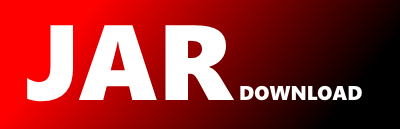
com.pulumi.aws.macie2.kotlin.inputs.ClassificationJobS3JobDefinitionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.macie2.kotlin.inputs
import com.pulumi.aws.macie2.inputs.ClassificationJobS3JobDefinitionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property bucketCriteria The property- and tag-based conditions that determine which S3 buckets to include or exclude from the analysis. Conflicts with `bucket_definitions`. (documented below)
* @property bucketDefinitions An array of objects, one for each AWS account that owns buckets to analyze. Each object specifies the account ID for an account and one or more buckets to analyze for the account. Conflicts with `bucket_criteria`. (documented below)
* @property scoping The property- and tag-based conditions that determine which objects to include or exclude from the analysis. (documented below)
*/
public data class ClassificationJobS3JobDefinitionArgs(
public val bucketCriteria: Output? = null,
public val bucketDefinitions: Output>? =
null,
public val scoping: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.macie2.inputs.ClassificationJobS3JobDefinitionArgs =
com.pulumi.aws.macie2.inputs.ClassificationJobS3JobDefinitionArgs.builder()
.bucketCriteria(bucketCriteria?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.bucketDefinitions(
bucketDefinitions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.scoping(scoping?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ClassificationJobS3JobDefinitionArgs].
*/
@PulumiTagMarker
public class ClassificationJobS3JobDefinitionArgsBuilder internal constructor() {
private var bucketCriteria: Output? = null
private var bucketDefinitions: Output>? =
null
private var scoping: Output? = null
/**
* @param value The property- and tag-based conditions that determine which S3 buckets to include or exclude from the analysis. Conflicts with `bucket_definitions`. (documented below)
*/
@JvmName("unmavvqoqkrrlqpp")
public suspend fun bucketCriteria(`value`: Output) {
this.bucketCriteria = value
}
/**
* @param value An array of objects, one for each AWS account that owns buckets to analyze. Each object specifies the account ID for an account and one or more buckets to analyze for the account. Conflicts with `bucket_criteria`. (documented below)
*/
@JvmName("dudhtayyentrfvvg")
public suspend fun bucketDefinitions(`value`: Output>) {
this.bucketDefinitions = value
}
@JvmName("nctpkghsytdyndaw")
public suspend fun bucketDefinitions(vararg values: Output) {
this.bucketDefinitions = Output.all(values.asList())
}
/**
* @param values An array of objects, one for each AWS account that owns buckets to analyze. Each object specifies the account ID for an account and one or more buckets to analyze for the account. Conflicts with `bucket_criteria`. (documented below)
*/
@JvmName("aldbrugsmimxbvqw")
public suspend fun bucketDefinitions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy