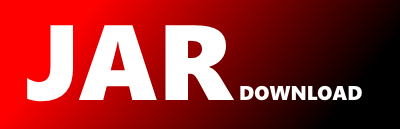
com.pulumi.aws.kms.kotlin.ReplicaExternalKey.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kms.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [ReplicaExternalKey].
*/
@PulumiTagMarker
public class ReplicaExternalKeyResourceBuilder internal constructor() {
public var name: String? = null
public var args: ReplicaExternalKeyArgs = ReplicaExternalKeyArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ReplicaExternalKeyArgsBuilder.() -> Unit) {
val builder = ReplicaExternalKeyArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ReplicaExternalKey {
val builtJavaResource = com.pulumi.aws.kms.ReplicaExternalKey(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ReplicaExternalKey(builtJavaResource)
}
}
/**
* Manages a KMS multi-Region replica key that uses external key material.
* See the [AWS KMS Developer Guide](https://docs.aws.amazon.com/kms/latest/developerguide/multi-region-keys-import.html) for more information on importing key material into multi-Region keys.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const primary = new aws.kms.ExternalKey("primary", {
* description: "Multi-Region primary key",
* deletionWindowInDays: 30,
* multiRegion: true,
* enabled: true,
* keyMaterialBase64: "...",
* });
* const replica = new aws.kms.ReplicaExternalKey("replica", {
* description: "Multi-Region replica key",
* deletionWindowInDays: 7,
* primaryKeyArn: primaryAwsKmsExternal.arn,
* keyMaterialBase64: "...",
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* primary = aws.kms.ExternalKey("primary",
* description="Multi-Region primary key",
* deletion_window_in_days=30,
* multi_region=True,
* enabled=True,
* key_material_base64="...")
* replica = aws.kms.ReplicaExternalKey("replica",
* description="Multi-Region replica key",
* deletion_window_in_days=7,
* primary_key_arn=primary_aws_kms_external["arn"],
* key_material_base64="...")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var primary = new Aws.Kms.ExternalKey("primary", new()
* {
* Description = "Multi-Region primary key",
* DeletionWindowInDays = 30,
* MultiRegion = true,
* Enabled = true,
* KeyMaterialBase64 = "...",
* });
* var replica = new Aws.Kms.ReplicaExternalKey("replica", new()
* {
* Description = "Multi-Region replica key",
* DeletionWindowInDays = 7,
* PrimaryKeyArn = primaryAwsKmsExternal.Arn,
* KeyMaterialBase64 = "...",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/kms"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := kms.NewExternalKey(ctx, "primary", &kms.ExternalKeyArgs{
* Description: pulumi.String("Multi-Region primary key"),
* DeletionWindowInDays: pulumi.Int(30),
* MultiRegion: pulumi.Bool(true),
* Enabled: pulumi.Bool(true),
* KeyMaterialBase64: pulumi.String("..."),
* })
* if err != nil {
* return err
* }
* _, err = kms.NewReplicaExternalKey(ctx, "replica", &kms.ReplicaExternalKeyArgs{
* Description: pulumi.String("Multi-Region replica key"),
* DeletionWindowInDays: pulumi.Int(7),
* PrimaryKeyArn: pulumi.Any(primaryAwsKmsExternal.Arn),
* KeyMaterialBase64: pulumi.String("..."),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.kms.ExternalKey;
* import com.pulumi.aws.kms.ExternalKeyArgs;
* import com.pulumi.aws.kms.ReplicaExternalKey;
* import com.pulumi.aws.kms.ReplicaExternalKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var primary = new ExternalKey("primary", ExternalKeyArgs.builder()
* .description("Multi-Region primary key")
* .deletionWindowInDays(30)
* .multiRegion(true)
* .enabled(true)
* .keyMaterialBase64("...")
* .build());
* var replica = new ReplicaExternalKey("replica", ReplicaExternalKeyArgs.builder()
* .description("Multi-Region replica key")
* .deletionWindowInDays(7)
* .primaryKeyArn(primaryAwsKmsExternal.arn())
* .keyMaterialBase64("...")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* primary:
* type: aws:kms:ExternalKey
* properties:
* description: Multi-Region primary key
* deletionWindowInDays: 30
* multiRegion: true
* enabled: true
* keyMaterialBase64: '...'
* replica:
* type: aws:kms:ReplicaExternalKey
* properties:
* description: Multi-Region replica key
* deletionWindowInDays: 7
* primaryKeyArn: ${primaryAwsKmsExternal.arn}
* keyMaterialBase64: '...'
* ```
*
* ## Import
* Using `pulumi import`, import KMS multi-Region replica keys using the `id`. For example:
* ```sh
* $ pulumi import aws:kms/replicaExternalKey:ReplicaExternalKey example 1234abcd-12ab-34cd-56ef-1234567890ab
* ```
*/
public class ReplicaExternalKey internal constructor(
override val javaResource: com.pulumi.aws.kms.ReplicaExternalKey,
) : KotlinCustomResource(javaResource, ReplicaExternalKeyMapper) {
/**
* The Amazon Resource Name (ARN) of the replica key. The key ARNs of related multi-Region keys differ only in the Region value.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* A flag to indicate whether to bypass the key policy lockout safety check.
* Setting this value to true increases the risk that the KMS key becomes unmanageable. Do not set this value to true indiscriminately.
* For more information, refer to the scenario in the [Default Key Policy](https://docs.aws.amazon.com/kms/latest/developerguide/key-policies.html#key-policy-default-allow-root-enable-iam) section in the _AWS Key Management Service Developer Guide_.
* The default value is `false`.
*/
public val bypassPolicyLockoutSafetyCheck: Output?
get() = javaResource.bypassPolicyLockoutSafetyCheck().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The waiting period, specified in number of days. After the waiting period ends, AWS KMS deletes the KMS key.
* If you specify a value, it must be between `7` and `30`, inclusive. If you do not specify a value, it defaults to `30`.
*/
public val deletionWindowInDays: Output?
get() = javaResource.deletionWindowInDays().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A description of the KMS key.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Specifies whether the replica key is enabled. Disabled KMS keys cannot be used in cryptographic operations. Keys pending import can only be `false`. Imported keys default to `true` unless expired.
*/
public val enabled: Output
get() = javaResource.enabled().applyValue({ args0 -> args0 })
/**
* Whether the key material expires. Empty when pending key material import, otherwise `KEY_MATERIAL_EXPIRES` or `KEY_MATERIAL_DOES_NOT_EXPIRE`.
*/
public val expirationModel: Output
get() = javaResource.expirationModel().applyValue({ args0 -> args0 })
/**
* The key ID of the replica key. Related multi-Region keys have the same key ID.
*/
public val keyId: Output
get() = javaResource.keyId().applyValue({ args0 -> args0 })
/**
* Base64 encoded 256-bit symmetric encryption key material to import. The KMS key is permanently associated with this key material. The same key material can be [reimported](https://docs.aws.amazon.com/kms/latest/developerguide/importing-keys.html#reimport-key-material), but you cannot import different key material.
*/
public val keyMaterialBase64: Output?
get() = javaResource.keyMaterialBase64().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The state of the replica key.
*/
public val keyState: Output
get() = javaResource.keyState().applyValue({ args0 -> args0 })
/**
* The [cryptographic operations](https://docs.aws.amazon.com/kms/latest/developerguide/concepts.html#cryptographic-operations) for which you can use the KMS key. This is a shared property of multi-Region keys.
*/
public val keyUsage: Output
get() = javaResource.keyUsage().applyValue({ args0 -> args0 })
/**
* The key policy to attach to the KMS key. If you do not specify a key policy, AWS KMS attaches the [default key policy](https://docs.aws.amazon.com/kms/latest/developerguide/key-policies.html#key-policy-default) to the KMS key.
*/
public val policy: Output
get() = javaResource.policy().applyValue({ args0 -> args0 })
/**
* The ARN of the multi-Region primary key to replicate. The primary key must be in a different AWS Region of the same AWS Partition. You can create only one replica of a given primary key in each AWS Region.
*/
public val primaryKeyArn: Output
get() = javaResource.primaryKeyArn().applyValue({ args0 -> args0 })
/**
* A map of tags to assign to the replica key. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy