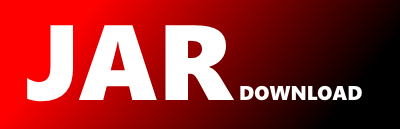
com.pulumi.aws.kms.kotlin.KmsFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kms.kotlin
import com.pulumi.aws.kms.KmsFunctions.getAliasPlain
import com.pulumi.aws.kms.KmsFunctions.getCipherTextPlain
import com.pulumi.aws.kms.KmsFunctions.getCustomKeyStorePlain
import com.pulumi.aws.kms.KmsFunctions.getKeyPlain
import com.pulumi.aws.kms.KmsFunctions.getPublicKeyPlain
import com.pulumi.aws.kms.KmsFunctions.getSecretPlain
import com.pulumi.aws.kms.KmsFunctions.getSecretsPlain
import com.pulumi.aws.kms.kotlin.inputs.GetAliasPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetAliasPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetCipherTextPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetCipherTextPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetCustomKeyStorePlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetCustomKeyStorePlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetKeyPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetKeyPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetPublicKeyPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetPublicKeyPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetSecretPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetSecretPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetSecretSecret
import com.pulumi.aws.kms.kotlin.inputs.GetSecretsPlainArgs
import com.pulumi.aws.kms.kotlin.inputs.GetSecretsPlainArgsBuilder
import com.pulumi.aws.kms.kotlin.inputs.GetSecretsSecret
import com.pulumi.aws.kms.kotlin.outputs.GetAliasResult
import com.pulumi.aws.kms.kotlin.outputs.GetCipherTextResult
import com.pulumi.aws.kms.kotlin.outputs.GetCustomKeyStoreResult
import com.pulumi.aws.kms.kotlin.outputs.GetKeyResult
import com.pulumi.aws.kms.kotlin.outputs.GetPublicKeyResult
import com.pulumi.aws.kms.kotlin.outputs.GetSecretResult
import com.pulumi.aws.kms.kotlin.outputs.GetSecretsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.aws.kms.kotlin.outputs.GetAliasResult.Companion.toKotlin as getAliasResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetCipherTextResult.Companion.toKotlin as getCipherTextResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetCustomKeyStoreResult.Companion.toKotlin as getCustomKeyStoreResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetKeyResult.Companion.toKotlin as getKeyResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetPublicKeyResult.Companion.toKotlin as getPublicKeyResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetSecretResult.Companion.toKotlin as getSecretResultToKotlin
import com.pulumi.aws.kms.kotlin.outputs.GetSecretsResult.Companion.toKotlin as getSecretsResultToKotlin
public object KmsFunctions {
/**
* Use this data source to get the ARN of a KMS key alias.
* By using this data source, you can reference key alias
* without having to hard code the ARN as input.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const s3 = aws.kms.getAlias({
* name: "alias/aws/s3",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* s3 = aws.kms.get_alias(name="alias/aws/s3")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var s3 = Aws.Kms.GetAlias.Invoke(new()
* {
* Name = "alias/aws/s3",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.LookupAlias(ctx, &kms.LookupAliasArgs{
* Name: "alias/aws/s3",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetAliasArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var s3 = KmsFunctions.getAlias(GetAliasArgs.builder()
* .name("alias/aws/s3")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* s3:
* fn::invoke:
* Function: aws:kms:getAlias
* Arguments:
* name: alias/aws/s3
* ```
*
* @param argument A collection of arguments for invoking getAlias.
* @return A collection of values returned by getAlias.
*/
public suspend fun getAlias(argument: GetAliasPlainArgs): GetAliasResult =
getAliasResultToKotlin(getAliasPlain(argument.toJava()).await())
/**
* @see [getAlias].
* @param name Display name of the alias. The name must start with the word "alias" followed by a forward slash (alias/)
* @return A collection of values returned by getAlias.
*/
public suspend fun getAlias(name: String): GetAliasResult {
val argument = GetAliasPlainArgs(
name = name,
)
return getAliasResultToKotlin(getAliasPlain(argument.toJava()).await())
}
/**
* @see [getAlias].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetAliasPlainArgs].
* @return A collection of values returned by getAlias.
*/
public suspend fun getAlias(argument: suspend GetAliasPlainArgsBuilder.() -> Unit): GetAliasResult {
val builder = GetAliasPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAliasResultToKotlin(getAliasPlain(builtArgument.toJava()).await())
}
/**
* The KMS ciphertext data source allows you to encrypt plaintext into ciphertext
* by using an AWS KMS customer master key. The value returned by this data source
* changes every apply. For a stable ciphertext value, see the `aws.kms.Ciphertext`
* resource.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const oauthConfig = new aws.kms.Key("oauth_config", {
* description: "oauth config",
* isEnabled: true,
* });
* const oauth = aws.kms.getCipherTextOutput({
* keyId: oauthConfig.keyId,
* plaintext: `{
* "client_id": "e587dbae22222f55da22",
* "client_secret": "8289575d00000ace55e1815ec13673955721b8a5"
* }
* `,
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* oauth_config = aws.kms.Key("oauth_config",
* description="oauth config",
* is_enabled=True)
* oauth = aws.kms.get_cipher_text_output(key_id=oauth_config.key_id,
* plaintext="""{
* "client_id": "e587dbae22222f55da22",
* "client_secret": "8289575d00000ace55e1815ec13673955721b8a5"
* }
* """)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var oauthConfig = new Aws.Kms.Key("oauth_config", new()
* {
* Description = "oauth config",
* IsEnabled = true,
* });
* var oauth = Aws.Kms.GetCipherText.Invoke(new()
* {
* KeyId = oauthConfig.KeyId,
* Plaintext = @"{
* ""client_id"": ""e587dbae22222f55da22"",
* ""client_secret"": ""8289575d00000ace55e1815ec13673955721b8a5""
* }
* ",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* oauthConfig, err := kms.NewKey(ctx, "oauth_config", &kms.KeyArgs{
* Description: pulumi.String("oauth config"),
* IsEnabled: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _ = kms.GetCipherTextOutput(ctx, kms.GetCipherTextOutputArgs{
* KeyId: oauthConfig.KeyId,
* Plaintext: pulumi.String("{\n \"client_id\": \"e587dbae22222f55da22\",\n \"client_secret\": \"8289575d00000ace55e1815ec13673955721b8a5\"\n}\n"),
* }, nil)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.Key;
* import com.pulumi.aws.kms.KeyArgs;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetCipherTextArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var oauthConfig = new Key("oauthConfig", KeyArgs.builder()
* .description("oauth config")
* .isEnabled(true)
* .build());
* final var oauth = KmsFunctions.getCipherText(GetCipherTextArgs.builder()
* .keyId(oauthConfig.keyId())
* .plaintext("""
* {
* "client_id": "e587dbae22222f55da22",
* "client_secret": "8289575d00000ace55e1815ec13673955721b8a5"
* }
* """)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* oauthConfig:
* type: aws:kms:Key
* name: oauth_config
* properties:
* description: oauth config
* isEnabled: true
* variables:
* oauth:
* fn::invoke:
* Function: aws:kms:getCipherText
* Arguments:
* keyId: ${oauthConfig.keyId}
* plaintext: |
* {
* "client_id": "e587dbae22222f55da22",
* "client_secret": "8289575d00000ace55e1815ec13673955721b8a5"
* }
* ```
*
* @param argument A collection of arguments for invoking getCipherText.
* @return A collection of values returned by getCipherText.
*/
public suspend fun getCipherText(argument: GetCipherTextPlainArgs): GetCipherTextResult =
getCipherTextResultToKotlin(getCipherTextPlain(argument.toJava()).await())
/**
* @see [getCipherText].
* @param context An optional mapping that makes up the encryption context.
* @param keyId Globally unique key ID for the customer master key.
* @param plaintext Data to be encrypted. Note that this may show up in logs, and it will be stored in the state file.
* @return A collection of values returned by getCipherText.
*/
public suspend fun getCipherText(
context: Map? = null,
keyId: String,
plaintext: String,
): GetCipherTextResult {
val argument = GetCipherTextPlainArgs(
context = context,
keyId = keyId,
plaintext = plaintext,
)
return getCipherTextResultToKotlin(getCipherTextPlain(argument.toJava()).await())
}
/**
* @see [getCipherText].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetCipherTextPlainArgs].
* @return A collection of values returned by getCipherText.
*/
public suspend fun getCipherText(argument: suspend GetCipherTextPlainArgsBuilder.() -> Unit): GetCipherTextResult {
val builder = GetCipherTextPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCipherTextResultToKotlin(getCipherTextPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to get the metadata KMS custom key store.
* By using this data source, you can reference KMS custom key store
* without having to hard code the ID as input.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const keystore = aws.kms.getCustomKeyStore({
* customKeyStoreName: "my_cloudhsm",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* keystore = aws.kms.get_custom_key_store(custom_key_store_name="my_cloudhsm")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var keystore = Aws.Kms.GetCustomKeyStore.Invoke(new()
* {
* CustomKeyStoreName = "my_cloudhsm",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.LookupCustomKeyStore(ctx, &kms.LookupCustomKeyStoreArgs{
* CustomKeyStoreName: pulumi.StringRef("my_cloudhsm"),
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetCustomKeyStoreArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var keystore = KmsFunctions.getCustomKeyStore(GetCustomKeyStoreArgs.builder()
* .customKeyStoreName("my_cloudhsm")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* keystore:
* fn::invoke:
* Function: aws:kms:getCustomKeyStore
* Arguments:
* customKeyStoreName: my_cloudhsm
* ```
*
* @param argument A collection of arguments for invoking getCustomKeyStore.
* @return A collection of values returned by getCustomKeyStore.
*/
public suspend fun getCustomKeyStore(argument: GetCustomKeyStorePlainArgs): GetCustomKeyStoreResult =
getCustomKeyStoreResultToKotlin(getCustomKeyStorePlain(argument.toJava()).await())
/**
* @see [getCustomKeyStore].
* @param customKeyStoreId The ID for the custom key store.
* @param customKeyStoreName The user-specified friendly name for the custom key store.
* @return A collection of values returned by getCustomKeyStore.
*/
public suspend fun getCustomKeyStore(
customKeyStoreId: String? = null,
customKeyStoreName: String? =
null,
): GetCustomKeyStoreResult {
val argument = GetCustomKeyStorePlainArgs(
customKeyStoreId = customKeyStoreId,
customKeyStoreName = customKeyStoreName,
)
return getCustomKeyStoreResultToKotlin(getCustomKeyStorePlain(argument.toJava()).await())
}
/**
* @see [getCustomKeyStore].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetCustomKeyStorePlainArgs].
* @return A collection of values returned by getCustomKeyStore.
*/
public suspend fun getCustomKeyStore(argument: suspend GetCustomKeyStorePlainArgsBuilder.() -> Unit): GetCustomKeyStoreResult {
val builder = GetCustomKeyStorePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCustomKeyStoreResultToKotlin(getCustomKeyStorePlain(builtArgument.toJava()).await())
}
/**
* Use this data source to get detailed information about
* the specified KMS Key with flexible key id input.
* This can be useful to reference key alias
* without having to hard code the ARN as input.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const byAlias = aws.kms.getKey({
* keyId: "alias/my-key",
* });
* const byId = aws.kms.getKey({
* keyId: "1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* const byAliasArn = aws.kms.getKey({
* keyId: "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* });
* const byKeyArn = aws.kms.getKey({
* keyId: "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* by_alias = aws.kms.get_key(key_id="alias/my-key")
* by_id = aws.kms.get_key(key_id="1234abcd-12ab-34cd-56ef-1234567890ab")
* by_alias_arn = aws.kms.get_key(key_id="arn:aws:kms:us-east-1:111122223333:alias/my-key")
* by_key_arn = aws.kms.get_key(key_id="arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var byAlias = Aws.Kms.GetKey.Invoke(new()
* {
* KeyId = "alias/my-key",
* });
* var byId = Aws.Kms.GetKey.Invoke(new()
* {
* KeyId = "1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* var byAliasArn = Aws.Kms.GetKey.Invoke(new()
* {
* KeyId = "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* });
* var byKeyArn = Aws.Kms.GetKey.Invoke(new()
* {
* KeyId = "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.LookupKey(ctx, &kms.LookupKeyArgs{
* KeyId: "alias/my-key",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.LookupKey(ctx, &kms.LookupKeyArgs{
* KeyId: "1234abcd-12ab-34cd-56ef-1234567890ab",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.LookupKey(ctx, &kms.LookupKeyArgs{
* KeyId: "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.LookupKey(ctx, &kms.LookupKeyArgs{
* KeyId: "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var byAlias = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("alias/my-key")
* .build());
* final var byId = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("1234abcd-12ab-34cd-56ef-1234567890ab")
* .build());
* final var byAliasArn = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("arn:aws:kms:us-east-1:111122223333:alias/my-key")
* .build());
* final var byKeyArn = KmsFunctions.getKey(GetKeyArgs.builder()
* .keyId("arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* byAlias:
* fn::invoke:
* Function: aws:kms:getKey
* Arguments:
* keyId: alias/my-key
* byId:
* fn::invoke:
* Function: aws:kms:getKey
* Arguments:
* keyId: 1234abcd-12ab-34cd-56ef-1234567890ab
* byAliasArn:
* fn::invoke:
* Function: aws:kms:getKey
* Arguments:
* keyId: arn:aws:kms:us-east-1:111122223333:alias/my-key
* byKeyArn:
* fn::invoke:
* Function: aws:kms:getKey
* Arguments:
* keyId: arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* ```
*
* @param argument A collection of arguments for invoking getKey.
* @return A collection of values returned by getKey.
*/
public suspend fun getKey(argument: GetKeyPlainArgs): GetKeyResult =
getKeyResultToKotlin(getKeyPlain(argument.toJava()).await())
/**
* @see [getKey].
* @param grantTokens List of grant tokens
* @param keyId Key identifier which can be one of the following format:
* * Key ID. E.g: `1234abcd-12ab-34cd-56ef-1234567890ab`
* * Key ARN. E.g.: `arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab`
* * Alias name. E.g.: `alias/my-key`
* * Alias ARN: E.g.: `arn:aws:kms:us-east-1:111122223333:alias/my-key`
* @return A collection of values returned by getKey.
*/
public suspend fun getKey(grantTokens: List? = null, keyId: String): GetKeyResult {
val argument = GetKeyPlainArgs(
grantTokens = grantTokens,
keyId = keyId,
)
return getKeyResultToKotlin(getKeyPlain(argument.toJava()).await())
}
/**
* @see [getKey].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetKeyPlainArgs].
* @return A collection of values returned by getKey.
*/
public suspend fun getKey(argument: suspend GetKeyPlainArgsBuilder.() -> Unit): GetKeyResult {
val builder = GetKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKeyResultToKotlin(getKeyPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to get the public key about the specified KMS Key with flexible key id input. This can be useful to reference key alias without having to hard code the ARN as input.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const byAlias = aws.kms.getPublicKey({
* keyId: "alias/my-key",
* });
* const byId = aws.kms.getPublicKey({
* keyId: "1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* const byAliasArn = aws.kms.getPublicKey({
* keyId: "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* });
* const byKeyArn = aws.kms.getPublicKey({
* keyId: "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* by_alias = aws.kms.get_public_key(key_id="alias/my-key")
* by_id = aws.kms.get_public_key(key_id="1234abcd-12ab-34cd-56ef-1234567890ab")
* by_alias_arn = aws.kms.get_public_key(key_id="arn:aws:kms:us-east-1:111122223333:alias/my-key")
* by_key_arn = aws.kms.get_public_key(key_id="arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var byAlias = Aws.Kms.GetPublicKey.Invoke(new()
* {
* KeyId = "alias/my-key",
* });
* var byId = Aws.Kms.GetPublicKey.Invoke(new()
* {
* KeyId = "1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* var byAliasArn = Aws.Kms.GetPublicKey.Invoke(new()
* {
* KeyId = "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* });
* var byKeyArn = Aws.Kms.GetPublicKey.Invoke(new()
* {
* KeyId = "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.GetPublicKey(ctx, &kms.GetPublicKeyArgs{
* KeyId: "alias/my-key",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.GetPublicKey(ctx, &kms.GetPublicKeyArgs{
* KeyId: "1234abcd-12ab-34cd-56ef-1234567890ab",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.GetPublicKey(ctx, &kms.GetPublicKeyArgs{
* KeyId: "arn:aws:kms:us-east-1:111122223333:alias/my-key",
* }, nil)
* if err != nil {
* return err
* }
* _, err = kms.GetPublicKey(ctx, &kms.GetPublicKeyArgs{
* KeyId: "arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.KmsFunctions;
* import com.pulumi.aws.kms.inputs.GetPublicKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var byAlias = KmsFunctions.getPublicKey(GetPublicKeyArgs.builder()
* .keyId("alias/my-key")
* .build());
* final var byId = KmsFunctions.getPublicKey(GetPublicKeyArgs.builder()
* .keyId("1234abcd-12ab-34cd-56ef-1234567890ab")
* .build());
* final var byAliasArn = KmsFunctions.getPublicKey(GetPublicKeyArgs.builder()
* .keyId("arn:aws:kms:us-east-1:111122223333:alias/my-key")
* .build());
* final var byKeyArn = KmsFunctions.getPublicKey(GetPublicKeyArgs.builder()
* .keyId("arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* byAlias:
* fn::invoke:
* Function: aws:kms:getPublicKey
* Arguments:
* keyId: alias/my-key
* byId:
* fn::invoke:
* Function: aws:kms:getPublicKey
* Arguments:
* keyId: 1234abcd-12ab-34cd-56ef-1234567890ab
* byAliasArn:
* fn::invoke:
* Function: aws:kms:getPublicKey
* Arguments:
* keyId: arn:aws:kms:us-east-1:111122223333:alias/my-key
* byKeyArn:
* fn::invoke:
* Function: aws:kms:getPublicKey
* Arguments:
* keyId: arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
* ```
*
* @param argument A collection of arguments for invoking getPublicKey.
* @return A collection of values returned by getPublicKey.
*/
public suspend fun getPublicKey(argument: GetPublicKeyPlainArgs): GetPublicKeyResult =
getPublicKeyResultToKotlin(getPublicKeyPlain(argument.toJava()).await())
/**
* @see [getPublicKey].
* @param grantTokens List of grant tokens
* @param keyId Key identifier which can be one of the following format:
* * Key ID. E.g - `1234abcd-12ab-34cd-56ef-1234567890ab`
* * Key ARN. E.g. - `arn:aws:kms:us-east-1:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab`
* * Alias name. E.g. - `alias/my-key`
* * Alias ARN - E.g. - `arn:aws:kms:us-east-1:111122223333:alias/my-key`
* @return A collection of values returned by getPublicKey.
*/
public suspend fun getPublicKey(grantTokens: List? = null, keyId: String): GetPublicKeyResult {
val argument = GetPublicKeyPlainArgs(
grantTokens = grantTokens,
keyId = keyId,
)
return getPublicKeyResultToKotlin(getPublicKeyPlain(argument.toJava()).await())
}
/**
* @see [getPublicKey].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetPublicKeyPlainArgs].
* @return A collection of values returned by getPublicKey.
*/
public suspend fun getPublicKey(argument: suspend GetPublicKeyPlainArgsBuilder.() -> Unit): GetPublicKeyResult {
val builder = GetPublicKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPublicKeyResultToKotlin(getPublicKeyPlain(builtArgument.toJava()).await())
}
/**
*
* @param argument A collection of arguments for invoking getSecret.
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: GetSecretPlainArgs): GetSecretResult =
getSecretResultToKotlin(getSecretPlain(argument.toJava()).await())
/**
* @see [getSecret].
* @param secrets
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(secrets: List): GetSecretResult {
val argument = GetSecretPlainArgs(
secrets = secrets,
)
return getSecretResultToKotlin(getSecretPlain(argument.toJava()).await())
}
/**
* @see [getSecret].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetSecretPlainArgs].
* @return A collection of values returned by getSecret.
*/
public suspend fun getSecret(argument: suspend GetSecretPlainArgsBuilder.() -> Unit): GetSecretResult {
val builder = GetSecretPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretResultToKotlin(getSecretPlain(builtArgument.toJava()).await())
}
/**
* Decrypt multiple secrets from data encrypted with the AWS KMS service.
* ## Example Usage
* If you do not already have a `CiphertextBlob` from encrypting a KMS secret, you can use the below commands to obtain one using the [AWS CLI kms encrypt](https://docs.aws.amazon.com/cli/latest/reference/kms/encrypt.html) command. This requires you to have your AWS CLI setup correctly and replace the `--key-id` with your own. Alternatively you can use `--plaintext 'master-password'` (CLIv1) or `--plaintext fileb://<(echo -n 'master-password')` (CLIv2) instead of reading from a file.
* > If you have a newline character at the end of your file, it will be decrypted with this newline character intact. For most use cases this is undesirable and leads to incorrect passwords or invalid values, as well as possible changes in the plan. Be sure to use `echo -n` if necessary.
* > If you are using asymmetric keys ensure you are using the right encryption algorithm when you encrypt and decrypt else you will get IncorrectKeyException during the decrypt phase.
* ```console
* % echo -n 'master-password' > plaintext-password
* % aws kms encrypt --key-id ab123456-c012-4567-890a-deadbeef123 --plaintext fileb://plaintext-password --encryption-context foo=bar --output text --query CiphertextBlob
* AQECAHgaPa0J8WadplGCqqVAr4HNvDaFSQ+NaiwIBhmm6qDSFwAAAGIwYAYJKoZIhvcNAQcGoFMwUQIBADBMBgkqhkiG9w0BBwEwHgYJYIZIAWUDBAEuMBEEDI+LoLdvYv8l41OhAAIBEIAfx49FFJCLeYrkfMfAw6XlnxP23MmDBdqP8dPp28OoAQ==
* % aws kms encrypt --key-id ab123456-c012-4567-890a-deadbeef123 --plaintext fileb://plaintext-password --encryption-algorithm RSAES_OAEP_SHA_256 --output text --query CiphertextBlob
* AQECAHgaPa0J8WadplGCqqVAr4HNvDaFSQ+NaiwIBhmm6qDSFwAAAGIwYAYJKoZIhvcNAQcGoFMwUQIBADBMBgkqhkiG9w0BBwEwHgYJYIZIAWUDBAEuMBEEDI+LoLdvYv8l41OhAAIBEIAfx49FFJCLeYrkfMfAw6XlnxP23MmDBdqP8dPp28OoAQ==
* ```
* That encrypted output can now be inserted into provider configurations without exposing the plaintext secret directly.
* @param argument A collection of arguments for invoking getSecrets.
* @return A collection of values returned by getSecrets.
*/
public suspend fun getSecrets(argument: GetSecretsPlainArgs): GetSecretsResult =
getSecretsResultToKotlin(getSecretsPlain(argument.toJava()).await())
/**
* @see [getSecrets].
* @param secrets One or more encrypted payload definitions from the KMS service. See the Secret Definitions below.
* @return A collection of values returned by getSecrets.
*/
public suspend fun getSecrets(secrets: List): GetSecretsResult {
val argument = GetSecretsPlainArgs(
secrets = secrets,
)
return getSecretsResultToKotlin(getSecretsPlain(argument.toJava()).await())
}
/**
* @see [getSecrets].
* @param argument Builder for [com.pulumi.aws.kms.kotlin.inputs.GetSecretsPlainArgs].
* @return A collection of values returned by getSecrets.
*/
public suspend fun getSecrets(argument: suspend GetSecretsPlainArgsBuilder.() -> Unit): GetSecretsResult {
val builder = GetSecretsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getSecretsResultToKotlin(getSecretsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy