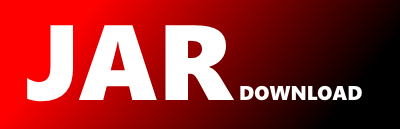
com.pulumi.aws.lakeformation.kotlin.inputs.GetPermissionsPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lakeformation.kotlin.inputs
import com.pulumi.aws.lakeformation.inputs.GetPermissionsPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getPermissions.
* @property catalogId Identifier for the Data Catalog. By default, the account ID. The Data Catalog is the persistent metadata store. It contains database definitions, table definitions, and other control information to manage your Lake Formation environment.
* @property catalogResource Whether the permissions are to be granted for the Data Catalog. Defaults to `false`.
* @property dataCellsFilter Configuration block for a data cells filter resource. Detailed below.
* @property dataLocation Configuration block for a data location resource. Detailed below.
* @property database Configuration block for a database resource. Detailed below.
* @property lfTag Configuration block for an LF-tag resource. Detailed below.
* @property lfTagPolicy Configuration block for an LF-tag policy resource. Detailed below.
* @property principal Principal to be granted the permissions on the resource. Supported principals are IAM users or IAM roles.
* One of the following is required:
* @property table Configuration block for a table resource. Detailed below.
* @property tableWithColumns Configuration block for a table with columns resource. Detailed below.
* The following arguments are optional:
*/
public data class GetPermissionsPlainArgs(
public val catalogId: String? = null,
public val catalogResource: Boolean? = null,
public val dataCellsFilter: GetPermissionsDataCellsFilter? = null,
public val dataLocation: GetPermissionsDataLocation? = null,
public val database: GetPermissionsDatabase? = null,
public val lfTag: GetPermissionsLfTag? = null,
public val lfTagPolicy: GetPermissionsLfTagPolicy? = null,
public val principal: String,
public val table: GetPermissionsTable? = null,
public val tableWithColumns: GetPermissionsTableWithColumns? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lakeformation.inputs.GetPermissionsPlainArgs =
com.pulumi.aws.lakeformation.inputs.GetPermissionsPlainArgs.builder()
.catalogId(catalogId?.let({ args0 -> args0 }))
.catalogResource(catalogResource?.let({ args0 -> args0 }))
.dataCellsFilter(dataCellsFilter?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dataLocation(dataLocation?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.database(database?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.lfTag(lfTag?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.lfTagPolicy(lfTagPolicy?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.principal(principal.let({ args0 -> args0 }))
.table(table?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tableWithColumns(tableWithColumns?.let({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [GetPermissionsPlainArgs].
*/
@PulumiTagMarker
public class GetPermissionsPlainArgsBuilder internal constructor() {
private var catalogId: String? = null
private var catalogResource: Boolean? = null
private var dataCellsFilter: GetPermissionsDataCellsFilter? = null
private var dataLocation: GetPermissionsDataLocation? = null
private var database: GetPermissionsDatabase? = null
private var lfTag: GetPermissionsLfTag? = null
private var lfTagPolicy: GetPermissionsLfTagPolicy? = null
private var principal: String? = null
private var table: GetPermissionsTable? = null
private var tableWithColumns: GetPermissionsTableWithColumns? = null
/**
* @param value Identifier for the Data Catalog. By default, the account ID. The Data Catalog is the persistent metadata store. It contains database definitions, table definitions, and other control information to manage your Lake Formation environment.
*/
@JvmName("itcldlppsmwvclmw")
public suspend fun catalogId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.catalogId = mapped
}
/**
* @param value Whether the permissions are to be granted for the Data Catalog. Defaults to `false`.
*/
@JvmName("qjrjrqyfmxifhcje")
public suspend fun catalogResource(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.catalogResource = mapped
}
/**
* @param value Configuration block for a data cells filter resource. Detailed below.
*/
@JvmName("rpyklugaxgevxujf")
public suspend fun dataCellsFilter(`value`: GetPermissionsDataCellsFilter?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.dataCellsFilter = mapped
}
/**
* @param argument Configuration block for a data cells filter resource. Detailed below.
*/
@JvmName("rpnffodobmdthyqc")
public suspend fun dataCellsFilter(argument: suspend GetPermissionsDataCellsFilterBuilder.() -> Unit) {
val toBeMapped = GetPermissionsDataCellsFilterBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.dataCellsFilter = mapped
}
/**
* @param value Configuration block for a data location resource. Detailed below.
*/
@JvmName("mjaneteeiwgtiasi")
public suspend fun dataLocation(`value`: GetPermissionsDataLocation?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.dataLocation = mapped
}
/**
* @param argument Configuration block for a data location resource. Detailed below.
*/
@JvmName("qxssbffxwhsueyfm")
public suspend fun dataLocation(argument: suspend GetPermissionsDataLocationBuilder.() -> Unit) {
val toBeMapped = GetPermissionsDataLocationBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.dataLocation = mapped
}
/**
* @param value Configuration block for a database resource. Detailed below.
*/
@JvmName("xmtfomslivgcvxwt")
public suspend fun database(`value`: GetPermissionsDatabase?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.database = mapped
}
/**
* @param argument Configuration block for a database resource. Detailed below.
*/
@JvmName("hpygaapaiiuueqim")
public suspend fun database(argument: suspend GetPermissionsDatabaseBuilder.() -> Unit) {
val toBeMapped = GetPermissionsDatabaseBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.database = mapped
}
/**
* @param value Configuration block for an LF-tag resource. Detailed below.
*/
@JvmName("ryoqtinwcclkbtcp")
public suspend fun lfTag(`value`: GetPermissionsLfTag?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.lfTag = mapped
}
/**
* @param argument Configuration block for an LF-tag resource. Detailed below.
*/
@JvmName("rgrqrbpubjdpfect")
public suspend fun lfTag(argument: suspend GetPermissionsLfTagBuilder.() -> Unit) {
val toBeMapped = GetPermissionsLfTagBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.lfTag = mapped
}
/**
* @param value Configuration block for an LF-tag policy resource. Detailed below.
*/
@JvmName("edaewcjtswycbdnd")
public suspend fun lfTagPolicy(`value`: GetPermissionsLfTagPolicy?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.lfTagPolicy = mapped
}
/**
* @param argument Configuration block for an LF-tag policy resource. Detailed below.
*/
@JvmName("vdojcihqwmqennml")
public suspend fun lfTagPolicy(argument: suspend GetPermissionsLfTagPolicyBuilder.() -> Unit) {
val toBeMapped = GetPermissionsLfTagPolicyBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.lfTagPolicy = mapped
}
/**
* @param value Principal to be granted the permissions on the resource. Supported principals are IAM users or IAM roles.
* One of the following is required:
*/
@JvmName("lrlxqoaajdyjlkgw")
public suspend fun principal(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> args0 })
this.principal = mapped
}
/**
* @param value Configuration block for a table resource. Detailed below.
*/
@JvmName("oqrvcoksnywemhji")
public suspend fun table(`value`: GetPermissionsTable?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.table = mapped
}
/**
* @param argument Configuration block for a table resource. Detailed below.
*/
@JvmName("fflsjigieeossako")
public suspend fun table(argument: suspend GetPermissionsTableBuilder.() -> Unit) {
val toBeMapped = GetPermissionsTableBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.table = mapped
}
/**
* @param value Configuration block for a table with columns resource. Detailed below.
* The following arguments are optional:
*/
@JvmName("ddnbuomaqgwwymen")
public suspend fun tableWithColumns(`value`: GetPermissionsTableWithColumns?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.tableWithColumns = mapped
}
/**
* @param argument Configuration block for a table with columns resource. Detailed below.
* The following arguments are optional:
*/
@JvmName("gkvkdebcrwnyswld")
public suspend fun tableWithColumns(argument: suspend GetPermissionsTableWithColumnsBuilder.() -> Unit) {
val toBeMapped = GetPermissionsTableWithColumnsBuilder().applySuspend { argument() }.build()
val mapped = toBeMapped
this.tableWithColumns = mapped
}
internal fun build(): GetPermissionsPlainArgs = GetPermissionsPlainArgs(
catalogId = catalogId,
catalogResource = catalogResource,
dataCellsFilter = dataCellsFilter,
dataLocation = dataLocation,
database = database,
lfTag = lfTag,
lfTagPolicy = lfTagPolicy,
principal = principal ?: throw PulumiNullFieldException("principal"),
table = table,
tableWithColumns = tableWithColumns,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy