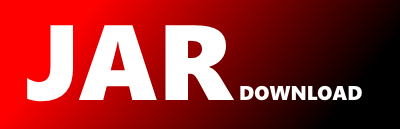
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsGlobalConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsGlobalConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property initialAudioGain Value to set the initial audio gain for the Live Event.
* @property inputEndAction Indicates the action to take when the current input completes (e.g. end-of-file). When switchAndLoopInputs is configured the encoder will restart at the beginning of the first input. When “none” is configured the encoder will transcode either black, a solid color, or a user specified slate images per the “Input Loss Behavior” configuration until the next input switch occurs (which is controlled through the Channel Schedule API).
* @property inputLossBehavior Settings for system actions when input is lost. See Input Loss Behavior for more details.
* @property outputLockingMode Indicates how MediaLive pipelines are synchronized. PIPELINE\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the other. EPOCH\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the Unix epoch.
* @property outputTimingSource Indicates whether the rate of frames emitted by the Live encoder should be paced by its system clock (which optionally may be locked to another source via NTP) or should be locked to the clock of the source that is providing the input stream.
* @property supportLowFramerateInputs Adjusts video input buffer for streams with very low video framerates. This is commonly set to enabled for music channels with less than one video frame per second.
*/
public data class ChannelEncoderSettingsGlobalConfigurationArgs(
public val initialAudioGain: Output? = null,
public val inputEndAction: Output? = null,
public val inputLossBehavior: Output? = null,
public val outputLockingMode: Output? = null,
public val outputTimingSource: Output? = null,
public val supportLowFramerateInputs: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsGlobalConfigurationArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsGlobalConfigurationArgs.builder()
.initialAudioGain(initialAudioGain?.applyValue({ args0 -> args0 }))
.inputEndAction(inputEndAction?.applyValue({ args0 -> args0 }))
.inputLossBehavior(inputLossBehavior?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.outputLockingMode(outputLockingMode?.applyValue({ args0 -> args0 }))
.outputTimingSource(outputTimingSource?.applyValue({ args0 -> args0 }))
.supportLowFramerateInputs(supportLowFramerateInputs?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsGlobalConfigurationArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsGlobalConfigurationArgsBuilder internal constructor() {
private var initialAudioGain: Output? = null
private var inputEndAction: Output? = null
private var inputLossBehavior:
Output? = null
private var outputLockingMode: Output? = null
private var outputTimingSource: Output? = null
private var supportLowFramerateInputs: Output? = null
/**
* @param value Value to set the initial audio gain for the Live Event.
*/
@JvmName("dqjpihldlotqvlqk")
public suspend fun initialAudioGain(`value`: Output) {
this.initialAudioGain = value
}
/**
* @param value Indicates the action to take when the current input completes (e.g. end-of-file). When switchAndLoopInputs is configured the encoder will restart at the beginning of the first input. When “none” is configured the encoder will transcode either black, a solid color, or a user specified slate images per the “Input Loss Behavior” configuration until the next input switch occurs (which is controlled through the Channel Schedule API).
*/
@JvmName("ybotcvakxjhqtwtj")
public suspend fun inputEndAction(`value`: Output) {
this.inputEndAction = value
}
/**
* @param value Settings for system actions when input is lost. See Input Loss Behavior for more details.
*/
@JvmName("jadhkuwolnpjmtku")
public suspend fun inputLossBehavior(`value`: Output) {
this.inputLossBehavior = value
}
/**
* @param value Indicates how MediaLive pipelines are synchronized. PIPELINE\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the other. EPOCH\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the Unix epoch.
*/
@JvmName("lmkesrvghaufmtdo")
public suspend fun outputLockingMode(`value`: Output) {
this.outputLockingMode = value
}
/**
* @param value Indicates whether the rate of frames emitted by the Live encoder should be paced by its system clock (which optionally may be locked to another source via NTP) or should be locked to the clock of the source that is providing the input stream.
*/
@JvmName("bqjxtlnyedyxcfvp")
public suspend fun outputTimingSource(`value`: Output) {
this.outputTimingSource = value
}
/**
* @param value Adjusts video input buffer for streams with very low video framerates. This is commonly set to enabled for music channels with less than one video frame per second.
*/
@JvmName("ekxvurfpongtirgf")
public suspend fun supportLowFramerateInputs(`value`: Output) {
this.supportLowFramerateInputs = value
}
/**
* @param value Value to set the initial audio gain for the Live Event.
*/
@JvmName("srwqjqoradsularh")
public suspend fun initialAudioGain(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.initialAudioGain = mapped
}
/**
* @param value Indicates the action to take when the current input completes (e.g. end-of-file). When switchAndLoopInputs is configured the encoder will restart at the beginning of the first input. When “none” is configured the encoder will transcode either black, a solid color, or a user specified slate images per the “Input Loss Behavior” configuration until the next input switch occurs (which is controlled through the Channel Schedule API).
*/
@JvmName("xyfptlasumurygmk")
public suspend fun inputEndAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputEndAction = mapped
}
/**
* @param value Settings for system actions when input is lost. See Input Loss Behavior for more details.
*/
@JvmName("gcndcejvmeptbcfy")
public suspend fun inputLossBehavior(`value`: ChannelEncoderSettingsGlobalConfigurationInputLossBehaviorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputLossBehavior = mapped
}
/**
* @param argument Settings for system actions when input is lost. See Input Loss Behavior for more details.
*/
@JvmName("woucflrtqixpidbu")
public suspend fun inputLossBehavior(argument: suspend ChannelEncoderSettingsGlobalConfigurationInputLossBehaviorArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsGlobalConfigurationInputLossBehaviorArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.inputLossBehavior = mapped
}
/**
* @param value Indicates how MediaLive pipelines are synchronized. PIPELINE\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the other. EPOCH\_LOCKING - MediaLive will attempt to synchronize the output of each pipeline to the Unix epoch.
*/
@JvmName("rrkkfenasqrgdhyr")
public suspend fun outputLockingMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outputLockingMode = mapped
}
/**
* @param value Indicates whether the rate of frames emitted by the Live encoder should be paced by its system clock (which optionally may be locked to another source via NTP) or should be locked to the clock of the source that is providing the input stream.
*/
@JvmName("qlboeymgngcaasil")
public suspend fun outputTimingSource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.outputTimingSource = mapped
}
/**
* @param value Adjusts video input buffer for streams with very low video framerates. This is commonly set to enabled for music channels with less than one video frame per second.
*/
@JvmName("enmkmfvylbevskww")
public suspend fun supportLowFramerateInputs(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.supportLowFramerateInputs = mapped
}
internal fun build(): ChannelEncoderSettingsGlobalConfigurationArgs =
ChannelEncoderSettingsGlobalConfigurationArgs(
initialAudioGain = initialAudioGain,
inputEndAction = inputEndAction,
inputLossBehavior = inputLossBehavior,
outputLockingMode = outputLockingMode,
outputTimingSource = outputTimingSource,
supportLowFramerateInputs = supportLowFramerateInputs,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy