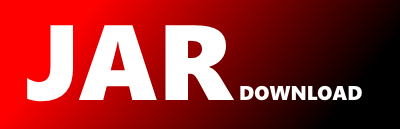
com.pulumi.aws.lightsail.kotlin.inputs.DistributionCacheBehaviorSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.lightsail.kotlin.inputs
import com.pulumi.aws.lightsail.inputs.DistributionCacheBehaviorSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property allowedHttpMethods The HTTP methods that are processed and forwarded to the distribution's origin.
* @property cachedHttpMethods The HTTP method responses that are cached by your distribution.
* @property defaultTtl The default amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the content has been updated.
* @property forwardedCookies An object that describes the cookies that are forwarded to the origin. Your content is cached based on the cookies that are forwarded. Detailed below
* @property forwardedHeaders An object that describes the headers that are forwarded to the origin. Your content is cached based on the headers that are forwarded. Detailed below
* @property forwardedQueryStrings An object that describes the query strings that are forwarded to the origin. Your content is cached based on the query strings that are forwarded. Detailed below
* @property maximumTtl The maximum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
* @property minimumTtl The minimum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
*/
public data class DistributionCacheBehaviorSettingsArgs(
public val allowedHttpMethods: Output? = null,
public val cachedHttpMethods: Output? = null,
public val defaultTtl: Output? = null,
public val forwardedCookies: Output? =
null,
public val forwardedHeaders: Output? =
null,
public val forwardedQueryStrings: Output? = null,
public val maximumTtl: Output? = null,
public val minimumTtl: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.lightsail.inputs.DistributionCacheBehaviorSettingsArgs =
com.pulumi.aws.lightsail.inputs.DistributionCacheBehaviorSettingsArgs.builder()
.allowedHttpMethods(allowedHttpMethods?.applyValue({ args0 -> args0 }))
.cachedHttpMethods(cachedHttpMethods?.applyValue({ args0 -> args0 }))
.defaultTtl(defaultTtl?.applyValue({ args0 -> args0 }))
.forwardedCookies(forwardedCookies?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.forwardedHeaders(forwardedHeaders?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.forwardedQueryStrings(
forwardedQueryStrings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.maximumTtl(maximumTtl?.applyValue({ args0 -> args0 }))
.minimumTtl(minimumTtl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DistributionCacheBehaviorSettingsArgs].
*/
@PulumiTagMarker
public class DistributionCacheBehaviorSettingsArgsBuilder internal constructor() {
private var allowedHttpMethods: Output? = null
private var cachedHttpMethods: Output? = null
private var defaultTtl: Output? = null
private var forwardedCookies: Output? =
null
private var forwardedHeaders: Output? =
null
private var forwardedQueryStrings:
Output? = null
private var maximumTtl: Output? = null
private var minimumTtl: Output? = null
/**
* @param value The HTTP methods that are processed and forwarded to the distribution's origin.
*/
@JvmName("quisxmxojkgsrrek")
public suspend fun allowedHttpMethods(`value`: Output) {
this.allowedHttpMethods = value
}
/**
* @param value The HTTP method responses that are cached by your distribution.
*/
@JvmName("joamfebvugjfoqpl")
public suspend fun cachedHttpMethods(`value`: Output) {
this.cachedHttpMethods = value
}
/**
* @param value The default amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the content has been updated.
*/
@JvmName("xfaytudsdkatkxvc")
public suspend fun defaultTtl(`value`: Output) {
this.defaultTtl = value
}
/**
* @param value An object that describes the cookies that are forwarded to the origin. Your content is cached based on the cookies that are forwarded. Detailed below
*/
@JvmName("jynaftdeiwnmhsxa")
public suspend fun forwardedCookies(`value`: Output) {
this.forwardedCookies = value
}
/**
* @param value An object that describes the headers that are forwarded to the origin. Your content is cached based on the headers that are forwarded. Detailed below
*/
@JvmName("cpurqxnaluqjkpae")
public suspend fun forwardedHeaders(`value`: Output) {
this.forwardedHeaders = value
}
/**
* @param value An object that describes the query strings that are forwarded to the origin. Your content is cached based on the query strings that are forwarded. Detailed below
*/
@JvmName("nupogqvjjhxwrsui")
public suspend fun forwardedQueryStrings(`value`: Output) {
this.forwardedQueryStrings = value
}
/**
* @param value The maximum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
*/
@JvmName("qasiwjsnjyqxviva")
public suspend fun maximumTtl(`value`: Output) {
this.maximumTtl = value
}
/**
* @param value The minimum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
*/
@JvmName("hdocsiowookvaggl")
public suspend fun minimumTtl(`value`: Output) {
this.minimumTtl = value
}
/**
* @param value The HTTP methods that are processed and forwarded to the distribution's origin.
*/
@JvmName("yknnndqueqskgseo")
public suspend fun allowedHttpMethods(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.allowedHttpMethods = mapped
}
/**
* @param value The HTTP method responses that are cached by your distribution.
*/
@JvmName("foanjwecqucaiwuy")
public suspend fun cachedHttpMethods(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cachedHttpMethods = mapped
}
/**
* @param value The default amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the content has been updated.
*/
@JvmName("kiplnbadpbtisbeu")
public suspend fun defaultTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultTtl = mapped
}
/**
* @param value An object that describes the cookies that are forwarded to the origin. Your content is cached based on the cookies that are forwarded. Detailed below
*/
@JvmName("ckdvfgwfyxpvnnjt")
public suspend fun forwardedCookies(`value`: DistributionCacheBehaviorSettingsForwardedCookiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardedCookies = mapped
}
/**
* @param argument An object that describes the cookies that are forwarded to the origin. Your content is cached based on the cookies that are forwarded. Detailed below
*/
@JvmName("mokuxdoavojpvjaj")
public suspend fun forwardedCookies(argument: suspend DistributionCacheBehaviorSettingsForwardedCookiesArgsBuilder.() -> Unit) {
val toBeMapped = DistributionCacheBehaviorSettingsForwardedCookiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardedCookies = mapped
}
/**
* @param value An object that describes the headers that are forwarded to the origin. Your content is cached based on the headers that are forwarded. Detailed below
*/
@JvmName("viwkimuuduhpqknf")
public suspend fun forwardedHeaders(`value`: DistributionCacheBehaviorSettingsForwardedHeadersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardedHeaders = mapped
}
/**
* @param argument An object that describes the headers that are forwarded to the origin. Your content is cached based on the headers that are forwarded. Detailed below
*/
@JvmName("uhatftkewfcrhyrs")
public suspend fun forwardedHeaders(argument: suspend DistributionCacheBehaviorSettingsForwardedHeadersArgsBuilder.() -> Unit) {
val toBeMapped = DistributionCacheBehaviorSettingsForwardedHeadersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardedHeaders = mapped
}
/**
* @param value An object that describes the query strings that are forwarded to the origin. Your content is cached based on the query strings that are forwarded. Detailed below
*/
@JvmName("evmstldmaxuvegva")
public suspend fun forwardedQueryStrings(`value`: DistributionCacheBehaviorSettingsForwardedQueryStringsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.forwardedQueryStrings = mapped
}
/**
* @param argument An object that describes the query strings that are forwarded to the origin. Your content is cached based on the query strings that are forwarded. Detailed below
*/
@JvmName("ipnmgkuaentcgsim")
public suspend fun forwardedQueryStrings(argument: suspend DistributionCacheBehaviorSettingsForwardedQueryStringsArgsBuilder.() -> Unit) {
val toBeMapped =
DistributionCacheBehaviorSettingsForwardedQueryStringsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.forwardedQueryStrings = mapped
}
/**
* @param value The maximum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
*/
@JvmName("knkpigdqoblqasik")
public suspend fun maximumTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maximumTtl = mapped
}
/**
* @param value The minimum amount of time that objects stay in the distribution's cache before the distribution forwards another request to the origin to determine whether the object has been updated.
*/
@JvmName("mafloltqcxealuql")
public suspend fun minimumTtl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minimumTtl = mapped
}
internal fun build(): DistributionCacheBehaviorSettingsArgs =
DistributionCacheBehaviorSettingsArgs(
allowedHttpMethods = allowedHttpMethods,
cachedHttpMethods = cachedHttpMethods,
defaultTtl = defaultTtl,
forwardedCookies = forwardedCookies,
forwardedHeaders = forwardedHeaders,
forwardedQueryStrings = forwardedQueryStrings,
maximumTtl = maximumTtl,
minimumTtl = minimumTtl,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy