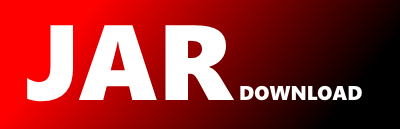
com.pulumi.aws.lex.kotlin.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property customPayload Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
* @property imageResponseCard Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
* @property plainTextMessage Configuration block for a message in plain text format. See `plain_text_message`.
* @property ssmlMessage Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
public data class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs(
public val customPayload: Output? =
null,
public val imageResponseCard: Output? =
null,
public val plainTextMessage: Output? =
null,
public val ssmlMessage: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs =
com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs.builder()
.customPayload(customPayload?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.imageResponseCard(imageResponseCard?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.plainTextMessage(plainTextMessage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ssmlMessage(ssmlMessage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs].
*/
@PulumiTagMarker
public class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgsBuilder
internal constructor() {
private var customPayload:
Output? =
null
private var imageResponseCard:
Output? =
null
private var plainTextMessage:
Output? =
null
private var ssmlMessage:
Output? =
null
/**
* @param value Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("iyrwynhqrprkahcc")
public suspend fun customPayload(`value`: Output) {
this.customPayload = value
}
/**
* @param value Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("ppijnrudtdcjtgia")
public suspend fun imageResponseCard(`value`: Output) {
this.imageResponseCard = value
}
/**
* @param value Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("yagmnufwpfvqkkxv")
public suspend fun plainTextMessage(`value`: Output) {
this.plainTextMessage = value
}
/**
* @param value Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("wwqitgmwjohwvrpo")
public suspend fun ssmlMessage(`value`: Output) {
this.ssmlMessage = value
}
/**
* @param value Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("frvpvsjoocrntkty")
public suspend fun customPayload(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationCustomPayloadArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customPayload = mapped
}
/**
* @param argument Configuration block for a message in a custom format defined by the client application. See `custom_payload`.
*/
@JvmName("hxcdtwvgngjojfdj")
public suspend fun customPayload(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationCustomPayloadArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationCustomPayloadArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.customPayload = mapped
}
/**
* @param value Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("nvdovdhogdrmdmyg")
public suspend fun imageResponseCard(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationImageResponseCardArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageResponseCard = mapped
}
/**
* @param argument Configuration block for a message that defines a response card that the client application can show to the user. See `image_response_card`.
*/
@JvmName("meorpxlwatmkepei")
public suspend fun imageResponseCard(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationImageResponseCardArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationImageResponseCardArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.imageResponseCard = mapped
}
/**
* @param value Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("wyrwrpbfppoofmys")
public suspend fun plainTextMessage(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationPlainTextMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.plainTextMessage = mapped
}
/**
* @param argument Configuration block for a message in plain text format. See `plain_text_message`.
*/
@JvmName("gelrxlogocypokbm")
public suspend fun plainTextMessage(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationPlainTextMessageArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationPlainTextMessageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.plainTextMessage = mapped
}
/**
* @param value Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("uqwewraqfmaevfmo")
public suspend fun ssmlMessage(`value`: V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationSsmlMessageArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ssmlMessage = mapped
}
/**
* @param argument Configuration block for a message in Speech Synthesis Markup Language (SSML). See `ssml_message`.
*/
@JvmName("pbxplwaoxpggdlby")
public suspend fun ssmlMessage(argument: suspend V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationSsmlMessageArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationSsmlMessageArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.ssmlMessage = mapped
}
internal fun build(): V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs =
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationFailureResponseMessageGroupVariationArgs(
customPayload = customPayload,
imageResponseCard = imageResponseCard,
plainTextMessage = plainTextMessage,
ssmlMessage = ssmlMessage,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy