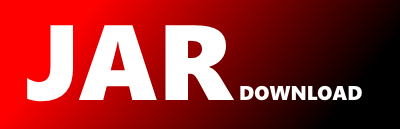
com.pulumi.aws.kinesis.kotlin.inputs.FirehoseDeliveryStreamOpensearchConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.inputs
import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamOpensearchConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bufferingInterval Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
* @property bufferingSize Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
* @property cloudwatchLoggingOptions The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
* @property clusterEndpoint The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
* @property documentIdOptions The method for setting up document ID. See [`document_id_options` block] below for details.
* @property domainArn The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
* @property indexName The OpenSearch index name.
* @property indexRotationPeriod The OpenSearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
* @property processingConfiguration The data processing configuration. See `processing_configuration` block below for details.
* @property retryDuration After an initial failure to deliver to Amazon OpenSearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
* @property roleArn The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeDomain`, `DescribeDomains`, and `DescribeDomainConfig`. The pattern needs to be `arn:.*`.
* @property s3BackupMode Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
* @property s3Configuration The S3 Configuration. See `s3_configuration` block below for details.
* @property typeName The Elasticsearch type name with maximum length of 100 characters. Types are deprecated in OpenSearch_1.1. TypeName must be empty.
* @property vpcConfig The VPC configuration for the delivery stream to connect to OpenSearch associated with the VPC. See `vpc_config` block below for details.
*/
public data class FirehoseDeliveryStreamOpensearchConfigurationArgs(
public val bufferingInterval: Output? = null,
public val bufferingSize: Output? = null,
public val cloudwatchLoggingOptions: Output? = null,
public val clusterEndpoint: Output? = null,
public val documentIdOptions: Output? = null,
public val domainArn: Output? = null,
public val indexName: Output,
public val indexRotationPeriod: Output? = null,
public val processingConfiguration: Output? = null,
public val retryDuration: Output? = null,
public val roleArn: Output,
public val s3BackupMode: Output? = null,
public val s3Configuration: Output,
public val typeName: Output? = null,
public val vpcConfig: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamOpensearchConfigurationArgs =
com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamOpensearchConfigurationArgs.builder()
.bufferingInterval(bufferingInterval?.applyValue({ args0 -> args0 }))
.bufferingSize(bufferingSize?.applyValue({ args0 -> args0 }))
.cloudwatchLoggingOptions(
cloudwatchLoggingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.clusterEndpoint(clusterEndpoint?.applyValue({ args0 -> args0 }))
.documentIdOptions(documentIdOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.domainArn(domainArn?.applyValue({ args0 -> args0 }))
.indexName(indexName.applyValue({ args0 -> args0 }))
.indexRotationPeriod(indexRotationPeriod?.applyValue({ args0 -> args0 }))
.processingConfiguration(
processingConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.retryDuration(retryDuration?.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.s3BackupMode(s3BackupMode?.applyValue({ args0 -> args0 }))
.s3Configuration(s3Configuration.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.typeName(typeName?.applyValue({ args0 -> args0 }))
.vpcConfig(vpcConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FirehoseDeliveryStreamOpensearchConfigurationArgs].
*/
@PulumiTagMarker
public class FirehoseDeliveryStreamOpensearchConfigurationArgsBuilder internal constructor() {
private var bufferingInterval: Output? = null
private var bufferingSize: Output? = null
private var cloudwatchLoggingOptions:
Output? = null
private var clusterEndpoint: Output? = null
private var documentIdOptions:
Output? = null
private var domainArn: Output? = null
private var indexName: Output? = null
private var indexRotationPeriod: Output? = null
private var processingConfiguration:
Output? = null
private var retryDuration: Output? = null
private var roleArn: Output? = null
private var s3BackupMode: Output? = null
private var s3Configuration:
Output? = null
private var typeName: Output? = null
private var vpcConfig: Output? = null
/**
* @param value Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
*/
@JvmName("ncgjljlbophpmbey")
public suspend fun bufferingInterval(`value`: Output) {
this.bufferingInterval = value
}
/**
* @param value Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
*/
@JvmName("uwhblmceyomedwfp")
public suspend fun bufferingSize(`value`: Output) {
this.bufferingSize = value
}
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("joiicuwydmeibwly")
public suspend fun cloudwatchLoggingOptions(`value`: Output) {
this.cloudwatchLoggingOptions = value
}
/**
* @param value The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
*/
@JvmName("fbjjfwtgxxgfpcyx")
public suspend fun clusterEndpoint(`value`: Output) {
this.clusterEndpoint = value
}
/**
* @param value The method for setting up document ID. See [`document_id_options` block] below for details.
*/
@JvmName("ejfbgdvaatiqyuvv")
public suspend fun documentIdOptions(`value`: Output) {
this.documentIdOptions = value
}
/**
* @param value The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
*/
@JvmName("wefwjieuncvdyroa")
public suspend fun domainArn(`value`: Output) {
this.domainArn = value
}
/**
* @param value The OpenSearch index name.
*/
@JvmName("fbxlbhnnqmfjndwx")
public suspend fun indexName(`value`: Output) {
this.indexName = value
}
/**
* @param value The OpenSearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
*/
@JvmName("svvqnvfttkacrvhj")
public suspend fun indexRotationPeriod(`value`: Output) {
this.indexRotationPeriod = value
}
/**
* @param value The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("lhlkswubnsstydya")
public suspend fun processingConfiguration(`value`: Output) {
this.processingConfiguration = value
}
/**
* @param value After an initial failure to deliver to Amazon OpenSearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
*/
@JvmName("nwmlmxmubhkicnwe")
public suspend fun retryDuration(`value`: Output) {
this.retryDuration = value
}
/**
* @param value The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeDomain`, `DescribeDomains`, and `DescribeDomainConfig`. The pattern needs to be `arn:.*`.
*/
@JvmName("atkipeftretgfkth")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
*/
@JvmName("fckkmfvefpolbrex")
public suspend fun s3BackupMode(`value`: Output) {
this.s3BackupMode = value
}
/**
* @param value The S3 Configuration. See `s3_configuration` block below for details.
*/
@JvmName("tkotbfjmfopypgqv")
public suspend fun s3Configuration(`value`: Output) {
this.s3Configuration = value
}
/**
* @param value The Elasticsearch type name with maximum length of 100 characters. Types are deprecated in OpenSearch_1.1. TypeName must be empty.
*/
@JvmName("cpygoqucbudnnrct")
public suspend fun typeName(`value`: Output) {
this.typeName = value
}
/**
* @param value The VPC configuration for the delivery stream to connect to OpenSearch associated with the VPC. See `vpc_config` block below for details.
*/
@JvmName("meankwlfrlitudbi")
public suspend fun vpcConfig(`value`: Output) {
this.vpcConfig = value
}
/**
* @param value Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
*/
@JvmName("gxcspccdemxbpbby")
public suspend fun bufferingInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufferingInterval = mapped
}
/**
* @param value Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
*/
@JvmName("rjlxrpparwutciry")
public suspend fun bufferingSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufferingSize = mapped
}
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("tsooitjftnjtcjul")
public suspend fun cloudwatchLoggingOptions(`value`: FirehoseDeliveryStreamOpensearchConfigurationCloudwatchLoggingOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudwatchLoggingOptions = mapped
}
/**
* @param argument The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("xqtelstubibxrjrp")
public suspend fun cloudwatchLoggingOptions(argument: suspend FirehoseDeliveryStreamOpensearchConfigurationCloudwatchLoggingOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamOpensearchConfigurationCloudwatchLoggingOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudwatchLoggingOptions = mapped
}
/**
* @param value The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
*/
@JvmName("nnqcurvqnjcrafva")
public suspend fun clusterEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterEndpoint = mapped
}
/**
* @param value The method for setting up document ID. See [`document_id_options` block] below for details.
*/
@JvmName("gikfauowutjgdyee")
public suspend fun documentIdOptions(`value`: FirehoseDeliveryStreamOpensearchConfigurationDocumentIdOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.documentIdOptions = mapped
}
/**
* @param argument The method for setting up document ID. See [`document_id_options` block] below for details.
*/
@JvmName("checjocugwomqrax")
public suspend fun documentIdOptions(argument: suspend FirehoseDeliveryStreamOpensearchConfigurationDocumentIdOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamOpensearchConfigurationDocumentIdOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.documentIdOptions = mapped
}
/**
* @param value The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
*/
@JvmName("kvpemntbssavhgsa")
public suspend fun domainArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.domainArn = mapped
}
/**
* @param value The OpenSearch index name.
*/
@JvmName("dxuapbtfqebcbtfb")
public suspend fun indexName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.indexName = mapped
}
/**
* @param value The OpenSearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
*/
@JvmName("rahhsugkdyakcjay")
public suspend fun indexRotationPeriod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.indexRotationPeriod = mapped
}
/**
* @param value The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("nfevjedkyvhuetyb")
public suspend fun processingConfiguration(`value`: FirehoseDeliveryStreamOpensearchConfigurationProcessingConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.processingConfiguration = mapped
}
/**
* @param argument The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("bldlkldfxbffbxjk")
public suspend fun processingConfiguration(argument: suspend FirehoseDeliveryStreamOpensearchConfigurationProcessingConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamOpensearchConfigurationProcessingConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.processingConfiguration = mapped
}
/**
* @param value After an initial failure to deliver to Amazon OpenSearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
*/
@JvmName("lngxoapwdkkwshdq")
public suspend fun retryDuration(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryDuration = mapped
}
/**
* @param value The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeDomain`, `DescribeDomains`, and `DescribeDomainConfig`. The pattern needs to be `arn:.*`.
*/
@JvmName("toagwutvfjofdgiq")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
*/
@JvmName("lbkhweyyjqolpjws")
public suspend fun s3BackupMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BackupMode = mapped
}
/**
* @param value The S3 Configuration. See `s3_configuration` block below for details.
*/
@JvmName("ihtixxnicvyvwddj")
public suspend fun s3Configuration(`value`: FirehoseDeliveryStreamOpensearchConfigurationS3ConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.s3Configuration = mapped
}
/**
* @param argument The S3 Configuration. See `s3_configuration` block below for details.
*/
@JvmName("kgcdpxfxmhpggmyl")
public suspend fun s3Configuration(argument: suspend FirehoseDeliveryStreamOpensearchConfigurationS3ConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamOpensearchConfigurationS3ConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.s3Configuration = mapped
}
/**
* @param value The Elasticsearch type name with maximum length of 100 characters. Types are deprecated in OpenSearch_1.1. TypeName must be empty.
*/
@JvmName("mkkgsbrkidmkqwhy")
public suspend fun typeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.typeName = mapped
}
/**
* @param value The VPC configuration for the delivery stream to connect to OpenSearch associated with the VPC. See `vpc_config` block below for details.
*/
@JvmName("dgtodjixautlypbp")
public suspend fun vpcConfig(`value`: FirehoseDeliveryStreamOpensearchConfigurationVpcConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vpcConfig = mapped
}
/**
* @param argument The VPC configuration for the delivery stream to connect to OpenSearch associated with the VPC. See `vpc_config` block below for details.
*/
@JvmName("tpfdnggmsnchvqis")
public suspend fun vpcConfig(argument: suspend FirehoseDeliveryStreamOpensearchConfigurationVpcConfigArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamOpensearchConfigurationVpcConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.vpcConfig = mapped
}
internal fun build(): FirehoseDeliveryStreamOpensearchConfigurationArgs =
FirehoseDeliveryStreamOpensearchConfigurationArgs(
bufferingInterval = bufferingInterval,
bufferingSize = bufferingSize,
cloudwatchLoggingOptions = cloudwatchLoggingOptions,
clusterEndpoint = clusterEndpoint,
documentIdOptions = documentIdOptions,
domainArn = domainArn,
indexName = indexName ?: throw PulumiNullFieldException("indexName"),
indexRotationPeriod = indexRotationPeriod,
processingConfiguration = processingConfiguration,
retryDuration = retryDuration,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
s3BackupMode = s3BackupMode,
s3Configuration = s3Configuration ?: throw PulumiNullFieldException("s3Configuration"),
typeName = typeName,
vpcConfig = vpcConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy