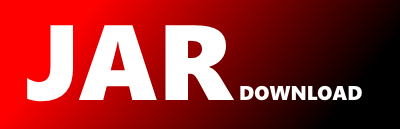
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property attenuationControl Sets the attenuation control.
* @property bitrate Average bitrate in bits/second.
* @property bitstreamMode Specifies the bitstream mode (bsmod) for the emitted AC-3 stream.
* @property codingMode Dolby Digital Plus coding mode.
* @property dcFilter
* @property dialnorm
* @property drcLine Sets the Dolby dynamic range compression profile.
* @property drcRf Sets the profile for heavy Dolby dynamic range compression.
* @property lfeControl
* @property lfeFilter When set to enabled, applies a 120Hz lowpass filter to the LFE channel prior to encoding.
* @property loRoCenterMixLevel
* @property loRoSurroundMixLevel
* @property ltRtCenterMixLevel
* @property ltRtSurroundMixLevel
* @property metadataControl Metadata control.
* @property passthroughControl
* @property phaseControl
* @property stereoDownmix
* @property surroundExMode
* @property surroundMode
*/
public data class ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs(
public val attenuationControl: Output? = null,
public val bitrate: Output? = null,
public val bitstreamMode: Output? = null,
public val codingMode: Output? = null,
public val dcFilter: Output? = null,
public val dialnorm: Output? = null,
public val drcLine: Output? = null,
public val drcRf: Output? = null,
public val lfeControl: Output? = null,
public val lfeFilter: Output? = null,
public val loRoCenterMixLevel: Output? = null,
public val loRoSurroundMixLevel: Output? = null,
public val ltRtCenterMixLevel: Output? = null,
public val ltRtSurroundMixLevel: Output? = null,
public val metadataControl: Output? = null,
public val passthroughControl: Output? = null,
public val phaseControl: Output? = null,
public val stereoDownmix: Output? = null,
public val surroundExMode: Output? = null,
public val surroundMode: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs.builder()
.attenuationControl(attenuationControl?.applyValue({ args0 -> args0 }))
.bitrate(bitrate?.applyValue({ args0 -> args0 }))
.bitstreamMode(bitstreamMode?.applyValue({ args0 -> args0 }))
.codingMode(codingMode?.applyValue({ args0 -> args0 }))
.dcFilter(dcFilter?.applyValue({ args0 -> args0 }))
.dialnorm(dialnorm?.applyValue({ args0 -> args0 }))
.drcLine(drcLine?.applyValue({ args0 -> args0 }))
.drcRf(drcRf?.applyValue({ args0 -> args0 }))
.lfeControl(lfeControl?.applyValue({ args0 -> args0 }))
.lfeFilter(lfeFilter?.applyValue({ args0 -> args0 }))
.loRoCenterMixLevel(loRoCenterMixLevel?.applyValue({ args0 -> args0 }))
.loRoSurroundMixLevel(loRoSurroundMixLevel?.applyValue({ args0 -> args0 }))
.ltRtCenterMixLevel(ltRtCenterMixLevel?.applyValue({ args0 -> args0 }))
.ltRtSurroundMixLevel(ltRtSurroundMixLevel?.applyValue({ args0 -> args0 }))
.metadataControl(metadataControl?.applyValue({ args0 -> args0 }))
.passthroughControl(passthroughControl?.applyValue({ args0 -> args0 }))
.phaseControl(phaseControl?.applyValue({ args0 -> args0 }))
.stereoDownmix(stereoDownmix?.applyValue({ args0 -> args0 }))
.surroundExMode(surroundExMode?.applyValue({ args0 -> args0 }))
.surroundMode(surroundMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgsBuilder internal constructor() {
private var attenuationControl: Output? = null
private var bitrate: Output? = null
private var bitstreamMode: Output? = null
private var codingMode: Output? = null
private var dcFilter: Output? = null
private var dialnorm: Output? = null
private var drcLine: Output? = null
private var drcRf: Output? = null
private var lfeControl: Output? = null
private var lfeFilter: Output? = null
private var loRoCenterMixLevel: Output? = null
private var loRoSurroundMixLevel: Output? = null
private var ltRtCenterMixLevel: Output? = null
private var ltRtSurroundMixLevel: Output? = null
private var metadataControl: Output? = null
private var passthroughControl: Output? = null
private var phaseControl: Output? = null
private var stereoDownmix: Output? = null
private var surroundExMode: Output? = null
private var surroundMode: Output? = null
/**
* @param value Sets the attenuation control.
*/
@JvmName("fwvvgjaptnjtpnby")
public suspend fun attenuationControl(`value`: Output) {
this.attenuationControl = value
}
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("qvewhejxduapcnbp")
public suspend fun bitrate(`value`: Output) {
this.bitrate = value
}
/**
* @param value Specifies the bitstream mode (bsmod) for the emitted AC-3 stream.
*/
@JvmName("ayvckuvlwfauqoev")
public suspend fun bitstreamMode(`value`: Output) {
this.bitstreamMode = value
}
/**
* @param value Dolby Digital Plus coding mode.
*/
@JvmName("sydnaavwhvegotnt")
public suspend fun codingMode(`value`: Output) {
this.codingMode = value
}
/**
* @param value
*/
@JvmName("dqghalriaidnahob")
public suspend fun dcFilter(`value`: Output) {
this.dcFilter = value
}
/**
* @param value
*/
@JvmName("kfwwdycrnkpixojt")
public suspend fun dialnorm(`value`: Output) {
this.dialnorm = value
}
/**
* @param value Sets the Dolby dynamic range compression profile.
*/
@JvmName("jtkqiupqqahjyvls")
public suspend fun drcLine(`value`: Output) {
this.drcLine = value
}
/**
* @param value Sets the profile for heavy Dolby dynamic range compression.
*/
@JvmName("mssnvuoemjhtqgjq")
public suspend fun drcRf(`value`: Output) {
this.drcRf = value
}
/**
* @param value
*/
@JvmName("lksgidtvfwyroapr")
public suspend fun lfeControl(`value`: Output) {
this.lfeControl = value
}
/**
* @param value When set to enabled, applies a 120Hz lowpass filter to the LFE channel prior to encoding.
*/
@JvmName("bvwxckxvxcbvdrrx")
public suspend fun lfeFilter(`value`: Output) {
this.lfeFilter = value
}
/**
* @param value
*/
@JvmName("aciiearmafkxbxsv")
public suspend fun loRoCenterMixLevel(`value`: Output) {
this.loRoCenterMixLevel = value
}
/**
* @param value
*/
@JvmName("aafkguqsgptufspn")
public suspend fun loRoSurroundMixLevel(`value`: Output) {
this.loRoSurroundMixLevel = value
}
/**
* @param value
*/
@JvmName("gmjpqlssapxxsqhg")
public suspend fun ltRtCenterMixLevel(`value`: Output) {
this.ltRtCenterMixLevel = value
}
/**
* @param value
*/
@JvmName("gytajfwghmbmnndu")
public suspend fun ltRtSurroundMixLevel(`value`: Output) {
this.ltRtSurroundMixLevel = value
}
/**
* @param value Metadata control.
*/
@JvmName("htnpbkikedphkvir")
public suspend fun metadataControl(`value`: Output) {
this.metadataControl = value
}
/**
* @param value
*/
@JvmName("ggytefmtectagicj")
public suspend fun passthroughControl(`value`: Output) {
this.passthroughControl = value
}
/**
* @param value
*/
@JvmName("jpuguwdsqccmwdpq")
public suspend fun phaseControl(`value`: Output) {
this.phaseControl = value
}
/**
* @param value
*/
@JvmName("oiqibrgduwsaphpq")
public suspend fun stereoDownmix(`value`: Output) {
this.stereoDownmix = value
}
/**
* @param value
*/
@JvmName("tmpsylmhlcdetshv")
public suspend fun surroundExMode(`value`: Output) {
this.surroundExMode = value
}
/**
* @param value
*/
@JvmName("fmuhhvwcvikwkypd")
public suspend fun surroundMode(`value`: Output) {
this.surroundMode = value
}
/**
* @param value Sets the attenuation control.
*/
@JvmName("snudcgojkqvgejyc")
public suspend fun attenuationControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.attenuationControl = mapped
}
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("rirfxhdixlentnef")
public suspend fun bitrate(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bitrate = mapped
}
/**
* @param value Specifies the bitstream mode (bsmod) for the emitted AC-3 stream.
*/
@JvmName("vrwvocjmkinlrotq")
public suspend fun bitstreamMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bitstreamMode = mapped
}
/**
* @param value Dolby Digital Plus coding mode.
*/
@JvmName("diqlbfcymuyhenpu")
public suspend fun codingMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codingMode = mapped
}
/**
* @param value
*/
@JvmName("arahnxtqtviclvlb")
public suspend fun dcFilter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dcFilter = mapped
}
/**
* @param value
*/
@JvmName("axcticijwismxsgn")
public suspend fun dialnorm(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dialnorm = mapped
}
/**
* @param value Sets the Dolby dynamic range compression profile.
*/
@JvmName("mnqaxoxethpbapaj")
public suspend fun drcLine(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.drcLine = mapped
}
/**
* @param value Sets the profile for heavy Dolby dynamic range compression.
*/
@JvmName("hdillnmemksdfkut")
public suspend fun drcRf(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.drcRf = mapped
}
/**
* @param value
*/
@JvmName("cfowyphshjbgqkfr")
public suspend fun lfeControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lfeControl = mapped
}
/**
* @param value When set to enabled, applies a 120Hz lowpass filter to the LFE channel prior to encoding.
*/
@JvmName("knsweghoqdytsubg")
public suspend fun lfeFilter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lfeFilter = mapped
}
/**
* @param value
*/
@JvmName("nutbxpcyqdwaehrh")
public suspend fun loRoCenterMixLevel(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.loRoCenterMixLevel = mapped
}
/**
* @param value
*/
@JvmName("sfxsnnulplltprcf")
public suspend fun loRoSurroundMixLevel(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.loRoSurroundMixLevel = mapped
}
/**
* @param value
*/
@JvmName("mmmworctgsqojjoy")
public suspend fun ltRtCenterMixLevel(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ltRtCenterMixLevel = mapped
}
/**
* @param value
*/
@JvmName("eviounkmykqlpsgn")
public suspend fun ltRtSurroundMixLevel(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ltRtSurroundMixLevel = mapped
}
/**
* @param value Metadata control.
*/
@JvmName("rxsxnmtxrndwvnjv")
public suspend fun metadataControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadataControl = mapped
}
/**
* @param value
*/
@JvmName("stybvkgupjqhlcpl")
public suspend fun passthroughControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passthroughControl = mapped
}
/**
* @param value
*/
@JvmName("jdpjiaokfebdtxjj")
public suspend fun phaseControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phaseControl = mapped
}
/**
* @param value
*/
@JvmName("pgtfiskkjlyjwptk")
public suspend fun stereoDownmix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stereoDownmix = mapped
}
/**
* @param value
*/
@JvmName("lxrnhwdwchsvmdyv")
public suspend fun surroundExMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.surroundExMode = mapped
}
/**
* @param value
*/
@JvmName("wjbhehokfafbwmyb")
public suspend fun surroundMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.surroundMode = mapped
}
internal fun build(): ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs =
ChannelEncoderSettingsAudioDescriptionCodecSettingsEac3SettingsArgs(
attenuationControl = attenuationControl,
bitrate = bitrate,
bitstreamMode = bitstreamMode,
codingMode = codingMode,
dcFilter = dcFilter,
dialnorm = dialnorm,
drcLine = drcLine,
drcRf = drcRf,
lfeControl = lfeControl,
lfeFilter = lfeFilter,
loRoCenterMixLevel = loRoCenterMixLevel,
loRoSurroundMixLevel = loRoSurroundMixLevel,
ltRtCenterMixLevel = ltRtCenterMixLevel,
ltRtSurroundMixLevel = ltRtSurroundMixLevel,
metadataControl = metadataControl,
passthroughControl = passthroughControl,
phaseControl = phaseControl,
stereoDownmix = stereoDownmix,
surroundExMode = surroundExMode,
surroundMode = surroundMode,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy