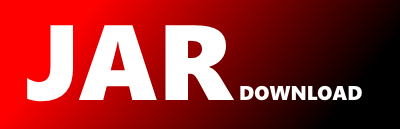
com.pulumi.aws.lex.kotlin.inputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property failureConditional Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
* @property failureNextStep Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
* @property failureResponse Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
* @property successConditional Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
* @property successNextStep Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
* @property successResponse Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
* @property timeoutConditional Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
* @property timeoutNextStep Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
* @property timeoutResponse Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*/
public data class V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs(
public val failureConditional: Output? =
null,
public val failureNextStep: Output? =
null,
public val failureResponse: Output? =
null,
public val successConditional: Output? =
null,
public val successNextStep: Output? =
null,
public val successResponse: Output? =
null,
public val timeoutConditional: Output? =
null,
public val timeoutNextStep: Output? =
null,
public val timeoutResponse: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs =
com.pulumi.aws.lex.inputs.V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs.builder()
.failureConditional(
failureConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.failureNextStep(failureNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.failureResponse(failureResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.successConditional(
successConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.successNextStep(successNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.successResponse(successResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeoutConditional(
timeoutConditional?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.timeoutNextStep(timeoutNextStep?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeoutResponse(
timeoutResponse?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs].
*/
@PulumiTagMarker
public class V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgsBuilder internal constructor() {
private var failureConditional:
Output? =
null
private var failureNextStep:
Output? =
null
private var failureResponse:
Output? =
null
private var successConditional:
Output? =
null
private var successNextStep:
Output? =
null
private var successResponse:
Output? =
null
private var timeoutConditional:
Output? =
null
private var timeoutNextStep:
Output? =
null
private var timeoutResponse:
Output? =
null
/**
* @param value Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
*/
@JvmName("bfwnqtasxgfqffts")
public suspend fun failureConditional(`value`: Output) {
this.failureConditional = value
}
/**
* @param value Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
*/
@JvmName("hbfuyfrecfhqrleo")
public suspend fun failureNextStep(`value`: Output) {
this.failureNextStep = value
}
/**
* @param value Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
*/
@JvmName("uykkmsnbmnbpsngr")
public suspend fun failureResponse(`value`: Output) {
this.failureResponse = value
}
/**
* @param value Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
*/
@JvmName("rbygkbioebfpdjet")
public suspend fun successConditional(`value`: Output) {
this.successConditional = value
}
/**
* @param value Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
*/
@JvmName("nryckqgsfwwawdny")
public suspend fun successNextStep(`value`: Output) {
this.successNextStep = value
}
/**
* @param value Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
*/
@JvmName("lcifhbvrtqmkajnu")
public suspend fun successResponse(`value`: Output) {
this.successResponse = value
}
/**
* @param value Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
*/
@JvmName("wdvhyepnmsyyeehh")
public suspend fun timeoutConditional(`value`: Output) {
this.timeoutConditional = value
}
/**
* @param value Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
*/
@JvmName("lolrrvwgrgkuexgn")
public suspend fun timeoutNextStep(`value`: Output) {
this.timeoutNextStep = value
}
/**
* @param value Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*/
@JvmName("vyjehdjcduncedlx")
public suspend fun timeoutResponse(`value`: Output) {
this.timeoutResponse = value
}
/**
* @param value Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
*/
@JvmName("hrtuvkflhjijcojy")
public suspend fun failureConditional(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditionalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureConditional = mapped
}
/**
* @param argument Configuration block for conditional branches to evaluate after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_conditional`.
*/
@JvmName("thwqgxcwsscnpntx")
public suspend fun failureConditional(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditionalArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureConditionalArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.failureConditional = mapped
}
/**
* @param value Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
*/
@JvmName("emtwujmpygqdtvbj")
public suspend fun failureNextStep(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStepArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureNextStep = mapped
}
/**
* @param argument Configuration block for the next step the bot runs after the dialog code hook throws an exception or returns with the State field of the Intent object set to Failed. See `failure_next_step`.
*/
@JvmName("cydwxebavocfdbbk")
public suspend fun failureNextStep(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStepArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureNextStepArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.failureNextStep = mapped
}
/**
* @param value Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
*/
@JvmName("ahonjvneugbngyua")
public suspend fun failureResponse(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureResponse = mapped
}
/**
* @param argument Configuration block for message groups that Amazon Lex uses to respond the user input. See `failure_response`.
*/
@JvmName("tnijabnixsnnavov")
public suspend fun failureResponse(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponseArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationFailureResponseArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.failureResponse = mapped
}
/**
* @param value Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
*/
@JvmName("ncdxsftgwjbqskhe")
public suspend fun successConditional(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditionalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successConditional = mapped
}
/**
* @param argument Configuration block for conditional branches to evaluate after the dialog code hook finishes successfully. See `success_conditional`.
*/
@JvmName("qnravuwcktfraluh")
public suspend fun successConditional(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditionalArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessConditionalArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.successConditional = mapped
}
/**
* @param value Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
*/
@JvmName("yecyfdkxkyurwlea")
public suspend fun successNextStep(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStepArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successNextStep = mapped
}
/**
* @param argument Configuration block for the next step the bot runs after the dialog code hook finishes successfully. See `success_next_step`.
*/
@JvmName("lxscbalayddnoqqt")
public suspend fun successNextStep(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStepArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessNextStepArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.successNextStep = mapped
}
/**
* @param value Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
*/
@JvmName("hxsiunhefjmvtefh")
public suspend fun successResponse(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successResponse = mapped
}
/**
* @param argument Configuration block for message groups that Amazon Lex uses to respond the user input. See `success_response`.
*/
@JvmName("kajgwrgwlvritnmn")
public suspend fun successResponse(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponseArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationSuccessResponseArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.successResponse = mapped
}
/**
* @param value Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
*/
@JvmName("awsdfqounwvrnpcw")
public suspend fun timeoutConditional(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditionalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutConditional = mapped
}
/**
* @param argument Configuration block for conditional branches to evaluate if the code hook times out. See `timeout_conditional`.
*/
@JvmName("wbgakbqgibeuaaur")
public suspend fun timeoutConditional(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditionalArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutConditionalArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeoutConditional = mapped
}
/**
* @param value Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
*/
@JvmName("jufcxhxpguduistr")
public suspend fun timeoutNextStep(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStepArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutNextStep = mapped
}
/**
* @param argument Configuration block for the next step that the bot runs when the code hook times out. See `timeout_next_step`.
*/
@JvmName("vcnfdstkovlnrbbt")
public suspend fun timeoutNextStep(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStepArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutNextStepArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeoutNextStep = mapped
}
/**
* @param value Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*/
@JvmName("atyimaqeehnfvevy")
public suspend fun timeoutResponse(`value`: V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutResponse = mapped
}
/**
* @param argument Configuration block for a list of message groups that Amazon Lex uses to respond the user input. See `timeout_response`.
*/
@JvmName("iddyesjxrmyqliqf")
public suspend fun timeoutResponse(argument: suspend V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponseArgsBuilder.() -> Unit) {
val toBeMapped =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationTimeoutResponseArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timeoutResponse = mapped
}
internal fun build(): V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs =
V2modelsIntentFulfillmentCodeHookPostFulfillmentStatusSpecificationArgs(
failureConditional = failureConditional,
failureNextStep = failureNextStep,
failureResponse = failureResponse,
successConditional = successConditional,
successNextStep = successNextStep,
successResponse = successResponse,
timeoutConditional = timeoutConditional,
timeoutNextStep = timeoutNextStep,
timeoutResponse = timeoutResponse,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy