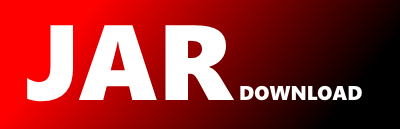
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property alignment If no explicit xPosition or yPosition is provided, setting alignment to centered will place the captions at the bottom center of the output. Similarly, setting a left alignment will align captions to the bottom left of the output. If x and y positions are given in conjunction with the alignment parameter, the font will be justified (either left or centered) relative to those coordinates. Selecting “smart” justification will left-justify live subtitles and center-justify pre-recorded subtitles. This option is not valid for source captions that are STL or 608/embedded. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
* @property backgroundColor Specifies the color of the rectangle behind the captions. All burn-in and DVB-Sub font settings must match.
* @property backgroundOpacity Specifies the opacity of the background rectangle. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
* @property font External font file used for caption burn-in. File extension must be ‘ttf’ or ‘tte’. Although the user can select output fonts for many different types of input captions, embedded, STL and teletext sources use a strict grid system. Using external fonts with these caption sources could cause unexpected display of proportional fonts. All burn-in and DVB-Sub font settings must match. See Font for more details.
* @property fontColor Specifies the color of the burned-in captions. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
* @property fontOpacity Specifies the opacity of the burned-in captions. 255 is opaque; 0 is transparent. All burn-in and DVB-Sub font settings must match.
* @property fontResolution Font resolution in DPI (dots per inch); default is 96 dpi. All burn-in and DVB-Sub font settings must match.
* @property fontSize When set to auto fontSize will scale depending on the size of the output. Giving a positive integer will specify the exact font size in points. All burn-in and DVB-Sub font settings must match.
* @property outlineColor Specifies font outline color. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
* @property outlineSize Specifies font outline size in pixels. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
* @property shadowColor Specifies the color of the shadow cast by the captions. All burn-in and DVB-Sub font settings must match.
* @property shadowOpacity Specifies the opacity of the shadow. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
* @property shadowXOffset Specifies the horizontal offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels to the left. All burn-in and DVB-Sub font settings must match.
* @property shadowYOffset Specifies the vertical offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels above the text. All burn-in and DVB-Sub font settings must match.
* @property teletextGridControl Controls whether a fixed grid size will be used to generate the output subtitles bitmap. Only applicable for Teletext inputs and DVB-Sub/Burn-in outputs.
* @property xPosition Specifies the horizontal position of the caption relative to the left side of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the left of the output. If no explicit xPosition is provided, the horizontal caption position will be determined by the alignment parameter. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
* @property yPosition Specifies the vertical position of the caption relative to the top of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the top of the output. If no explicit yPosition is provided, the caption will be positioned towards the bottom of the output. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
public data class
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs(
public val alignment: Output? = null,
public val backgroundColor: Output? = null,
public val backgroundOpacity: Output? = null,
public val font: Output? =
null,
public val fontColor: Output? = null,
public val fontOpacity: Output? = null,
public val fontResolution: Output? = null,
public val fontSize: Output? = null,
public val outlineColor: Output? = null,
public val outlineSize: Output? = null,
public val shadowColor: Output? = null,
public val shadowOpacity: Output? = null,
public val shadowXOffset: Output? = null,
public val shadowYOffset: Output? = null,
public val teletextGridControl: Output? = null,
public val xPosition: Output? = null,
public val yPosition: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs.builder()
.alignment(alignment?.applyValue({ args0 -> args0 }))
.backgroundColor(backgroundColor?.applyValue({ args0 -> args0 }))
.backgroundOpacity(backgroundOpacity?.applyValue({ args0 -> args0 }))
.font(font?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fontColor(fontColor?.applyValue({ args0 -> args0 }))
.fontOpacity(fontOpacity?.applyValue({ args0 -> args0 }))
.fontResolution(fontResolution?.applyValue({ args0 -> args0 }))
.fontSize(fontSize?.applyValue({ args0 -> args0 }))
.outlineColor(outlineColor?.applyValue({ args0 -> args0 }))
.outlineSize(outlineSize?.applyValue({ args0 -> args0 }))
.shadowColor(shadowColor?.applyValue({ args0 -> args0 }))
.shadowOpacity(shadowOpacity?.applyValue({ args0 -> args0 }))
.shadowXOffset(shadowXOffset?.applyValue({ args0 -> args0 }))
.shadowYOffset(shadowYOffset?.applyValue({ args0 -> args0 }))
.teletextGridControl(teletextGridControl?.applyValue({ args0 -> args0 }))
.xPosition(xPosition?.applyValue({ args0 -> args0 }))
.yPosition(yPosition?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs].
*/
@PulumiTagMarker
public class
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgsBuilder
internal constructor() {
private var alignment: Output? = null
private var backgroundColor: Output? = null
private var backgroundOpacity: Output? = null
private var font:
Output? =
null
private var fontColor: Output? = null
private var fontOpacity: Output? = null
private var fontResolution: Output? = null
private var fontSize: Output? = null
private var outlineColor: Output? = null
private var outlineSize: Output? = null
private var shadowColor: Output? = null
private var shadowOpacity: Output? = null
private var shadowXOffset: Output? = null
private var shadowYOffset: Output? = null
private var teletextGridControl: Output? = null
private var xPosition: Output? = null
private var yPosition: Output? = null
/**
* @param value If no explicit xPosition or yPosition is provided, setting alignment to centered will place the captions at the bottom center of the output. Similarly, setting a left alignment will align captions to the bottom left of the output. If x and y positions are given in conjunction with the alignment parameter, the font will be justified (either left or centered) relative to those coordinates. Selecting “smart” justification will left-justify live subtitles and center-justify pre-recorded subtitles. This option is not valid for source captions that are STL or 608/embedded. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("rlrltkhiwsxuidyi")
public suspend fun alignment(`value`: Output) {
this.alignment = value
}
/**
* @param value Specifies the color of the rectangle behind the captions. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("jmycorstlyjhuwqu")
public suspend fun backgroundColor(`value`: Output) {
this.backgroundColor = value
}
/**
* @param value Specifies the opacity of the background rectangle. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
*/
@JvmName("mirkykyircypjsju")
public suspend fun backgroundOpacity(`value`: Output) {
this.backgroundOpacity = value
}
/**
* @param value External font file used for caption burn-in. File extension must be ‘ttf’ or ‘tte’. Although the user can select output fonts for many different types of input captions, embedded, STL and teletext sources use a strict grid system. Using external fonts with these caption sources could cause unexpected display of proportional fonts. All burn-in and DVB-Sub font settings must match. See Font for more details.
*/
@JvmName("apogrusinjjeiett")
public suspend fun font(`value`: Output) {
this.font = value
}
/**
* @param value Specifies the color of the burned-in captions. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("hfrguiskfribbpnf")
public suspend fun fontColor(`value`: Output) {
this.fontColor = value
}
/**
* @param value Specifies the opacity of the burned-in captions. 255 is opaque; 0 is transparent. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("rrrkemuocbqovfjl")
public suspend fun fontOpacity(`value`: Output) {
this.fontOpacity = value
}
/**
* @param value Font resolution in DPI (dots per inch); default is 96 dpi. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("uxupdpmemerymppv")
public suspend fun fontResolution(`value`: Output) {
this.fontResolution = value
}
/**
* @param value When set to auto fontSize will scale depending on the size of the output. Giving a positive integer will specify the exact font size in points. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("upwynjuiqbvjxtyw")
public suspend fun fontSize(`value`: Output) {
this.fontSize = value
}
/**
* @param value Specifies font outline color. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("mrgboidwrbohprmx")
public suspend fun outlineColor(`value`: Output) {
this.outlineColor = value
}
/**
* @param value Specifies font outline size in pixels. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("dyfkgakppyuxfnvf")
public suspend fun outlineSize(`value`: Output) {
this.outlineSize = value
}
/**
* @param value Specifies the color of the shadow cast by the captions. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("dihrrwcuopegvdhs")
public suspend fun shadowColor(`value`: Output) {
this.shadowColor = value
}
/**
* @param value Specifies the opacity of the shadow. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
*/
@JvmName("ajksixlvexddpnjo")
public suspend fun shadowOpacity(`value`: Output) {
this.shadowOpacity = value
}
/**
* @param value Specifies the horizontal offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels to the left. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("aepexsgdcikypwlq")
public suspend fun shadowXOffset(`value`: Output) {
this.shadowXOffset = value
}
/**
* @param value Specifies the vertical offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels above the text. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("uueqaaixqjiwtaql")
public suspend fun shadowYOffset(`value`: Output) {
this.shadowYOffset = value
}
/**
* @param value Controls whether a fixed grid size will be used to generate the output subtitles bitmap. Only applicable for Teletext inputs and DVB-Sub/Burn-in outputs.
*/
@JvmName("gcyovtedkwvqmpfb")
public suspend fun teletextGridControl(`value`: Output) {
this.teletextGridControl = value
}
/**
* @param value Specifies the horizontal position of the caption relative to the left side of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the left of the output. If no explicit xPosition is provided, the horizontal caption position will be determined by the alignment parameter. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("bfwwvappuvjjijfb")
public suspend fun xPosition(`value`: Output) {
this.xPosition = value
}
/**
* @param value Specifies the vertical position of the caption relative to the top of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the top of the output. If no explicit yPosition is provided, the caption will be positioned towards the bottom of the output. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("ruicopytjtvduwtv")
public suspend fun yPosition(`value`: Output) {
this.yPosition = value
}
/**
* @param value If no explicit xPosition or yPosition is provided, setting alignment to centered will place the captions at the bottom center of the output. Similarly, setting a left alignment will align captions to the bottom left of the output. If x and y positions are given in conjunction with the alignment parameter, the font will be justified (either left or centered) relative to those coordinates. Selecting “smart” justification will left-justify live subtitles and center-justify pre-recorded subtitles. This option is not valid for source captions that are STL or 608/embedded. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("uksumlhbtikwvcry")
public suspend fun alignment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alignment = mapped
}
/**
* @param value Specifies the color of the rectangle behind the captions. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("kbsmyglfvtreaucc")
public suspend fun backgroundColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundColor = mapped
}
/**
* @param value Specifies the opacity of the background rectangle. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
*/
@JvmName("gvrxsqmndhhghvsg")
public suspend fun backgroundOpacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundOpacity = mapped
}
/**
* @param value External font file used for caption burn-in. File extension must be ‘ttf’ or ‘tte’. Although the user can select output fonts for many different types of input captions, embedded, STL and teletext sources use a strict grid system. Using external fonts with these caption sources could cause unexpected display of proportional fonts. All burn-in and DVB-Sub font settings must match. See Font for more details.
*/
@JvmName("wbvuykfaasfnvjqh")
public suspend fun font(`value`: ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsFontArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.font = mapped
}
/**
* @param argument External font file used for caption burn-in. File extension must be ‘ttf’ or ‘tte’. Although the user can select output fonts for many different types of input captions, embedded, STL and teletext sources use a strict grid system. Using external fonts with these caption sources could cause unexpected display of proportional fonts. All burn-in and DVB-Sub font settings must match. See Font for more details.
*/
@JvmName("ipldbfwjjofjcpfx")
public suspend fun font(argument: suspend ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsFontArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsFontArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.font = mapped
}
/**
* @param value Specifies the color of the burned-in captions. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("yytoyhiswuesxahh")
public suspend fun fontColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontColor = mapped
}
/**
* @param value Specifies the opacity of the burned-in captions. 255 is opaque; 0 is transparent. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("pxwagryavipxafra")
public suspend fun fontOpacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontOpacity = mapped
}
/**
* @param value Font resolution in DPI (dots per inch); default is 96 dpi. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("cflthqbfbnobqugv")
public suspend fun fontResolution(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontResolution = mapped
}
/**
* @param value When set to auto fontSize will scale depending on the size of the output. Giving a positive integer will specify the exact font size in points. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("fuigibbwmmduqkwe")
public suspend fun fontSize(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontSize = mapped
}
/**
* @param value Specifies font outline color. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("mecerdxnuyladehu")
public suspend fun outlineColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outlineColor = mapped
}
/**
* @param value Specifies font outline size in pixels. This option is not valid for source captions that are either 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("kjdttqpldahafnjj")
public suspend fun outlineSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outlineSize = mapped
}
/**
* @param value Specifies the color of the shadow cast by the captions. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("dhdfloawbemdullm")
public suspend fun shadowColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shadowColor = mapped
}
/**
* @param value Specifies the opacity of the shadow. 255 is opaque; 0 is transparent. Leaving this parameter blank is equivalent to setting it to 0 (transparent). All burn-in and DVB-Sub font settings must match.
*/
@JvmName("howbgyiyhklngfsx")
public suspend fun shadowOpacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shadowOpacity = mapped
}
/**
* @param value Specifies the horizontal offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels to the left. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("dquwpretushgendf")
public suspend fun shadowXOffset(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shadowXOffset = mapped
}
/**
* @param value Specifies the vertical offset of the shadow relative to the captions in pixels. A value of -2 would result in a shadow offset 2 pixels above the text. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("vtqcewyenmlftljy")
public suspend fun shadowYOffset(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shadowYOffset = mapped
}
/**
* @param value Controls whether a fixed grid size will be used to generate the output subtitles bitmap. Only applicable for Teletext inputs and DVB-Sub/Burn-in outputs.
*/
@JvmName("dsxlwkydpmobtubo")
public suspend fun teletextGridControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.teletextGridControl = mapped
}
/**
* @param value Specifies the horizontal position of the caption relative to the left side of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the left of the output. If no explicit xPosition is provided, the horizontal caption position will be determined by the alignment parameter. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("nbnfyuitollmdfkx")
public suspend fun xPosition(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.xPosition = mapped
}
/**
* @param value Specifies the vertical position of the caption relative to the top of the output in pixels. A value of 10 would result in the captions starting 10 pixels from the top of the output. If no explicit yPosition is provided, the caption will be positioned towards the bottom of the output. This option is not valid for source captions that are STL, 608/embedded or teletext. These source settings are already pre-defined by the caption stream. All burn-in and DVB-Sub font settings must match.
*/
@JvmName("osuutsvocewbactv")
public suspend fun yPosition(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.yPosition = mapped
}
internal fun build(): ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs =
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsDvbSubDestinationSettingsArgs(
alignment = alignment,
backgroundColor = backgroundColor,
backgroundOpacity = backgroundOpacity,
font = font,
fontColor = fontColor,
fontOpacity = fontOpacity,
fontResolution = fontResolution,
fontSize = fontSize,
outlineColor = outlineColor,
outlineSize = outlineSize,
shadowColor = shadowColor,
shadowOpacity = shadowOpacity,
shadowXOffset = shadowXOffset,
shadowYOffset = shadowYOffset,
teletextGridControl = teletextGridControl,
xPosition = xPosition,
yPosition = yPosition,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy