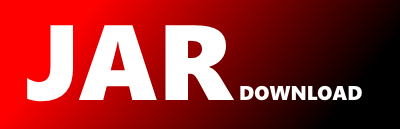
com.pulumi.aws.kotlin.inputs.GetServicePlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.kotlin.inputs
import com.pulumi.aws.inputs.GetServicePlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getService.
* @property dnsName DNS name of the service (_e.g.,_ `rds.us-east-1.amazonaws.com`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
* @property id
* @property region Region of the service (_e.g.,_ `us-west-2`, `ap-northeast-1`).
* @property reverseDnsName Reverse DNS name of the service (_e.g.,_ `com.amazonaws.us-west-2.s3`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
* @property reverseDnsPrefix Prefix of the service (_e.g.,_ `com.amazonaws` in AWS Commercial, `cn.com.amazonaws` in AWS China).
* @property serviceId Service endpoint ID (_e.g.,_ `s3`, `rds`, `ec2`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required. A service's endpoint ID can be found in the [_AWS General Reference_](https://docs.aws.amazon.com/general/latest/gr/aws-service-information.html).
*/
public data class GetServicePlainArgs(
public val dnsName: String? = null,
public val id: String? = null,
public val region: String? = null,
public val reverseDnsName: String? = null,
public val reverseDnsPrefix: String? = null,
public val serviceId: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.inputs.GetServicePlainArgs =
com.pulumi.aws.inputs.GetServicePlainArgs.builder()
.dnsName(dnsName?.let({ args0 -> args0 }))
.id(id?.let({ args0 -> args0 }))
.region(region?.let({ args0 -> args0 }))
.reverseDnsName(reverseDnsName?.let({ args0 -> args0 }))
.reverseDnsPrefix(reverseDnsPrefix?.let({ args0 -> args0 }))
.serviceId(serviceId?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetServicePlainArgs].
*/
@PulumiTagMarker
public class GetServicePlainArgsBuilder internal constructor() {
private var dnsName: String? = null
private var id: String? = null
private var region: String? = null
private var reverseDnsName: String? = null
private var reverseDnsPrefix: String? = null
private var serviceId: String? = null
/**
* @param value DNS name of the service (_e.g.,_ `rds.us-east-1.amazonaws.com`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*/
@JvmName("lnsnmlgkexjpgvvk")
public suspend fun dnsName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.dnsName = mapped
}
/**
* @param value
*/
@JvmName("cjkmhoyeebqshluw")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.id = mapped
}
/**
* @param value Region of the service (_e.g.,_ `us-west-2`, `ap-northeast-1`).
*/
@JvmName("ycrdqndbdtwfarbu")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.region = mapped
}
/**
* @param value Reverse DNS name of the service (_e.g.,_ `com.amazonaws.us-west-2.s3`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required.
*/
@JvmName("vwrfvnxkxsqcsyyh")
public suspend fun reverseDnsName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.reverseDnsName = mapped
}
/**
* @param value Prefix of the service (_e.g.,_ `com.amazonaws` in AWS Commercial, `cn.com.amazonaws` in AWS China).
*/
@JvmName("hhxhkvorticxelbn")
public suspend fun reverseDnsPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.reverseDnsPrefix = mapped
}
/**
* @param value Service endpoint ID (_e.g.,_ `s3`, `rds`, `ec2`). One of `dns_name`, `reverse_dns_name`, or `service_id` is required. A service's endpoint ID can be found in the [_AWS General Reference_](https://docs.aws.amazon.com/general/latest/gr/aws-service-information.html).
*/
@JvmName("gpwcbsskvjfhgblf")
public suspend fun serviceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.serviceId = mapped
}
internal fun build(): GetServicePlainArgs = GetServicePlainArgs(
dnsName = dnsName,
id = id,
region = region,
reverseDnsName = reverseDnsName,
reverseDnsPrefix = reverseDnsPrefix,
serviceId = serviceId,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy