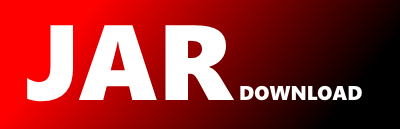
com.pulumi.aws.kinesis.kotlin.inputs.FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.inputs
import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bucketArn The ARN of the S3 bucket
* @property bufferingInterval Buffer incoming data for the specified period of time, in seconds, before delivering it to the destination. The default value is 300.
* @property bufferingSize Buffer incoming data to the specified size, in MBs, before delivering it to the destination. The default value is 5.
* We recommend setting SizeInMBs to a value greater than the amount of data you typically ingest into the delivery stream in 10 seconds. For example, if you typically ingest data at 1 MB/sec set SizeInMBs to be 10 MB or higher.
* @property cloudwatchLoggingOptions The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
* @property compressionFormat The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
* @property errorOutputPrefix Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
* @property kmsKeyArn Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
* @property prefix The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
* @property roleArn The ARN of the AWS credentials.
*/
public data class FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs(
public val bucketArn: Output,
public val bufferingInterval: Output? = null,
public val bufferingSize: Output? = null,
public val cloudwatchLoggingOptions: Output? =
null,
public val compressionFormat: Output? = null,
public val errorOutputPrefix: Output? = null,
public val kmsKeyArn: Output? = null,
public val prefix: Output? = null,
public val roleArn: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs =
com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs.builder()
.bucketArn(bucketArn.applyValue({ args0 -> args0 }))
.bufferingInterval(bufferingInterval?.applyValue({ args0 -> args0 }))
.bufferingSize(bufferingSize?.applyValue({ args0 -> args0 }))
.cloudwatchLoggingOptions(
cloudwatchLoggingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.compressionFormat(compressionFormat?.applyValue({ args0 -> args0 }))
.errorOutputPrefix(errorOutputPrefix?.applyValue({ args0 -> args0 }))
.kmsKeyArn(kmsKeyArn?.applyValue({ args0 -> args0 }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs].
*/
@PulumiTagMarker
public class FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgsBuilder internal constructor() {
private var bucketArn: Output? = null
private var bufferingInterval: Output? = null
private var bufferingSize: Output? = null
private var cloudwatchLoggingOptions:
Output? =
null
private var compressionFormat: Output? = null
private var errorOutputPrefix: Output? = null
private var kmsKeyArn: Output? = null
private var prefix: Output? = null
private var roleArn: Output? = null
/**
* @param value The ARN of the S3 bucket
*/
@JvmName("bwldpusnpwyjpryt")
public suspend fun bucketArn(`value`: Output) {
this.bucketArn = value
}
/**
* @param value Buffer incoming data for the specified period of time, in seconds, before delivering it to the destination. The default value is 300.
*/
@JvmName("elymxbfjdhdygkwf")
public suspend fun bufferingInterval(`value`: Output) {
this.bufferingInterval = value
}
/**
* @param value Buffer incoming data to the specified size, in MBs, before delivering it to the destination. The default value is 5.
* We recommend setting SizeInMBs to a value greater than the amount of data you typically ingest into the delivery stream in 10 seconds. For example, if you typically ingest data at 1 MB/sec set SizeInMBs to be 10 MB or higher.
*/
@JvmName("asewoftrwduygomx")
public suspend fun bufferingSize(`value`: Output) {
this.bufferingSize = value
}
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("ymlqbbibmisomkpn")
public suspend fun cloudwatchLoggingOptions(`value`: Output) {
this.cloudwatchLoggingOptions = value
}
/**
* @param value The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
*/
@JvmName("yboiiyvpoxfodqdk")
public suspend fun compressionFormat(`value`: Output) {
this.compressionFormat = value
}
/**
* @param value Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
*/
@JvmName("hjpowccaepknyrae")
public suspend fun errorOutputPrefix(`value`: Output) {
this.errorOutputPrefix = value
}
/**
* @param value Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
*/
@JvmName("vhryhrpfijiqkhhn")
public suspend fun kmsKeyArn(`value`: Output) {
this.kmsKeyArn = value
}
/**
* @param value The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
*/
@JvmName("sordplftigmlokql")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value The ARN of the AWS credentials.
*/
@JvmName("kqylcamarpyexqgp")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The ARN of the S3 bucket
*/
@JvmName("vyeahkygysendsqi")
public suspend fun bucketArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucketArn = mapped
}
/**
* @param value Buffer incoming data for the specified period of time, in seconds, before delivering it to the destination. The default value is 300.
*/
@JvmName("spdtemfbsqxgeusm")
public suspend fun bufferingInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufferingInterval = mapped
}
/**
* @param value Buffer incoming data to the specified size, in MBs, before delivering it to the destination. The default value is 5.
* We recommend setting SizeInMBs to a value greater than the amount of data you typically ingest into the delivery stream in 10 seconds. For example, if you typically ingest data at 1 MB/sec set SizeInMBs to be 10 MB or higher.
*/
@JvmName("shtfixngobeanpqp")
public suspend fun bufferingSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufferingSize = mapped
}
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("iokdgwejyqbgvflj")
public suspend fun cloudwatchLoggingOptions(`value`: FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationCloudwatchLoggingOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudwatchLoggingOptions = mapped
}
/**
* @param argument The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("pukxxdyltgdywnyu")
public suspend fun cloudwatchLoggingOptions(argument: suspend FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationCloudwatchLoggingOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationCloudwatchLoggingOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudwatchLoggingOptions = mapped
}
/**
* @param value The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
*/
@JvmName("awerxcjamnjxmlni")
public suspend fun compressionFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.compressionFormat = mapped
}
/**
* @param value Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
*/
@JvmName("tbdcqjwtdhlxajbr")
public suspend fun errorOutputPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.errorOutputPrefix = mapped
}
/**
* @param value Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
*/
@JvmName("jkierjpquduvvbck")
public suspend fun kmsKeyArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyArn = mapped
}
/**
* @param value The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
*/
@JvmName("xigpmaxluthglqoj")
public suspend fun prefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefix = mapped
}
/**
* @param value The ARN of the AWS credentials.
*/
@JvmName("ulfpulfkbtgympjt")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
internal fun build(): FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs =
FirehoseDeliveryStreamHttpEndpointConfigurationS3ConfigurationArgs(
bucketArn = bucketArn ?: throw PulumiNullFieldException("bucketArn"),
bufferingInterval = bufferingInterval,
bufferingSize = bufferingSize,
cloudwatchLoggingOptions = cloudwatchLoggingOptions,
compressionFormat = compressionFormat,
errorOutputPrefix = errorOutputPrefix,
kmsKeyArn = kmsKeyArn,
prefix = prefix,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy