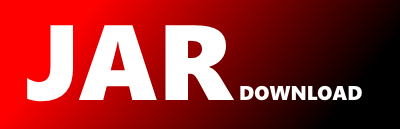
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property bitrate Average bitrate in bits/second.
* @property codingMode Mono, Stereo, or 5.1 channel layout.
* @property inputType Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair.
* @property profile AAC profile.
* @property rateControlMode The rate control mode.
* @property rawFormat Sets LATM/LOAS AAC output for raw containers.
* @property sampleRate Sample rate in Hz.
* @property spec Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
* @property vbrQuality VBR Quality Level - Only used if rateControlMode is VBR.
*/
public data class ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs(
public val bitrate: Output? = null,
public val codingMode: Output? = null,
public val inputType: Output? = null,
public val profile: Output? = null,
public val rateControlMode: Output? = null,
public val rawFormat: Output? = null,
public val sampleRate: Output? = null,
public val spec: Output? = null,
public val vbrQuality: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs.builder()
.bitrate(bitrate?.applyValue({ args0 -> args0 }))
.codingMode(codingMode?.applyValue({ args0 -> args0 }))
.inputType(inputType?.applyValue({ args0 -> args0 }))
.profile(profile?.applyValue({ args0 -> args0 }))
.rateControlMode(rateControlMode?.applyValue({ args0 -> args0 }))
.rawFormat(rawFormat?.applyValue({ args0 -> args0 }))
.sampleRate(sampleRate?.applyValue({ args0 -> args0 }))
.spec(spec?.applyValue({ args0 -> args0 }))
.vbrQuality(vbrQuality?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgsBuilder internal constructor() {
private var bitrate: Output? = null
private var codingMode: Output? = null
private var inputType: Output? = null
private var profile: Output? = null
private var rateControlMode: Output? = null
private var rawFormat: Output? = null
private var sampleRate: Output? = null
private var spec: Output? = null
private var vbrQuality: Output? = null
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("atscwmclryehihpg")
public suspend fun bitrate(`value`: Output) {
this.bitrate = value
}
/**
* @param value Mono, Stereo, or 5.1 channel layout.
*/
@JvmName("bvxdoepoehygocle")
public suspend fun codingMode(`value`: Output) {
this.codingMode = value
}
/**
* @param value Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair.
*/
@JvmName("rbnkjtecscujlocg")
public suspend fun inputType(`value`: Output) {
this.inputType = value
}
/**
* @param value AAC profile.
*/
@JvmName("jjkphrcdiojbthwq")
public suspend fun profile(`value`: Output) {
this.profile = value
}
/**
* @param value The rate control mode.
*/
@JvmName("xoxfyrelstmqygkr")
public suspend fun rateControlMode(`value`: Output) {
this.rateControlMode = value
}
/**
* @param value Sets LATM/LOAS AAC output for raw containers.
*/
@JvmName("wcypisctchyhghid")
public suspend fun rawFormat(`value`: Output) {
this.rawFormat = value
}
/**
* @param value Sample rate in Hz.
*/
@JvmName("lmtnthwcrlotiwoa")
public suspend fun sampleRate(`value`: Output) {
this.sampleRate = value
}
/**
* @param value Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
*/
@JvmName("tpieoxwbwhqyfjqy")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value VBR Quality Level - Only used if rateControlMode is VBR.
*/
@JvmName("qktpjklojgcscenb")
public suspend fun vbrQuality(`value`: Output) {
this.vbrQuality = value
}
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("lbktlyilohbmybbd")
public suspend fun bitrate(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bitrate = mapped
}
/**
* @param value Mono, Stereo, or 5.1 channel layout.
*/
@JvmName("cbenybofyhthrcwd")
public suspend fun codingMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codingMode = mapped
}
/**
* @param value Set to "broadcasterMixedAd" when input contains pre-mixed main audio + AD (narration) as a stereo pair.
*/
@JvmName("ynseeookwrvcnrcd")
public suspend fun inputType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputType = mapped
}
/**
* @param value AAC profile.
*/
@JvmName("smdxgvxabgwtaaqg")
public suspend fun profile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.profile = mapped
}
/**
* @param value The rate control mode.
*/
@JvmName("gibhpyufnnjoknte")
public suspend fun rateControlMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rateControlMode = mapped
}
/**
* @param value Sets LATM/LOAS AAC output for raw containers.
*/
@JvmName("rfppchmmnkmuyydv")
public suspend fun rawFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rawFormat = mapped
}
/**
* @param value Sample rate in Hz.
*/
@JvmName("jtfxchdxvxjfbkex")
public suspend fun sampleRate(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sampleRate = mapped
}
/**
* @param value Use MPEG-2 AAC audio instead of MPEG-4 AAC audio for raw or MPEG-2 Transport Stream containers.
*/
@JvmName("ikdbhrlhlhybejtv")
public suspend fun spec(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param value VBR Quality Level - Only used if rateControlMode is VBR.
*/
@JvmName("ucaisfspkehgltsu")
public suspend fun vbrQuality(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vbrQuality = mapped
}
internal fun build(): ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs =
ChannelEncoderSettingsAudioDescriptionCodecSettingsAacSettingsArgs(
bitrate = bitrate,
codingMode = codingMode,
inputType = inputType,
profile = profile,
rateControlMode = rateControlMode,
rawFormat = rawFormat,
sampleRate = sampleRate,
spec = spec,
vbrQuality = vbrQuality,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy