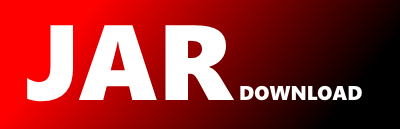
com.pulumi.aws.lex.kotlin.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property buttons Configuration blocks for buttons that should be displayed on the response card. The arrangement of the buttons is determined by the platform that displays the button. See `button`.
* @property imageUrl URL of an image to display on the response card. The image URL must be publicly available so that the platform displaying the response card has access to the image.
* @property subtitle Subtitle to display on the response card. The format of the subtitle is determined by the platform displaying the response card.
* @property title Title to display on the response card. The format of the title is determined by the platform displaying the response card.
*/
public data class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs(
public val buttons: Output>? =
null,
public val imageUrl: Output? = null,
public val subtitle: Output? = null,
public val title: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs =
com.pulumi.aws.lex.inputs.V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs.builder()
.buttons(
buttons?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.imageUrl(imageUrl?.applyValue({ args0 -> args0 }))
.subtitle(subtitle?.applyValue({ args0 -> args0 }))
.title(title.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgs].
*/
@PulumiTagMarker
public class
V2modelsIntentConfirmationSettingCodeHookPostCodeHookSpecificationTimeoutConditionalConditionalBranchResponseMessageGroupVariationImageResponseCardArgsBuilder
internal constructor() {
private var buttons:
Output>? =
null
private var imageUrl: Output? = null
private var subtitle: Output? = null
private var title: Output? = null
/**
* @param value Configuration blocks for buttons that should be displayed on the response card. The arrangement of the buttons is determined by the platform that displays the button. See `button`.
*/
@JvmName("xokmgybajjyulpme")
public suspend fun buttons(`value`: Output>) {
this.buttons = value
}
@JvmName("cjvcfttryfaahvnb")
public suspend fun buttons(vararg values: Output) {
this.buttons = Output.all(values.asList())
}
/**
* @param values Configuration blocks for buttons that should be displayed on the response card. The arrangement of the buttons is determined by the platform that displays the button. See `button`.
*/
@JvmName("ennybqnesalroakg")
public suspend fun buttons(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy