preserving the configuration details.
*/
@JvmName("hdlcrxplayirmsnk")
public suspend fun enabled(`value`: Output) {
this.enabled = value
}
/**
* @param value Specifies the deserializer that you want Kinesis Data Firehose to use to convert the format of your data from JSON. See `input_format_configuration` block below for details.
*/
@JvmName("ifnutaoibfsniqbx")
public suspend fun inputFormatConfiguration(`value`: Output) {
this.inputFormatConfiguration = value
}
/**
* @param value Specifies the serializer that you want Kinesis Data Firehose to use to convert the format of your data to the Parquet or ORC format. See `output_format_configuration` block below for details.
*/
@JvmName("lgfxdlxtohlbmhwo")
public suspend fun outputFormatConfiguration(`value`: Output) {
this.outputFormatConfiguration = value
}
/**
* @param value Specifies the AWS Glue Data Catalog table that contains the column information. See `schema_configuration` block below for details.
*/
@JvmName("lkuwwrgywbvyhogj")
public suspend fun schemaConfiguration(`value`: Output) {
this.schemaConfiguration = value
}
/**
* @param value Defaults to `true`. Set it to `false` if you want to disable format conversion while preserving the configuration details.
*/
@JvmName("herhkdncnlctuuhi")
public suspend fun enabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enabled = mapped
}
/**
* @param value Specifies the deserializer that you want Kinesis Data Firehose to use to convert the format of your data from JSON. See `input_format_configuration` block below for details.
*/
@JvmName("xmnhedohylabjdna")
public suspend fun inputFormatConfiguration(`value`: FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationInputFormatConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.inputFormatConfiguration = mapped
}
/**
* @param argument Specifies the deserializer that you want Kinesis Data Firehose to use to convert the format of your data from JSON. See `input_format_configuration` block below for details.
*/
@JvmName("sotlgkhuprnrvcpr")
public suspend fun inputFormatConfiguration(argument: suspend FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationInputFormatConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationInputFormatConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.inputFormatConfiguration = mapped
}
/**
* @param value Specifies the serializer that you want Kinesis Data Firehose to use to convert the format of your data to the Parquet or ORC format. See `output_format_configuration` block below for details.
*/
@JvmName("yycnxttcmtpvvtgy")
public suspend fun outputFormatConfiguration(`value`: FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationOutputFormatConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.outputFormatConfiguration = mapped
}
/**
* @param argument Specifies the serializer that you want Kinesis Data Firehose to use to convert the format of your data to the Parquet or ORC format. See `output_format_configuration` block below for details.
*/
@JvmName("gvmfugymsbamadrk")
public suspend fun outputFormatConfiguration(argument: suspend FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationOutputFormatConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationOutputFormatConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.outputFormatConfiguration = mapped
}
/**
* @param value Specifies the AWS Glue Data Catalog table that contains the column information. See `schema_configuration` block below for details.
*/
@JvmName("vlolsmpjcqdkaono")
public suspend fun schemaConfiguration(`value`: FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationSchemaConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.schemaConfiguration = mapped
}
/**
* @param argument Specifies the AWS Glue Data Catalog table that contains the column information. See `schema_configuration` block below for details.
*/
@JvmName("hfispdtcdwrdyyfs")
public suspend fun schemaConfiguration(argument: suspend FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationSchemaConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationSchemaConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.schemaConfiguration = mapped
}
internal fun build(): FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs =
FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfigurationArgs(
enabled = enabled,
inputFormatConfiguration = inputFormatConfiguration ?: throw
PulumiNullFieldException("inputFormatConfiguration"),
outputFormatConfiguration = outputFormatConfiguration ?: throw
PulumiNullFieldException("outputFormatConfiguration"),
schemaConfiguration = schemaConfiguration ?: throw PulumiNullFieldException("schemaConfiguration"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy