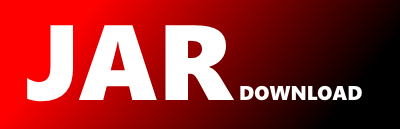
com.pulumi.aws.macie2.kotlin.MemberArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.macie2.kotlin
import com.pulumi.aws.macie2.MemberArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Provides a resource to manage an [Amazon Macie Member](https://docs.aws.amazon.com/macie/latest/APIReference/members-id.html).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as aws from "@pulumi/aws";
* const example = new aws.macie2.Account("example", {});
* const exampleMember = new aws.macie2.Member("example", {
* accountId: "AWS ACCOUNT ID",
* email: "EMAIL",
* invite: true,
* invitationMessage: "Message of the invitation",
* invitationDisableEmailNotification: true,
* }, {
* dependsOn: [example],
* });
* ```
* ```python
* import pulumi
* import pulumi_aws as aws
* example = aws.macie2.Account("example")
* example_member = aws.macie2.Member("example",
* account_id="AWS ACCOUNT ID",
* email="EMAIL",
* invite=True,
* invitation_message="Message of the invitation",
* invitation_disable_email_notification=True,
* opts = pulumi.ResourceOptions(depends_on=[example]))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Aws = Pulumi.Aws;
* return await Deployment.RunAsync(() =>
* {
* var example = new Aws.Macie2.Account("example");
* var exampleMember = new Aws.Macie2.Member("example", new()
* {
* AccountId = "AWS ACCOUNT ID",
* Email = "EMAIL",
* Invite = true,
* InvitationMessage = "Message of the invitation",
* InvitationDisableEmailNotification = true,
* }, new CustomResourceOptions
* {
* DependsOn =
* {
* example,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-aws/sdk/v6/go/aws/macie2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := macie2.NewAccount(ctx, "example", nil)
* if err != nil {
* return err
* }
* _, err = macie2.NewMember(ctx, "example", &macie2.MemberArgs{
* AccountId: pulumi.String("AWS ACCOUNT ID"),
* Email: pulumi.String("EMAIL"),
* Invite: pulumi.Bool(true),
* InvitationMessage: pulumi.String("Message of the invitation"),
* InvitationDisableEmailNotification: pulumi.Bool(true),
* }, pulumi.DependsOn([]pulumi.Resource{
* example,
* }))
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.macie2.Account;
* import com.pulumi.aws.macie2.Member;
* import com.pulumi.aws.macie2.MemberArgs;
* import com.pulumi.resources.CustomResourceOptions;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Account("example");
* var exampleMember = new Member("exampleMember", MemberArgs.builder()
* .accountId("AWS ACCOUNT ID")
* .email("EMAIL")
* .invite(true)
* .invitationMessage("Message of the invitation")
* .invitationDisableEmailNotification(true)
* .build(), CustomResourceOptions.builder()
* .dependsOn(example)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: aws:macie2:Account
* exampleMember:
* type: aws:macie2:Member
* name: example
* properties:
* accountId: AWS ACCOUNT ID
* email: EMAIL
* invite: true
* invitationMessage: Message of the invitation
* invitationDisableEmailNotification: true
* options:
* dependson:
* - ${example}
* ```
*
* ## Import
* Using `pulumi import`, import `aws_macie2_member` using the account ID of the member account. For example:
* ```sh
* $ pulumi import aws:macie2/member:Member example 123456789012
* ```
* @property accountId The AWS account ID for the account.
* @property email The email address for the account.
* @property invitationDisableEmailNotification Specifies whether to send an email notification to the root user of each account that the invitation will be sent to. This notification is in addition to an alert that the root user receives in AWS Personal Health Dashboard. To send an email notification to the root user of each account, set this value to `true`.
* @property invitationMessage A custom message to include in the invitation. Amazon Macie adds this message to the standard content that it sends for an invitation.
* @property invite Send an invitation to a member
* @property status Specifies the status for the account. To enable Amazon Macie and start all Macie activities for the account, set this value to `ENABLED`. Valid values are `ENABLED` or `PAUSED`.
* @property tags A map of key-value pairs that specifies the tags to associate with the account in Amazon Macie.
*/
public data class MemberArgs(
public val accountId: Output? = null,
public val email: Output? = null,
public val invitationDisableEmailNotification: Output? = null,
public val invitationMessage: Output? = null,
public val invite: Output? = null,
public val status: Output? = null,
public val tags: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy