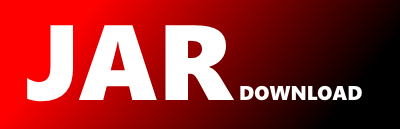
com.pulumi.aws.lex.kotlin.inputs.V2modelsBotMemberArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsBotMemberArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property aliasId (Required) - Alias ID of a bot that is a member of this network of bots.
* @property aliasName (Required) - Alias name of a bot that is a member of this network of bots.
* @property id (Required) - Unique ID of a bot that is a member of this network of bots.
* @property name Name of the bot. The bot name must be unique in the account that creates the bot. Type String. Length Constraints: Minimum length of 1. Maximum length of 100.
* @property version (Required) - Version of a bot that is a member of this network of bots.
*/
public data class V2modelsBotMemberArgs(
public val aliasId: Output,
public val aliasName: Output,
public val id: Output,
public val name: Output,
public val version: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsBotMemberArgs =
com.pulumi.aws.lex.inputs.V2modelsBotMemberArgs.builder()
.aliasId(aliasId.applyValue({ args0 -> args0 }))
.aliasName(aliasName.applyValue({ args0 -> args0 }))
.id(id.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.version(version.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [V2modelsBotMemberArgs].
*/
@PulumiTagMarker
public class V2modelsBotMemberArgsBuilder internal constructor() {
private var aliasId: Output? = null
private var aliasName: Output? = null
private var id: Output? = null
private var name: Output? = null
private var version: Output? = null
/**
* @param value (Required) - Alias ID of a bot that is a member of this network of bots.
*/
@JvmName("lhxcdfggagohrylh")
public suspend fun aliasId(`value`: Output) {
this.aliasId = value
}
/**
* @param value (Required) - Alias name of a bot that is a member of this network of bots.
*/
@JvmName("dlrweyqhjsxfoxdn")
public suspend fun aliasName(`value`: Output) {
this.aliasName = value
}
/**
* @param value (Required) - Unique ID of a bot that is a member of this network of bots.
*/
@JvmName("asasexqsxkneacsc")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Name of the bot. The bot name must be unique in the account that creates the bot. Type String. Length Constraints: Minimum length of 1. Maximum length of 100.
*/
@JvmName("gniorohxujwtncqp")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value (Required) - Version of a bot that is a member of this network of bots.
*/
@JvmName("kxuhjwhiuqnspldu")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value (Required) - Alias ID of a bot that is a member of this network of bots.
*/
@JvmName("dgbkkwdxyebugajv")
public suspend fun aliasId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.aliasId = mapped
}
/**
* @param value (Required) - Alias name of a bot that is a member of this network of bots.
*/
@JvmName("jcgcjermvnqjqbnc")
public suspend fun aliasName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.aliasName = mapped
}
/**
* @param value (Required) - Unique ID of a bot that is a member of this network of bots.
*/
@JvmName("evmpyyfofjabqvbm")
public suspend fun id(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Name of the bot. The bot name must be unique in the account that creates the bot. Type String. Length Constraints: Minimum length of 1. Maximum length of 100.
*/
@JvmName("qqvgjnkykslebnjr")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value (Required) - Version of a bot that is a member of this network of bots.
*/
@JvmName("ieohkmwcccjvkctk")
public suspend fun version(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): V2modelsBotMemberArgs = V2modelsBotMemberArgs(
aliasId = aliasId ?: throw PulumiNullFieldException("aliasId"),
aliasName = aliasName ?: throw PulumiNullFieldException("aliasName"),
id = id ?: throw PulumiNullFieldException("id"),
name = name ?: throw PulumiNullFieldException("name"),
version = version ?: throw PulumiNullFieldException("version"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy