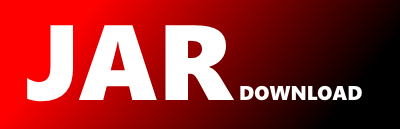
com.pulumi.aws.lambda.kotlin.InvocationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lambda.kotlin
import com.pulumi.aws.lambda.InvocationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Use this resource to invoke a lambda function. The lambda function is invoked with the [RequestResponse](https://docs.aws.amazon.com/lambda/latest/dg/API_Invoke.html#API_Invoke_RequestSyntax) invocation type.
* > **NOTE:** By default this resource _only_ invokes the function when the arguments call for a create or replace. In other words, after an initial invocation on _apply_, if the arguments do not change, a subsequent _apply_ does not invoke the function again. To dynamically invoke the function, see the `triggers` example below. To always invoke a function on each _apply_, see the `aws.lambda.Invocation` data source. To invoke the lambda function when the Pulumi resource is updated and deleted, see the CRUD Lifecycle Scope example below.
* > **NOTE:** If you get a `KMSAccessDeniedException: Lambda was unable to decrypt the environment variables because KMS access was denied` error when invoking an `aws.lambda.Function` with environment variables, the IAM role associated with the function may have been deleted and recreated _after_ the function was created. You can fix the problem two ways: 1) updating the function's role to another role and then updating it back again to the recreated role, or 2) by using Pulumi to `taint` the function and `apply` your configuration again to recreate the function. (When you create a function, Lambda grants permissions on the KMS key to the function's IAM role. If the IAM role is recreated, the grant is no longer valid. Changing the function's role or recreating the function causes Lambda to update the grant.)
* ## Example Usage
* ### Dynamic Invocation Example Using Triggers
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* import * as std from "@pulumi/std";
* const example = new aws.lambda.Invocation("example", {
* functionName: lambdaFunctionTest.functionName,
* triggers: {
* redeployment: std.sha1({
* input: JSON.stringify([exampleAwsLambdaFunction.environment]),
* }).then(invoke => invoke.result),
* },
* input: JSON.stringify({
* key1: "value1",
* key2: "value2",
* }),
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* import pulumi_std as std
* example = aws.lambda_.Invocation("example",
* function_name=lambda_function_test["functionName"],
* triggers={
* "redeployment": std.sha1(input=json.dumps([example_aws_lambda_function["environment"]])).result,
* },
* input=json.dumps({
* "key1": "value1",
* "key2": "value2",
* }))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Lambda.Invocation("example", new()
* {
* FunctionName = lambdaFunctionTest.FunctionName,
* Triggers =
* {
* { "redeployment", Std.Sha1.Invoke(new()
* {
* Input = JsonSerializer.Serialize(new[]
* {
* exampleAwsLambdaFunction.Environment,
* }),
* }).Apply(invoke => invoke.Result) },
* },
* Input = JsonSerializer.Serialize(new Dictionary
* {
* ["key1"] = "value1",
* ["key2"] = "value2",
* }),
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lambda"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal([]interface{}{
* exampleAwsLambdaFunction.Environment,
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* invokeSha1, err := std.Sha1(ctx, &std.Sha1Args{
* Input: json0,
* }, nil)
* if err != nil {
* return err
* }
* tmpJSON1, err := json.Marshal(map[string]interface{}{
* "key1": "value1",
* "key2": "value2",
* })
* if err != nil {
* return err
* }
* json1 := string(tmpJSON1)
* _, err = lambda.NewInvocation(ctx, "example", &lambda.InvocationArgs{
* FunctionName: pulumi.Any(lambdaFunctionTest.FunctionName),
* Triggers: pulumi.StringMap{
* "redeployment": pulumi.String(invokeSha1.Result),
* },
* Input: pulumi.String(json1),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Invocation;
* import com.pulumi.aws.lambda.InvocationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Invocation("example", InvocationArgs.builder()
* .functionName(lambdaFunctionTest.functionName())
* .triggers(Map.of("redeployment", StdFunctions.sha1(Sha1Args.builder()
* .input(serializeJson(
* jsonArray(exampleAwsLambdaFunction.environment())))
* .build()).result()))
* .input(serializeJson(
* jsonObject(
* jsonProperty("key1", "value1"),
* jsonProperty("key2", "value2")
* )))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:lambda:Invocation
* properties:
* functionName: ${lambdaFunctionTest.functionName}
* triggers:
* redeployment:
* fn::invoke:
* Function: std:sha1
* Arguments:
* input:
* fn::toJSON:
* - ${exampleAwsLambdaFunction.environment}
* Return: result
* input:
* fn::toJSON:
* key1: value1
* key2: value2
* ```
*
* ### CRUD Lifecycle Scope
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.lambda.Invocation("example", {
* functionName: lambdaFunctionTest.functionName,
* input: JSON.stringify({
* key1: "value1",
* key2: "value2",
* }),
* lifecycleScope: "CRUD",
* });
* ```
* ```python
* import pulumi
* import json
* import pulumi_aws as aws
* example = aws.lambda_.Invocation("example",
* function_name=lambda_function_test["functionName"],
* input=json.dumps({
* "key1": "value1",
* "key2": "value2",
* }),
* lifecycle_scope="CRUD")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using System.Text.Json;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Lambda.Invocation("example", new()
* {
* FunctionName = lambdaFunctionTest.FunctionName,
* Input = JsonSerializer.Serialize(new Dictionary
* {
* ["key1"] = "value1",
* ["key2"] = "value2",
* }),
* LifecycleScope = "CRUD",
* });
* });
* ```
* ```go
* package main
* import (
* "encoding/json"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lambda"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* tmpJSON0, err := json.Marshal(map[string]interface{}{
* "key1": "value1",
* "key2": "value2",
* })
* if err != nil {
* return err
* }
* json0 := string(tmpJSON0)
* _, err = lambda.NewInvocation(ctx, "example", &lambda.InvocationArgs{
* FunctionName: pulumi.Any(lambdaFunctionTest.FunctionName),
* Input: pulumi.String(json0),
* LifecycleScope: pulumi.String("CRUD"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lambda.Invocation;
* import com.pulumi.aws.lambda.InvocationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Invocation("example", InvocationArgs.builder()
* .functionName(lambdaFunctionTest.functionName())
* .input(serializeJson(
* jsonObject(
* jsonProperty("key1", "value1"),
* jsonProperty("key2", "value2")
* )))
* .lifecycleScope("CRUD")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:lambda:Invocation
* properties:
* functionName: ${lambdaFunctionTest.functionName}
* input:
* fn::toJSON:
* key1: value1
* key2: value2
* lifecycleScope: CRUD
* ```
*
* > **NOTE:** `lifecycle_scope = "CRUD"` will inject a key `tf` in the input event to pass lifecycle information! This allows the lambda function to handle different lifecycle transitions uniquely. If you need to use a key `tf` in your own input JSON, the default key name can be overridden with the `pulumi_key` argument.
* The key `tf` gets added with subkeys:
* * `action` - Action Pulumi performs on the resource. Values are `create`, `update`, or `delete`.
* * `prev_input` - Input JSON payload from the previous invocation. This can be used to handle update and delete events.
* When the resource from the example above is created, the Lambda will get following JSON payload:
* ```json
* {
* "key1": "value1",
* "key2": "value2",
* "tf": {
* "action": "create",
* "prev_input": null
* }
* }
* ```
* If the input value of `key1` changes to "valueB", then the lambda will be invoked again with the following JSON payload:
* ```json
* {
* "key1": "valueB",
* "key2": "value2",
* "tf": {
* "action": "update",
* "prev_input": {
* "key1": "value1",
* "key2": "value2"
* }
* }
* }
* ```
* When the invocation resource is removed, the final invocation will have the following JSON payload:
* ```json
* {
* "key1": "valueB",
* "key2": "value2",
* "tf": {
* "action": "delete",
* "prev_input": {
* "key1": "valueB",
* "key2": "value2"
* }
* }
* }
* ```
* @property functionName Name of the lambda function.
* @property input JSON payload to the lambda function.
* The following arguments are optional:
* @property lifecycleScope Lifecycle scope of the resource to manage. Valid values are `CREATE_ONLY` and `CRUD`. Defaults to `CREATE_ONLY`. `CREATE_ONLY` will invoke the function only on creation or replacement. `CRUD` will invoke the function on each lifecycle event, and augment the input JSON payload with additional lifecycle information.
* @property qualifier Qualifier (i.e., version) of the lambda function. Defaults to `$LATEST`.
* @property terraformKey
* @property triggers Map of arbitrary keys and values that, when changed, will trigger a re-invocation.
*/
public data class InvocationArgs(
public val functionName: Output? = null,
public val input: Output? = null,
public val lifecycleScope: Output? = null,
public val qualifier: Output? = null,
public val terraformKey: Output? = null,
public val triggers: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy