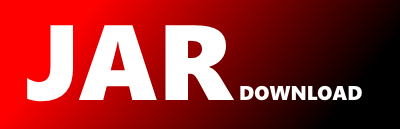
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property copyrightHolder Complete this field if you want to include the name of the copyright holder in the copyright tag in the captions metadata.
* @property fillLineGap Specifies how to handle the gap between the lines (in multi-line captions). - enabled: Fill with the captions background color (as specified in the input captions). - disabled: Leave the gap unfilled.
* @property fontFamily Specifies the font family to include in the font data attached to the EBU-TT captions. Valid only if styleControl is set to include. If you leave this field empty, the font family is set to “monospaced”. (If styleControl is set to exclude, the font family is always set to “monospaced”.) You specify only the font family. All other style information (color, bold, position and so on) is copied from the input captions. The size is always set to 100% to allow the downstream player to choose the size. - Enter a list of font families, as a comma-separated list of font names, in order of preference. The name can be a font family (such as “Arial”), or a generic font family (such as “serif”), or “default” (to let the downstream player choose the font). - Leave blank to set the family to “monospace”.
* @property styleControl Specifies the style information (font color, font position, and so on) to include in the font data that is attached to the EBU-TT captions. - include: Take the style information (font color, font position, and so on) from the source captions and include that information in the font data attached to the EBU-TT captions. This option is valid only if the source captions are Embedded or Teletext. - exclude: In the font data attached to the EBU-TT captions, set the font family to “monospaced”. Do not include any other style information.
*/
public data class
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs(
public val copyrightHolder: Output? = null,
public val fillLineGap: Output? = null,
public val fontFamily: Output? = null,
public val styleControl: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs.builder()
.copyrightHolder(copyrightHolder?.applyValue({ args0 -> args0 }))
.fillLineGap(fillLineGap?.applyValue({ args0 -> args0 }))
.fontFamily(fontFamily?.applyValue({ args0 -> args0 }))
.styleControl(styleControl?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs].
*/
@PulumiTagMarker
public class
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgsBuilder
internal constructor() {
private var copyrightHolder: Output? = null
private var fillLineGap: Output? = null
private var fontFamily: Output? = null
private var styleControl: Output? = null
/**
* @param value Complete this field if you want to include the name of the copyright holder in the copyright tag in the captions metadata.
*/
@JvmName("vshusnrmcmeuwequ")
public suspend fun copyrightHolder(`value`: Output) {
this.copyrightHolder = value
}
/**
* @param value Specifies how to handle the gap between the lines (in multi-line captions). - enabled: Fill with the captions background color (as specified in the input captions). - disabled: Leave the gap unfilled.
*/
@JvmName("aocirheqtvfhlwwh")
public suspend fun fillLineGap(`value`: Output) {
this.fillLineGap = value
}
/**
* @param value Specifies the font family to include in the font data attached to the EBU-TT captions. Valid only if styleControl is set to include. If you leave this field empty, the font family is set to “monospaced”. (If styleControl is set to exclude, the font family is always set to “monospaced”.) You specify only the font family. All other style information (color, bold, position and so on) is copied from the input captions. The size is always set to 100% to allow the downstream player to choose the size. - Enter a list of font families, as a comma-separated list of font names, in order of preference. The name can be a font family (such as “Arial”), or a generic font family (such as “serif”), or “default” (to let the downstream player choose the font). - Leave blank to set the family to “monospace”.
*/
@JvmName("flympgeephaipjtp")
public suspend fun fontFamily(`value`: Output) {
this.fontFamily = value
}
/**
* @param value Specifies the style information (font color, font position, and so on) to include in the font data that is attached to the EBU-TT captions. - include: Take the style information (font color, font position, and so on) from the source captions and include that information in the font data attached to the EBU-TT captions. This option is valid only if the source captions are Embedded or Teletext. - exclude: In the font data attached to the EBU-TT captions, set the font family to “monospaced”. Do not include any other style information.
*/
@JvmName("rnoeivaecqilafgl")
public suspend fun styleControl(`value`: Output) {
this.styleControl = value
}
/**
* @param value Complete this field if you want to include the name of the copyright holder in the copyright tag in the captions metadata.
*/
@JvmName("rifnardqeqyttsod")
public suspend fun copyrightHolder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.copyrightHolder = mapped
}
/**
* @param value Specifies how to handle the gap between the lines (in multi-line captions). - enabled: Fill with the captions background color (as specified in the input captions). - disabled: Leave the gap unfilled.
*/
@JvmName("ympocjuvcffqrnwx")
public suspend fun fillLineGap(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fillLineGap = mapped
}
/**
* @param value Specifies the font family to include in the font data attached to the EBU-TT captions. Valid only if styleControl is set to include. If you leave this field empty, the font family is set to “monospaced”. (If styleControl is set to exclude, the font family is always set to “monospaced”.) You specify only the font family. All other style information (color, bold, position and so on) is copied from the input captions. The size is always set to 100% to allow the downstream player to choose the size. - Enter a list of font families, as a comma-separated list of font names, in order of preference. The name can be a font family (such as “Arial”), or a generic font family (such as “serif”), or “default” (to let the downstream player choose the font). - Leave blank to set the family to “monospace”.
*/
@JvmName("rokbsltdhnciboeb")
public suspend fun fontFamily(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontFamily = mapped
}
/**
* @param value Specifies the style information (font color, font position, and so on) to include in the font data that is attached to the EBU-TT captions. - include: Take the style information (font color, font position, and so on) from the source captions and include that information in the font data attached to the EBU-TT captions. This option is valid only if the source captions are Embedded or Teletext. - exclude: In the font data attached to the EBU-TT captions, set the font family to “monospaced”. Do not include any other style information.
*/
@JvmName("dwrqijqffjjbxwiw")
public suspend fun styleControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.styleControl = mapped
}
internal fun build(): ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs =
ChannelEncoderSettingsCaptionDescriptionDestinationSettingsEbuTtDDestinationSettingsArgs(
copyrightHolder = copyrightHolder,
fillLineGap = fillLineGap,
fontFamily = fontFamily,
styleControl = styleControl,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy