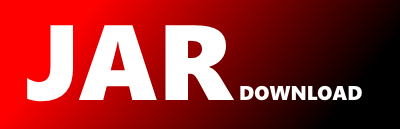
com.pulumi.aws.lex.kotlin.inputs.BotAliasConversationLogsLogSettingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.BotAliasConversationLogsLogSettingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property destination The destination where logs are delivered. Options are `CLOUDWATCH_LOGS` or `S3`.
* @property kmsKeyArn The Amazon Resource Name (ARN) of the key used to encrypt audio logs in an S3 bucket. This can only be specified when `destination` is set to `S3`. Must be between 20 and 2048 characters in length.
* @property logType The type of logging that is enabled. Options are `AUDIO` or `TEXT`.
* @property resourceArn The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs are delivered. Must be less than or equal to 2048 characters in length.
* @property resourcePrefix The prefix of the S3 object key for `AUDIO` logs or the log stream name for `TEXT` logs.
*/
public data class BotAliasConversationLogsLogSettingArgs(
public val destination: Output,
public val kmsKeyArn: Output? = null,
public val logType: Output,
public val resourceArn: Output,
public val resourcePrefix: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.BotAliasConversationLogsLogSettingArgs =
com.pulumi.aws.lex.inputs.BotAliasConversationLogsLogSettingArgs.builder()
.destination(destination.applyValue({ args0 -> args0 }))
.kmsKeyArn(kmsKeyArn?.applyValue({ args0 -> args0 }))
.logType(logType.applyValue({ args0 -> args0 }))
.resourceArn(resourceArn.applyValue({ args0 -> args0 }))
.resourcePrefix(resourcePrefix?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BotAliasConversationLogsLogSettingArgs].
*/
@PulumiTagMarker
public class BotAliasConversationLogsLogSettingArgsBuilder internal constructor() {
private var destination: Output? = null
private var kmsKeyArn: Output? = null
private var logType: Output? = null
private var resourceArn: Output? = null
private var resourcePrefix: Output? = null
/**
* @param value The destination where logs are delivered. Options are `CLOUDWATCH_LOGS` or `S3`.
*/
@JvmName("rdlvtswhrplnqgte")
public suspend fun destination(`value`: Output) {
this.destination = value
}
/**
* @param value The Amazon Resource Name (ARN) of the key used to encrypt audio logs in an S3 bucket. This can only be specified when `destination` is set to `S3`. Must be between 20 and 2048 characters in length.
*/
@JvmName("ouxgnudcnwrnrmot")
public suspend fun kmsKeyArn(`value`: Output) {
this.kmsKeyArn = value
}
/**
* @param value The type of logging that is enabled. Options are `AUDIO` or `TEXT`.
*/
@JvmName("ujippmennnxesxjo")
public suspend fun logType(`value`: Output) {
this.logType = value
}
/**
* @param value The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs are delivered. Must be less than or equal to 2048 characters in length.
*/
@JvmName("jbdoptopwslxsrfi")
public suspend fun resourceArn(`value`: Output) {
this.resourceArn = value
}
/**
* @param value The prefix of the S3 object key for `AUDIO` logs or the log stream name for `TEXT` logs.
*/
@JvmName("diythdwjrarsefyh")
public suspend fun resourcePrefix(`value`: Output) {
this.resourcePrefix = value
}
/**
* @param value The destination where logs are delivered. Options are `CLOUDWATCH_LOGS` or `S3`.
*/
@JvmName("pscyhpwvdexpjydr")
public suspend fun destination(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.destination = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the key used to encrypt audio logs in an S3 bucket. This can only be specified when `destination` is set to `S3`. Must be between 20 and 2048 characters in length.
*/
@JvmName("knfejrvqkuwmgqfa")
public suspend fun kmsKeyArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsKeyArn = mapped
}
/**
* @param value The type of logging that is enabled. Options are `AUDIO` or `TEXT`.
*/
@JvmName("ggwsabcdvnoqrxdm")
public suspend fun logType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.logType = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the CloudWatch Logs log group or S3 bucket where the logs are delivered. Must be less than or equal to 2048 characters in length.
*/
@JvmName("qanlrlvwvyouthcw")
public suspend fun resourceArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.resourceArn = mapped
}
/**
* @param value The prefix of the S3 object key for `AUDIO` logs or the log stream name for `TEXT` logs.
*/
@JvmName("jlvbwtdbmqksmjbi")
public suspend fun resourcePrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePrefix = mapped
}
internal fun build(): BotAliasConversationLogsLogSettingArgs =
BotAliasConversationLogsLogSettingArgs(
destination = destination ?: throw PulumiNullFieldException("destination"),
kmsKeyArn = kmsKeyArn,
logType = logType ?: throw PulumiNullFieldException("logType"),
resourceArn = resourceArn ?: throw PulumiNullFieldException("resourceArn"),
resourcePrefix = resourcePrefix,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy