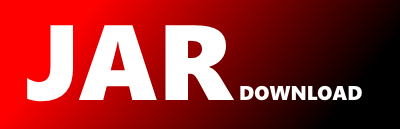
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesis.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property bufferingInterval Buffer incoming data for the specified period of time, in seconds between 0 to 900, before delivering it to the destination. The default value is 300s.
* @property bufferingSize Buffer incoming data to the specified size, in MBs between 1 to 100, before delivering it to the destination. The default value is 5MB.
* @property cloudwatchLoggingOptions The CloudWatch Logging Options for the delivery stream. See `cloudwatch_logging_options` block below for details.
* @property clusterEndpoint The endpoint to use when communicating with the cluster. Conflicts with `domain_arn`.
* @property documentIdOptions The method for setting up document ID. See [`document_id_options` block] below for details.
* @property domainArn The ARN of the Amazon ES domain. The pattern needs to be `arn:.*`. Conflicts with `cluster_endpoint`.
* @property indexName The OpenSearch index name.
* @property indexRotationPeriod The OpenSearch index rotation period. Index rotation appends a timestamp to the IndexName to facilitate expiration of old data. Valid values are `NoRotation`, `OneHour`, `OneDay`, `OneWeek`, and `OneMonth`. The default value is `OneDay`.
* @property processingConfiguration The data processing configuration. See `processing_configuration` block below for details.
* @property retryDuration After an initial failure to deliver to Amazon OpenSearch, the total amount of time, in seconds between 0 to 7200, during which Firehose re-attempts delivery (including the first attempt). After this time has elapsed, the failed documents are written to Amazon S3. The default value is 300s. There will be no retry if the value is 0.
* @property roleArn The ARN of the IAM role to be assumed by Firehose for calling the Amazon ES Configuration API and for indexing documents. The IAM role must have permission for `DescribeDomain`, `DescribeDomains`, and `DescribeDomainConfig`. The pattern needs to be `arn:.*`.
* @property s3BackupMode Defines how documents should be delivered to Amazon S3. Valid values are `FailedDocumentsOnly` and `AllDocuments`. Default value is `FailedDocumentsOnly`.
* @property s3Configuration The S3 Configuration. See `s3_configuration` block below for details.
* @property typeName The Elasticsearch type name with maximum length of 100 characters. Types are deprecated in OpenSearch_1.1. TypeName must be empty.
* @property vpcConfig The VPC configuration for the delivery stream to connect to OpenSearch associated with the VPC. See `vpc_config` block below for details.
*/
public data class FirehoseDeliveryStreamOpensearchConfiguration(
public val bufferingInterval: Int? = null,
public val bufferingSize: Int? = null,
public val cloudwatchLoggingOptions: FirehoseDeliveryStreamOpensearchConfigurationCloudwatchLoggingOptions? = null,
public val clusterEndpoint: String? = null,
public val documentIdOptions: FirehoseDeliveryStreamOpensearchConfigurationDocumentIdOptions? =
null,
public val domainArn: String? = null,
public val indexName: String,
public val indexRotationPeriod: String? = null,
public val processingConfiguration: FirehoseDeliveryStreamOpensearchConfigurationProcessingConfiguration? = null,
public val retryDuration: Int? = null,
public val roleArn: String,
public val s3BackupMode: String? = null,
public val s3Configuration: FirehoseDeliveryStreamOpensearchConfigurationS3Configuration,
public val typeName: String? = null,
public val vpcConfig: FirehoseDeliveryStreamOpensearchConfigurationVpcConfig? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.kinesis.outputs.FirehoseDeliveryStreamOpensearchConfiguration): FirehoseDeliveryStreamOpensearchConfiguration =
FirehoseDeliveryStreamOpensearchConfiguration(
bufferingInterval = javaType.bufferingInterval().map({ args0 -> args0 }).orElse(null),
bufferingSize = javaType.bufferingSize().map({ args0 -> args0 }).orElse(null),
cloudwatchLoggingOptions = javaType.cloudwatchLoggingOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfigurationCloudwatchLoggingOptions.Companion.toKotlin(args0)
})
}).orElse(null),
clusterEndpoint = javaType.clusterEndpoint().map({ args0 -> args0 }).orElse(null),
documentIdOptions = javaType.documentIdOptions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfigurationDocumentIdOptions.Companion.toKotlin(args0)
})
}).orElse(null),
domainArn = javaType.domainArn().map({ args0 -> args0 }).orElse(null),
indexName = javaType.indexName(),
indexRotationPeriod = javaType.indexRotationPeriod().map({ args0 -> args0 }).orElse(null),
processingConfiguration = javaType.processingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfigurationProcessingConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
retryDuration = javaType.retryDuration().map({ args0 -> args0 }).orElse(null),
roleArn = javaType.roleArn(),
s3BackupMode = javaType.s3BackupMode().map({ args0 -> args0 }).orElse(null),
s3Configuration = javaType.s3Configuration().let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfigurationS3Configuration.Companion.toKotlin(args0)
}),
typeName = javaType.typeName().map({ args0 -> args0 }).orElse(null),
vpcConfig = javaType.vpcConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.kinesis.kotlin.outputs.FirehoseDeliveryStreamOpensearchConfigurationVpcConfig.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy