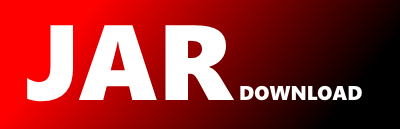
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3Configuration.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property bucketArn The ARN of the S3 bucket
* @property bufferingInterval
* @property bufferingSize
* @property cloudwatchLoggingOptions
* @property compressionFormat The compression format. If no value is specified, the default is `UNCOMPRESSED`. Other supported values are `GZIP`, `ZIP`, `Snappy`, & `HADOOP_SNAPPY`.
* @property customTimeZone The time zone you prefer. Valid values are `UTC` or a non-3-letter IANA time zones (for example, `America/Los_Angeles`). Default value is `UTC`.
* @property dataFormatConversionConfiguration Nested argument for the serializer, deserializer, and schema for converting data from the JSON format to the Parquet or ORC format before writing it to Amazon S3. See `data_format_conversion_configuration` block below for details.
* @property dynamicPartitioningConfiguration The configuration for dynamic partitioning. Required when using [dynamic partitioning](https://docs.aws.amazon.com/firehose/latest/dev/dynamic-partitioning.html). See `dynamic_partitioning_configuration` block below for details.
* @property errorOutputPrefix Prefix added to failed records before writing them to S3. Not currently supported for `redshift` destination. This prefix appears immediately following the bucket name. For information about how to specify this prefix, see [Custom Prefixes for Amazon S3 Objects](https://docs.aws.amazon.com/firehose/latest/dev/s3-prefixes.html).
* @property fileExtension The file extension to override the default file extension (for example, `.json`).
* @property kmsKeyArn Specifies the KMS key ARN the stream will use to encrypt data. If not set, no encryption will
* be used.
* @property prefix The "YYYY/MM/DD/HH" time format prefix is automatically used for delivered S3 files. You can specify an extra prefix to be added in front of the time format prefix. Note that if the prefix ends with a slash, it appears as a folder in the S3 bucket
* @property processingConfiguration The data processing configuration. See `processing_configuration` block below for details.
* @property roleArn
* @property s3BackupConfiguration The configuration for backup in Amazon S3. Required if `s3_backup_mode` is `Enabled`. Supports the same fields as `s3_configuration` object.
* @property s3BackupMode The Amazon S3 backup mode. Valid values are `Disabled` and `Enabled`. Default value is `Disabled`.
*/
public data class FirehoseDeliveryStreamExtendedS3Configuration(
public val bucketArn: String,
public val bufferingInterval: Int? = null,
public val bufferingSize: Int? = null,
public val cloudwatchLoggingOptions: FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions? = null,
public val compressionFormat: String? = null,
public val customTimeZone: String? = null,
public val dataFormatConversionConfiguration: FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration? = null,
public val dynamicPartitioningConfiguration: FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration? = null,
public val errorOutputPrefix: String? = null,
public val fileExtension: String? = null,
public val kmsKeyArn: String? = null,
public val prefix: String? = null,
public val processingConfiguration: FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration? = null,
public val roleArn: String,
public val s3BackupConfiguration: FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration? = null,
public val s3BackupMode: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamExtendedS3Configuration): FirehoseDeliveryStreamExtendedS3Configuration =
FirehoseDeliveryStreamExtendedS3Configuration(
bucketArn = javaType.bucketArn(),
bufferingInterval = javaType.bufferingInterval().map({ args0 -> args0 }).orElse(null),
bufferingSize = javaType.bufferingSize().map({ args0 -> args0 }).orElse(null),
cloudwatchLoggingOptions = javaType.cloudwatchLoggingOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationCloudwatchLoggingOptions.Companion.toKotlin(args0)
})
}).orElse(null),
compressionFormat = javaType.compressionFormat().map({ args0 -> args0 }).orElse(null),
customTimeZone = javaType.customTimeZone().map({ args0 -> args0 }).orElse(null),
dataFormatConversionConfiguration = javaType.dataFormatConversionConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationDataFormatConversionConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
dynamicPartitioningConfiguration = javaType.dynamicPartitioningConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationDynamicPartitioningConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
errorOutputPrefix = javaType.errorOutputPrefix().map({ args0 -> args0 }).orElse(null),
fileExtension = javaType.fileExtension().map({ args0 -> args0 }).orElse(null),
kmsKeyArn = javaType.kmsKeyArn().map({ args0 -> args0 }).orElse(null),
prefix = javaType.prefix().map({ args0 -> args0 }).orElse(null),
processingConfiguration = javaType.processingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationProcessingConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
roleArn = javaType.roleArn(),
s3BackupConfiguration = javaType.s3BackupConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamExtendedS3ConfigurationS3BackupConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
s3BackupMode = javaType.s3BackupMode().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy