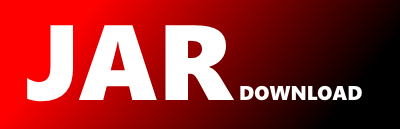
com.pulumi.aws.lex.kotlin.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property customPayload Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
* @property imageResponseCard Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
* @property plainTextMessage Configuration block for a message in plain text format. See `plain_text_message`.
* @property ssmlMessage Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
public data class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs(
public val customPayload: Output? =
null,
public val imageResponseCard: Output? =
null,
public val plainTextMessage: Output? =
null,
public val ssmlMessage: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs =
com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs.builder()
.customPayload(customPayload?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.imageResponseCard(imageResponseCard?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.plainTextMessage(plainTextMessage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ssmlMessage(ssmlMessage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs].
*/
@PulumiTagMarker
public class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgsBuilder
internal constructor() {
private var customPayload:
Output? =
null
private var imageResponseCard:
Output? =
null
private var plainTextMessage:
Output? =
null
private var ssmlMessage:
Output? =
null
/**
* @param value Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("fmcqcwhsoluntanw")
public suspend fun customPayload(`value`: Output) {
this.customPayload = value
}
/**
* @param value Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("dimdrjiqbgygnigw")
public suspend fun imageResponseCard(`value`: Output) {
this.imageResponseCard = value
}
/**
* @param value Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("mgkjxxiibosvjovt")
public suspend fun plainTextMessage(`value`: Output) {
this.plainTextMessage = value
}
/**
* @param value Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("jtxwcnwvxpnjvxmk")
public suspend fun ssmlMessage(`value`: Output) {
this.ssmlMessage = value
}
/**
* @param value Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("jjqehdeaslqsvbdw")
public suspend fun customPayload(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationCustomPayloadArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customPayload = mapped
}
/**
* @param argument Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("ssaaifgvmwkmpwvm")
public suspend fun customPayload(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationCustomPayloadArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationCustomPayloadArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customPayload = mapped
}
/**
* @param value Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("hpcvtbimdpwxhefq")
public suspend fun imageResponseCard(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationImageResponseCardArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageResponseCard = mapped
}
/**
* @param argument Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("uwxjfwkcvyfhflhb")
public suspend fun imageResponseCard(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationImageResponseCardArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationImageResponseCardArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.imageResponseCard = mapped
}
/**
* @param value Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("sxwsxsnveipqtvit")
public suspend fun plainTextMessage(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationPlainTextMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.plainTextMessage = mapped
}
/**
* @param argument Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("okgpgnxsfjtjldej")
public suspend fun plainTextMessage(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationPlainTextMessageArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationPlainTextMessageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.plainTextMessage = mapped
}
/**
* @param value Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("jjmigitaqcfuamoq")
public suspend fun ssmlMessage(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationSsmlMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ssmlMessage = mapped
}
/**
* @param argument Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("hwxctesgmspvuqdu")
public suspend fun ssmlMessage(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationSsmlMessageArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationSsmlMessageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ssmlMessage = mapped
}
internal fun build(): V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureConditionalDefaultBranchResponseMessageGroupVariationArgs(
customPayload = customPayload,
imageResponseCard = imageResponseCard,
plainTextMessage = plainTextMessage,
ssmlMessage = ssmlMessage,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy