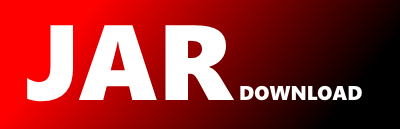
com.pulumi.aws.lightsail.kotlin.ContainerService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lightsail.kotlin
import com.pulumi.aws.lightsail.kotlin.outputs.ContainerServicePrivateRegistryAccess
import com.pulumi.aws.lightsail.kotlin.outputs.ContainerServicePublicDomainNames
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.aws.lightsail.kotlin.outputs.ContainerServicePrivateRegistryAccess.Companion.toKotlin as containerServicePrivateRegistryAccessToKotlin
import com.pulumi.aws.lightsail.kotlin.outputs.ContainerServicePublicDomainNames.Companion.toKotlin as containerServicePublicDomainNamesToKotlin
/**
* Builder for [ContainerService].
*/
@PulumiTagMarker
public class ContainerServiceResourceBuilder internal constructor() {
public var name: String? = null
public var args: ContainerServiceArgs = ContainerServiceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ContainerServiceArgsBuilder.() -> Unit) {
val builder = ContainerServiceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ContainerService {
val builtJavaResource = com.pulumi.aws.lightsail.ContainerService(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ContainerService(builtJavaResource)
}
}
/**
* An Amazon Lightsail container service is a highly scalable compute and networking resource on which you can deploy, run,
* and manage containers. For more information, see
* [Container services in Amazon Lightsail](https://lightsail.aws.amazon.com/ls/docs/en_us/articles/amazon-lightsail-container-services).
* > **Note:** For more information about the AWS Regions in which you can create Amazon Lightsail container services,
* see ["Regions and Availability Zones in Amazon Lightsail"](https://lightsail.aws.amazon.com/ls/docs/overview/article/understanding-regions-and-availability-zones-in-amazon-lightsail).
* ## Example Usage
* ### Basic Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const myContainerService = new aws.lightsail.ContainerService("my_container_service", {
* name: "container-service-1",
* power: "nano",
* scale: 1,
* isDisabled: false,
* tags: {
* foo1: "bar1",
* foo2: "",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* my_container_service = aws.lightsail.ContainerService("my_container_service",
* name="container-service-1",
* power="nano",
* scale=1,
* is_disabled=False,
* tags={
* "foo1": "bar1",
* "foo2": "",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var myContainerService = new Aws.LightSail.ContainerService("my_container_service", new()
* {
* Name = "container-service-1",
* Power = "nano",
* Scale = 1,
* IsDisabled = false,
* Tags =
* {
* { "foo1", "bar1" },
* { "foo2", "" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lightsail"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := lightsail.NewContainerService(ctx, "my_container_service", &lightsail.ContainerServiceArgs{
* Name: pulumi.String("container-service-1"),
* Power: pulumi.String("nano"),
* Scale: pulumi.Int(1),
* IsDisabled: pulumi.Bool(false),
* Tags: pulumi.StringMap{
* "foo1": pulumi.String("bar1"),
* "foo2": pulumi.String(""),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lightsail.ContainerService;
* import com.pulumi.aws.lightsail.ContainerServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myContainerService = new ContainerService("myContainerService", ContainerServiceArgs.builder()
* .name("container-service-1")
* .power("nano")
* .scale(1)
* .isDisabled(false)
* .tags(Map.ofEntries(
* Map.entry("foo1", "bar1"),
* Map.entry("foo2", "")
* ))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myContainerService:
* type: aws:lightsail:ContainerService
* name: my_container_service
* properties:
* name: container-service-1
* power: nano
* scale: 1
* isDisabled: false
* tags:
* foo1: bar1
* foo2:
* ```
*
* ### Public Domain Names
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const myContainerService = new aws.lightsail.ContainerService("my_container_service", {publicDomainNames: {
* certificates: [{
* certificateName: "example-certificate",
* domainNames: ["www.example.com"],
* }],
* }});
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* my_container_service = aws.lightsail.ContainerService("my_container_service", public_domain_names={
* "certificates": [{
* "certificate_name": "example-certificate",
* "domain_names": ["www.example.com"],
* }],
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var myContainerService = new Aws.LightSail.ContainerService("my_container_service", new()
* {
* PublicDomainNames = new Aws.LightSail.Inputs.ContainerServicePublicDomainNamesArgs
* {
* Certificates = new[]
* {
* new Aws.LightSail.Inputs.ContainerServicePublicDomainNamesCertificateArgs
* {
* CertificateName = "example-certificate",
* DomainNames = new[]
* {
* "www.example.com",
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lightsail"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := lightsail.NewContainerService(ctx, "my_container_service", &lightsail.ContainerServiceArgs{
* PublicDomainNames: &lightsail.ContainerServicePublicDomainNamesArgs{
* Certificates: lightsail.ContainerServicePublicDomainNamesCertificateArray{
* &lightsail.ContainerServicePublicDomainNamesCertificateArgs{
* CertificateName: pulumi.String("example-certificate"),
* DomainNames: pulumi.StringArray{
* pulumi.String("www.example.com"),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lightsail.ContainerService;
* import com.pulumi.aws.lightsail.ContainerServiceArgs;
* import com.pulumi.aws.lightsail.inputs.ContainerServicePublicDomainNamesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myContainerService = new ContainerService("myContainerService", ContainerServiceArgs.builder()
* .publicDomainNames(ContainerServicePublicDomainNamesArgs.builder()
* .certificates(ContainerServicePublicDomainNamesCertificateArgs.builder()
* .certificateName("example-certificate")
* .domainNames("www.example.com")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myContainerService:
* type: aws:lightsail:ContainerService
* name: my_container_service
* properties:
* publicDomainNames:
* certificates:
* - certificateName: example-certificate
* domainNames:
* - www.example.com
* ```
*
* ### Private Registry Access
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const defaultContainerService = new aws.lightsail.ContainerService("default", {privateRegistryAccess: {
* ecrImagePullerRole: {
* isActive: true,
* },
* }});
* const default = defaultContainerService.privateRegistryAccess.apply(privateRegistryAccess => aws.iam.getPolicyDocumentOutput({
* statements: [{
* effect: "Allow",
* principals: [{
* type: "AWS",
* identifiers: [privateRegistryAccess.ecrImagePullerRole?.principalArn],
* }],
* actions: [
* "ecr:BatchGetImage",
* "ecr:GetDownloadUrlForLayer",
* ],
* }],
* }));
* const defaultRepositoryPolicy = new aws.ecr.RepositoryPolicy("default", {
* repository: defaultAwsEcrRepository.name,
* policy: _default.apply(_default => _default.json),
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* default_container_service = aws.lightsail.ContainerService("default", private_registry_access={
* "ecr_image_puller_role": {
* "is_active": True,
* },
* })
* default = default_container_service.private_registry_access.apply(lambda private_registry_access: aws.iam.get_policy_document_output(statements=[{
* "effect": "Allow",
* "principals": [{
* "type": "AWS",
* "identifiers": [private_registry_access.ecr_image_puller_role.principal_arn],
* }],
* "actions": [
* "ecr:BatchGetImage",
* "ecr:GetDownloadUrlForLayer",
* ],
* }]))
* default_repository_policy = aws.ecr.RepositoryPolicy("default",
* repository=default_aws_ecr_repository["name"],
* policy=default.json)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var defaultContainerService = new Aws.LightSail.ContainerService("default", new()
* {
* PrivateRegistryAccess = new Aws.LightSail.Inputs.ContainerServicePrivateRegistryAccessArgs
* {
* EcrImagePullerRole = new Aws.LightSail.Inputs.ContainerServicePrivateRegistryAccessEcrImagePullerRoleArgs
* {
* IsActive = true,
* },
* },
* });
* var @default = Aws.Iam.GetPolicyDocument.Invoke(new()
* {
* Statements = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementInputArgs
* {
* Effect = "Allow",
* Principals = new[]
* {
* new Aws.Iam.Inputs.GetPolicyDocumentStatementPrincipalInputArgs
* {
* Type = "AWS",
* Identifiers = new[]
* {
* defaultContainerService.PrivateRegistryAccess.EcrImagePullerRole?.PrincipalArn,
* },
* },
* },
* Actions = new[]
* {
* "ecr:BatchGetImage",
* "ecr:GetDownloadUrlForLayer",
* },
* },
* },
* });
* var defaultRepositoryPolicy = new Aws.Ecr.RepositoryPolicy("default", new()
* {
* Repository = defaultAwsEcrRepository.Name,
* Policy = @default.Apply(@default => @default.Apply(getPolicyDocumentResult => getPolicyDocumentResult.Json)),
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/ecr"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/iam"
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/lightsail"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultContainerService, err := lightsail.NewContainerService(ctx, "default", &lightsail.ContainerServiceArgs{
* PrivateRegistryAccess: &lightsail.ContainerServicePrivateRegistryAccessArgs{
* EcrImagePullerRole: &lightsail.ContainerServicePrivateRegistryAccessEcrImagePullerRoleArgs{
* IsActive: pulumi.Bool(true),
* },
* },
* })
* if err != nil {
* return err
* }
* _default := defaultContainerService.PrivateRegistryAccess.ApplyT(func(privateRegistryAccess lightsail.ContainerServicePrivateRegistryAccess) (iam.GetPolicyDocumentResult, error) {
* return iam.GetPolicyDocumentResult(interface{}(iam.GetPolicyDocumentOutput(ctx, iam.GetPolicyDocumentOutputArgs{
* Statements: []iam.GetPolicyDocumentStatement{
* {
* Effect: "Allow",
* Principals: []iam.GetPolicyDocumentStatementPrincipal{
* {
* Type: "AWS",
* Identifiers: interface{}{
* privateRegistryAccess.EcrImagePullerRole.PrincipalArn,
* },
* },
* },
* Actions: []string{
* "ecr:BatchGetImage",
* "ecr:GetDownloadUrlForLayer",
* },
* },
* },
* }, nil))), nil
* }).(iam.GetPolicyDocumentResultOutput)
* _, err = ecr.NewRepositoryPolicy(ctx, "default", &ecr.RepositoryPolicyArgs{
* Repository: pulumi.Any(defaultAwsEcrRepository.Name),
* Policy: pulumi.String(_default.ApplyT(func(_default iam.GetPolicyDocumentResult) (*string, error) {
* return &default.Json, nil
* }).(pulumi.StringPtrOutput)),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.lightsail.ContainerService;
* import com.pulumi.aws.lightsail.ContainerServiceArgs;
* import com.pulumi.aws.lightsail.inputs.ContainerServicePrivateRegistryAccessArgs;
* import com.pulumi.aws.lightsail.inputs.ContainerServicePrivateRegistryAccessEcrImagePullerRoleArgs;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyDocumentArgs;
* import com.pulumi.aws.ecr.RepositoryPolicy;
* import com.pulumi.aws.ecr.RepositoryPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultContainerService = new ContainerService("defaultContainerService", ContainerServiceArgs.builder()
* .privateRegistryAccess(ContainerServicePrivateRegistryAccessArgs.builder()
* .ecrImagePullerRole(ContainerServicePrivateRegistryAccessEcrImagePullerRoleArgs.builder()
* .isActive(true)
* .build())
* .build())
* .build());
* final var default = IamFunctions.getPolicyDocument(GetPolicyDocumentArgs.builder()
* .statements(GetPolicyDocumentStatementArgs.builder()
* .effect("Allow")
* .principals(GetPolicyDocumentStatementPrincipalArgs.builder()
* .type("AWS")
* .identifiers(defaultContainerService.privateRegistryAccess().applyValue(privateRegistryAccess -> privateRegistryAccess.ecrImagePullerRole().principalArn()))
* .build())
* .actions(
* "ecr:BatchGetImage",
* "ecr:GetDownloadUrlForLayer")
* .build())
* .build());
* var defaultRepositoryPolicy = new RepositoryPolicy("defaultRepositoryPolicy", RepositoryPolicyArgs.builder()
* .repository(defaultAwsEcrRepository.name())
* .policy(default_.applyValue(default_ -> default_.json()))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* defaultContainerService:
* type: aws:lightsail:ContainerService
* name: default
* properties:
* privateRegistryAccess:
* ecrImagePullerRole:
* isActive: true
* defaultRepositoryPolicy:
* type: aws:ecr:RepositoryPolicy
* name: default
* properties:
* repository: ${defaultAwsEcrRepository.name}
* policy: ${default.json}
* variables:
* default:
* fn::invoke:
* Function: aws:iam:getPolicyDocument
* Arguments:
* statements:
* - effect: Allow
* principals:
* - type: AWS
* identifiers:
* - ${defaultContainerService.privateRegistryAccess.ecrImagePullerRole.principalArn}
* actions:
* - ecr:BatchGetImage
* - ecr:GetDownloadUrlForLayer
* ```
*
* ## Import
* Using `pulumi import`, import Lightsail Container Service using the `name`. For example:
* ```sh
* $ pulumi import aws:lightsail/containerService:ContainerService my_container_service container-service-1
* ```
*/
public class ContainerService internal constructor(
override val javaResource: com.pulumi.aws.lightsail.ContainerService,
) : KotlinCustomResource(javaResource, ContainerServiceMapper) {
/**
* The Amazon Resource Name (ARN) of the container service.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The Availability Zone. Follows the format us-east-2a (case-sensitive).
*/
public val availabilityZone: Output
get() = javaResource.availabilityZone().applyValue({ args0 -> args0 })
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* A Boolean value indicating whether the container service is disabled. Defaults to `false`.
*/
public val isDisabled: Output?
get() = javaResource.isDisabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name for the container service. Names must be of length 1 to 63, and be
* unique within each AWS Region in your Lightsail account.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The power specification for the container service. The power specifies the amount of memory,
* the number of vCPUs, and the monthly price of each node of the container service.
* Possible values: `nano`, `micro`, `small`, `medium`, `large`, `xlarge`.
*/
public val power: Output
get() = javaResource.power().applyValue({ args0 -> args0 })
/**
* The ID of the power of the container service.
*/
public val powerId: Output
get() = javaResource.powerId().applyValue({ args0 -> args0 })
/**
* The principal ARN of the container service. The principal ARN can be used to create a trust
* relationship between your standard AWS account and your Lightsail container service. This allows you to give your
* service permission to access resources in your standard AWS account.
*/
public val principalArn: Output
get() = javaResource.principalArn().applyValue({ args0 -> args0 })
/**
* The private domain name of the container service. The private domain name is accessible only
* by other resources within the default virtual private cloud (VPC) of your Lightsail account.
*/
public val privateDomainName: Output
get() = javaResource.privateDomainName().applyValue({ args0 -> args0 })
/**
* An object to describe the configuration for the container service to access private container image repositories, such as Amazon Elastic Container Registry (Amazon ECR) private repositories. See Private Registry Access below for more details.
*/
public val privateRegistryAccess: Output
get() = javaResource.privateRegistryAccess().applyValue({ args0 ->
args0.let({ args0 ->
containerServicePrivateRegistryAccessToKotlin(args0)
})
})
/**
* The public domain names to use with the container service, such as example.com
* and www.example.com. You can specify up to four public domain names for a container service. The domain names that you
* specify are used when you create a deployment with a container configured as the public endpoint of your container
* service. If you don't specify public domain names, then you can use the default domain of the container service.
* Defined below.
*/
public val publicDomainNames: Output?
get() = javaResource.publicDomainNames().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> containerServicePublicDomainNamesToKotlin(args0) })
}).orElse(null)
})
/**
* The Lightsail resource type of the container service (i.e., ContainerService).
*/
public val resourceType: Output
get() = javaResource.resourceType().applyValue({ args0 -> args0 })
/**
* The scale specification for the container service. The scale specifies the allocated compute
* nodes of the container service.
*/
public val scale: Output
get() = javaResource.scale().applyValue({ args0 -> args0 })
/**
* The current state of the container service.
*/
public val state: Output
get() = javaResource.state().applyValue({ args0 -> args0 })
/**
* Map of container service tags. To create a key-only tag, use an empty string as the value. To tag at launch, specify the tags in the Launch Template. If
* configured with a provider
* `default_tags` configuration block
* present, tags with matching keys will overwrite those defined at the provider-level.
*/
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy