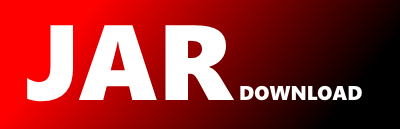
com.pulumi.aws.kinesis.kotlin.inputs.FirehoseDeliveryStreamRedshiftConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.inputs
import com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamRedshiftConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cloudwatchLoggingOptions The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
* @property clusterJdbcurl The jdbcurl of the redshift cluster.
* @property copyOptions Copy options for copying the data from the s3 intermediate bucket into redshift, for example to change the default delimiter. For valid values, see the [AWS documentation](http://docs.aws.amazon.com/firehose/latest/APIReference/API_CopyCommand.html)
* @property dataTableColumns The data table columns that will be targeted by the copy command.
* @property dataTableName The name of the table in the redshift cluster that the s3 bucket will copy to.
* @property password The password for the username above. This value is required if `secrets_manager_configuration` is not provided.
* @property processingConfiguration The data processing configuration. See `processing_configuration` block below for details.
* @property retryDuration The length of time during which Firehose retries delivery after a failure, starting from the initial request and including the first attempt. The default value is 3600 seconds (60 minutes). Firehose does not retry if the value of DurationInSeconds is 0 (zero) or if the first delivery attempt takes longer than the current value.
* @property roleArn The arn of the role the stream assumes.
* @property s3BackupConfiguration The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
* `secrets_manager_configuration` - (Optional) The Secrets Manager configuration. See `secrets_manager_configuration` block below for details. This value is required if `username` and `password` are not provided.
* @property s3BackupMode The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
* @property s3Configuration The S3 Configuration. See s3_configuration below for details.
* @property secretsManagerConfiguration
* @property username The username that the firehose delivery stream will assume. It is strongly recommended that the username and password provided is used exclusively for Amazon Kinesis Firehose purposes, and that the permissions for the account are restricted for Amazon Redshift INSERT permissions. This value is required if `secrets_manager_configuration` is not provided.
*/
public data class FirehoseDeliveryStreamRedshiftConfigurationArgs(
public val cloudwatchLoggingOptions: Output? = null,
public val clusterJdbcurl: Output,
public val copyOptions: Output? = null,
public val dataTableColumns: Output? = null,
public val dataTableName: Output,
public val password: Output? = null,
public val processingConfiguration: Output? = null,
public val retryDuration: Output? = null,
public val roleArn: Output,
public val s3BackupConfiguration: Output? = null,
public val s3BackupMode: Output? = null,
public val s3Configuration: Output,
public val secretsManagerConfiguration: Output? = null,
public val username: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamRedshiftConfigurationArgs =
com.pulumi.aws.kinesis.inputs.FirehoseDeliveryStreamRedshiftConfigurationArgs.builder()
.cloudwatchLoggingOptions(
cloudwatchLoggingOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.clusterJdbcurl(clusterJdbcurl.applyValue({ args0 -> args0 }))
.copyOptions(copyOptions?.applyValue({ args0 -> args0 }))
.dataTableColumns(dataTableColumns?.applyValue({ args0 -> args0 }))
.dataTableName(dataTableName.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.processingConfiguration(
processingConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.retryDuration(retryDuration?.applyValue({ args0 -> args0 }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.s3BackupConfiguration(
s3BackupConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.s3BackupMode(s3BackupMode?.applyValue({ args0 -> args0 }))
.s3Configuration(s3Configuration.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secretsManagerConfiguration(
secretsManagerConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.username(username?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FirehoseDeliveryStreamRedshiftConfigurationArgs].
*/
@PulumiTagMarker
public class FirehoseDeliveryStreamRedshiftConfigurationArgsBuilder internal constructor() {
private var cloudwatchLoggingOptions:
Output? = null
private var clusterJdbcurl: Output? = null
private var copyOptions: Output? = null
private var dataTableColumns: Output? = null
private var dataTableName: Output? = null
private var password: Output? = null
private var processingConfiguration:
Output? = null
private var retryDuration: Output? = null
private var roleArn: Output? = null
private var s3BackupConfiguration:
Output? = null
private var s3BackupMode: Output? = null
private var s3Configuration:
Output? = null
private var secretsManagerConfiguration:
Output? = null
private var username: Output? = null
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("aawkcqfjbaugqdah")
public suspend fun cloudwatchLoggingOptions(`value`: Output) {
this.cloudwatchLoggingOptions = value
}
/**
* @param value The jdbcurl of the redshift cluster.
*/
@JvmName("bjgqwqixoyfqbclx")
public suspend fun clusterJdbcurl(`value`: Output) {
this.clusterJdbcurl = value
}
/**
* @param value Copy options for copying the data from the s3 intermediate bucket into redshift, for example to change the default delimiter. For valid values, see the [AWS documentation](http://docs.aws.amazon.com/firehose/latest/APIReference/API_CopyCommand.html)
*/
@JvmName("wvituuprgeiejlsf")
public suspend fun copyOptions(`value`: Output) {
this.copyOptions = value
}
/**
* @param value The data table columns that will be targeted by the copy command.
*/
@JvmName("pfliesihqjylymkm")
public suspend fun dataTableColumns(`value`: Output) {
this.dataTableColumns = value
}
/**
* @param value The name of the table in the redshift cluster that the s3 bucket will copy to.
*/
@JvmName("roxqpmpabgufwumf")
public suspend fun dataTableName(`value`: Output) {
this.dataTableName = value
}
/**
* @param value The password for the username above. This value is required if `secrets_manager_configuration` is not provided.
*/
@JvmName("modlvuotpqygebfc")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("jejbmxeibffxlwdp")
public suspend fun processingConfiguration(`value`: Output) {
this.processingConfiguration = value
}
/**
* @param value The length of time during which Firehose retries delivery after a failure, starting from the initial request and including the first attempt. The default value is 3600 seconds (60 minutes). Firehose does not retry if the value of DurationInSeconds is 0 (zero) or if the first delivery attempt takes longer than the current value.
*/
@JvmName("bbjfcxfbimppmcfa")
public suspend fun retryDuration(`value`: Output) {
this.retryDuration = value
}
/**
* @param value The arn of the role the stream assumes.
*/
@JvmName("fehnboxlfpdkydrr")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
* `secrets_manager_configuration` - (Optional) The Secrets Manager configuration. See `secrets_manager_configuration` block below for details. This value is required if `username` and `password` are not provided.
*/
@JvmName("ntovphcbydhspymw")
public suspend fun s3BackupConfiguration(`value`: Output) {
this.s3BackupConfiguration = value
}
/**
* @param value The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
*/
@JvmName("hypqnfkquimcriuy")
public suspend fun s3BackupMode(`value`: Output) {
this.s3BackupMode = value
}
/**
* @param value The S3 Configuration. See s3_configuration below for details.
*/
@JvmName("yhvtnaijhulojgni")
public suspend fun s3Configuration(`value`: Output) {
this.s3Configuration = value
}
/**
* @param value
*/
@JvmName("vvpmnlqxbtlwjtlx")
public suspend fun secretsManagerConfiguration(`value`: Output) {
this.secretsManagerConfiguration = value
}
/**
* @param value The username that the firehose delivery stream will assume. It is strongly recommended that the username and password provided is used exclusively for Amazon Kinesis Firehose purposes, and that the permissions for the account are restricted for Amazon Redshift INSERT permissions. This value is required if `secrets_manager_configuration` is not provided.
*/
@JvmName("pjqetdwfrqescqov")
public suspend fun username(`value`: Output) {
this.username = value
}
/**
* @param value The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("omwaaykbicxsdwlc")
public suspend fun cloudwatchLoggingOptions(`value`: FirehoseDeliveryStreamRedshiftConfigurationCloudwatchLoggingOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudwatchLoggingOptions = mapped
}
/**
* @param argument The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
*/
@JvmName("ywyjwhpydcavmqtb")
public suspend fun cloudwatchLoggingOptions(argument: suspend FirehoseDeliveryStreamRedshiftConfigurationCloudwatchLoggingOptionsArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamRedshiftConfigurationCloudwatchLoggingOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudwatchLoggingOptions = mapped
}
/**
* @param value The jdbcurl of the redshift cluster.
*/
@JvmName("hnkppioppspgdbjs")
public suspend fun clusterJdbcurl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.clusterJdbcurl = mapped
}
/**
* @param value Copy options for copying the data from the s3 intermediate bucket into redshift, for example to change the default delimiter. For valid values, see the [AWS documentation](http://docs.aws.amazon.com/firehose/latest/APIReference/API_CopyCommand.html)
*/
@JvmName("gbmupdkluggdymcg")
public suspend fun copyOptions(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.copyOptions = mapped
}
/**
* @param value The data table columns that will be targeted by the copy command.
*/
@JvmName("yirdhxvvaftivmhp")
public suspend fun dataTableColumns(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataTableColumns = mapped
}
/**
* @param value The name of the table in the redshift cluster that the s3 bucket will copy to.
*/
@JvmName("ydxwuunqklvynkup")
public suspend fun dataTableName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.dataTableName = mapped
}
/**
* @param value The password for the username above. This value is required if `secrets_manager_configuration` is not provided.
*/
@JvmName("ofjviqudsspwxpwf")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("xxcvdmlwmnfpjjce")
public suspend fun processingConfiguration(`value`: FirehoseDeliveryStreamRedshiftConfigurationProcessingConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.processingConfiguration = mapped
}
/**
* @param argument The data processing configuration. See `processing_configuration` block below for details.
*/
@JvmName("adulefwxjlnqkadj")
public suspend fun processingConfiguration(argument: suspend FirehoseDeliveryStreamRedshiftConfigurationProcessingConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamRedshiftConfigurationProcessingConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.processingConfiguration = mapped
}
/**
* @param value The length of time during which Firehose retries delivery after a failure, starting from the initial request and including the first attempt. The default value is 3600 seconds (60 minutes). Firehose does not retry if the value of DurationInSeconds is 0 (zero) or if the first delivery attempt takes longer than the current value.
*/
@JvmName("vmlyehpuwpujpxyg")
public suspend fun retryDuration(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryDuration = mapped
}
/**
* @param value The arn of the role the stream assumes.
*/
@JvmName("sssmvfyjlbcpgggf")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
* `secrets_manager_configuration` - (Optional) The Secrets Manager configuration. See `secrets_manager_configuration` block below for details. This value is required if `username` and `password` are not provided.
*/
@JvmName("cgncxtjsvhwkimqr")
public suspend fun s3BackupConfiguration(`value`: FirehoseDeliveryStreamRedshiftConfigurationS3BackupConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BackupConfiguration = mapped
}
/**
* @param argument The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
* `secrets_manager_configuration` - (Optional) The Secrets Manager configuration. See `secrets_manager_configuration` block below for details. This value is required if `username` and `password` are not provided.
*/
@JvmName("rvxohkohgtvgrilc")
public suspend fun s3BackupConfiguration(argument: suspend FirehoseDeliveryStreamRedshiftConfigurationS3BackupConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamRedshiftConfigurationS3BackupConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.s3BackupConfiguration = mapped
}
/**
* @param value The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
*/
@JvmName("pvcvrecglmtidxxj")
public suspend fun s3BackupMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3BackupMode = mapped
}
/**
* @param value The S3 Configuration. See s3_configuration below for details.
*/
@JvmName("yvbigpforxowttwc")
public suspend fun s3Configuration(`value`: FirehoseDeliveryStreamRedshiftConfigurationS3ConfigurationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.s3Configuration = mapped
}
/**
* @param argument The S3 Configuration. See s3_configuration below for details.
*/
@JvmName("kqwtkoaypcpiupet")
public suspend fun s3Configuration(argument: suspend FirehoseDeliveryStreamRedshiftConfigurationS3ConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamRedshiftConfigurationS3ConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.s3Configuration = mapped
}
/**
* @param value
*/
@JvmName("dlvukaavbcdtwxrs")
public suspend fun secretsManagerConfiguration(`value`: FirehoseDeliveryStreamRedshiftConfigurationSecretsManagerConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretsManagerConfiguration = mapped
}
/**
* @param argument
*/
@JvmName("thgkdyfurkcwnjfx")
public suspend fun secretsManagerConfiguration(argument: suspend FirehoseDeliveryStreamRedshiftConfigurationSecretsManagerConfigurationArgsBuilder.() -> Unit) {
val toBeMapped =
FirehoseDeliveryStreamRedshiftConfigurationSecretsManagerConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.secretsManagerConfiguration = mapped
}
/**
* @param value The username that the firehose delivery stream will assume. It is strongly recommended that the username and password provided is used exclusively for Amazon Kinesis Firehose purposes, and that the permissions for the account are restricted for Amazon Redshift INSERT permissions. This value is required if `secrets_manager_configuration` is not provided.
*/
@JvmName("eukqqkqtvotbwrwr")
public suspend fun username(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.username = mapped
}
internal fun build(): FirehoseDeliveryStreamRedshiftConfigurationArgs =
FirehoseDeliveryStreamRedshiftConfigurationArgs(
cloudwatchLoggingOptions = cloudwatchLoggingOptions,
clusterJdbcurl = clusterJdbcurl ?: throw PulumiNullFieldException("clusterJdbcurl"),
copyOptions = copyOptions,
dataTableColumns = dataTableColumns,
dataTableName = dataTableName ?: throw PulumiNullFieldException("dataTableName"),
password = password,
processingConfiguration = processingConfiguration,
retryDuration = retryDuration,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
s3BackupConfiguration = s3BackupConfiguration,
s3BackupMode = s3BackupMode,
s3Configuration = s3Configuration ?: throw PulumiNullFieldException("s3Configuration"),
secretsManagerConfiguration = secretsManagerConfiguration,
username = username,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy