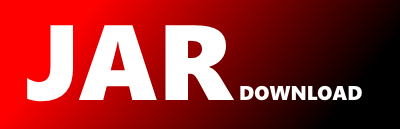
com.pulumi.aws.lex.kotlin.inputs.V2modelsSlotValueElicitationSettingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lex.kotlin.inputs
import com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property defaultValueSpecifications List of default values for a slot.
* See the `default_value_specification` argument reference below.
* @property promptSpecification Prompt that Amazon Lex uses to elicit the slot value from the user.
* See the `aws.lex.V2modelsIntent` resource for details on the `prompt_specification` argument reference - they are identical.
* @property sampleUtterances
* @property slotConstraint Whether the slot is required or optional. Valid values are `Required` or `Optional`.
* @property slotResolutionSettings Information about whether assisted slot resolution is turned on for the slot or not.
* See the `slot_resolution_setting` argument reference below.
* @property waitAndContinueSpecifications Specifies the prompts that Amazon Lex uses while a bot is waiting for customer input.
* See the `wait_and_continue_specification` argument reference below.
*/
public data class V2modelsSlotValueElicitationSettingArgs(
public val defaultValueSpecifications: Output>? = null,
public val promptSpecification: Output,
public val sampleUtterances: Output>? =
null,
public val slotConstraint: Output,
public val slotResolutionSettings: Output>? = null,
public val waitAndContinueSpecifications: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingArgs =
com.pulumi.aws.lex.inputs.V2modelsSlotValueElicitationSettingArgs.builder()
.defaultValueSpecifications(
defaultValueSpecifications?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.promptSpecification(
promptSpecification.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sampleUtterances(
sampleUtterances?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.slotConstraint(slotConstraint.applyValue({ args0 -> args0 }))
.slotResolutionSettings(
slotResolutionSettings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.waitAndContinueSpecifications(
waitAndContinueSpecifications?.applyValue({ args0 ->
args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
).build()
}
/**
* Builder for [V2modelsSlotValueElicitationSettingArgs].
*/
@PulumiTagMarker
public class V2modelsSlotValueElicitationSettingArgsBuilder internal constructor() {
private var defaultValueSpecifications:
Output>? = null
private var promptSpecification:
Output? = null
private var sampleUtterances:
Output>? = null
private var slotConstraint: Output? = null
private var slotResolutionSettings:
Output>? = null
private var waitAndContinueSpecifications:
Output>? = null
/**
* @param value List of default values for a slot.
* See the `default_value_specification` argument reference below.
*/
@JvmName("bhfujpetyiijvldf")
public suspend fun defaultValueSpecifications(`value`: Output>) {
this.defaultValueSpecifications = value
}
@JvmName("igdkhjohowvqsdow")
public suspend fun defaultValueSpecifications(vararg values: Output) {
this.defaultValueSpecifications = Output.all(values.asList())
}
/**
* @param values List of default values for a slot.
* See the `default_value_specification` argument reference below.
*/
@JvmName("lfsuwtlhuetxfjai")
public suspend fun defaultValueSpecifications(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy