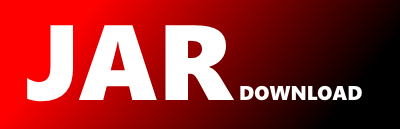
com.pulumi.aws.kinesisanalyticsv2.kotlin.inputs.ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kinesisanalyticsv2.kotlin.inputs
import com.pulumi.aws.kinesisanalyticsv2.inputs.ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property destinationSchema Describes the data format when records are written to the destination.
* @property kinesisFirehoseOutput Identifies a Kinesis Data Firehose delivery stream as the destination.
* @property kinesisStreamsOutput Identifies a Kinesis data stream as the destination.
* @property lambdaOutput Identifies a Lambda function as the destination.
* @property name The name of the in-application stream.
* @property outputId
*/
public data class ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs(
public val destinationSchema: Output,
public val kinesisFirehoseOutput: Output? =
null,
public val kinesisStreamsOutput: Output? =
null,
public val lambdaOutput: Output? =
null,
public val name: Output,
public val outputId: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.kinesisanalyticsv2.inputs.ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs =
com.pulumi.aws.kinesisanalyticsv2.inputs.ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs.builder()
.destinationSchema(destinationSchema.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kinesisFirehoseOutput(
kinesisFirehoseOutput?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kinesisStreamsOutput(
kinesisStreamsOutput?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.lambdaOutput(lambdaOutput?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.outputId(outputId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs].
*/
@PulumiTagMarker
public class ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgsBuilder
internal constructor() {
private var destinationSchema:
Output? =
null
private var kinesisFirehoseOutput:
Output? =
null
private var kinesisStreamsOutput:
Output? =
null
private var lambdaOutput:
Output? =
null
private var name: Output? = null
private var outputId: Output? = null
/**
* @param value Describes the data format when records are written to the destination.
*/
@JvmName("eccmjkhgrhdbxdmy")
public suspend fun destinationSchema(`value`: Output) {
this.destinationSchema = value
}
/**
* @param value Identifies a Kinesis Data Firehose delivery stream as the destination.
*/
@JvmName("eflsvqkeeubaetqm")
public suspend fun kinesisFirehoseOutput(`value`: Output) {
this.kinesisFirehoseOutput = value
}
/**
* @param value Identifies a Kinesis data stream as the destination.
*/
@JvmName("pujyalcbfhsyyadi")
public suspend fun kinesisStreamsOutput(`value`: Output) {
this.kinesisStreamsOutput = value
}
/**
* @param value Identifies a Lambda function as the destination.
*/
@JvmName("sucupnoujtymiits")
public suspend fun lambdaOutput(`value`: Output) {
this.lambdaOutput = value
}
/**
* @param value The name of the in-application stream.
*/
@JvmName("ylqrpdguliptidxq")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("cwsfuxbabkqloypm")
public suspend fun outputId(`value`: Output) {
this.outputId = value
}
/**
* @param value Describes the data format when records are written to the destination.
*/
@JvmName("gsffrtqyeyswjdrx")
public suspend fun destinationSchema(`value`: ApplicationApplicationConfigurationSqlApplicationConfigurationOutputDestinationSchemaArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.destinationSchema = mapped
}
/**
* @param argument Describes the data format when records are written to the destination.
*/
@JvmName("agtkkrysuuhsmjvi")
public suspend fun destinationSchema(argument: suspend ApplicationApplicationConfigurationSqlApplicationConfigurationOutputDestinationSchemaArgsBuilder.() -> Unit) {
val toBeMapped =
ApplicationApplicationConfigurationSqlApplicationConfigurationOutputDestinationSchemaArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.destinationSchema = mapped
}
/**
* @param value Identifies a Kinesis Data Firehose delivery stream as the destination.
*/
@JvmName("hnopobqhtjlkxdro")
public suspend fun kinesisFirehoseOutput(`value`: ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisFirehoseOutputArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisFirehoseOutput = mapped
}
/**
* @param argument Identifies a Kinesis Data Firehose delivery stream as the destination.
*/
@JvmName("itbuufdcrrficduk")
public suspend fun kinesisFirehoseOutput(argument: suspend ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisFirehoseOutputArgsBuilder.() -> Unit) {
val toBeMapped =
ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisFirehoseOutputArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisFirehoseOutput = mapped
}
/**
* @param value Identifies a Kinesis data stream as the destination.
*/
@JvmName("quqelafepmrwnhfv")
public suspend fun kinesisStreamsOutput(`value`: ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisStreamsOutputArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisStreamsOutput = mapped
}
/**
* @param argument Identifies a Kinesis data stream as the destination.
*/
@JvmName("icbqfmfjgoebfoce")
public suspend fun kinesisStreamsOutput(argument: suspend ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisStreamsOutputArgsBuilder.() -> Unit) {
val toBeMapped =
ApplicationApplicationConfigurationSqlApplicationConfigurationOutputKinesisStreamsOutputArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.kinesisStreamsOutput = mapped
}
/**
* @param value Identifies a Lambda function as the destination.
*/
@JvmName("elgmfmueumbtauac")
public suspend fun lambdaOutput(`value`: ApplicationApplicationConfigurationSqlApplicationConfigurationOutputLambdaOutputArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lambdaOutput = mapped
}
/**
* @param argument Identifies a Lambda function as the destination.
*/
@JvmName("kthjwsgfgvoxkedc")
public suspend fun lambdaOutput(argument: suspend ApplicationApplicationConfigurationSqlApplicationConfigurationOutputLambdaOutputArgsBuilder.() -> Unit) {
val toBeMapped =
ApplicationApplicationConfigurationSqlApplicationConfigurationOutputLambdaOutputArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.lambdaOutput = mapped
}
/**
* @param value The name of the in-application stream.
*/
@JvmName("bjhutquwxuwinddr")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("ubgfvqpnujikfhly")
public suspend fun outputId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outputId = mapped
}
internal fun build(): ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs =
ApplicationApplicationConfigurationSqlApplicationConfigurationOutputArgs(
destinationSchema = destinationSchema ?: throw PulumiNullFieldException("destinationSchema"),
kinesisFirehoseOutput = kinesisFirehoseOutput,
kinesisStreamsOutput = kinesisStreamsOutput,
lambdaOutput = lambdaOutput,
name = name ?: throw PulumiNullFieldException("name"),
outputId = outputId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy