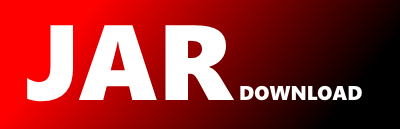
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property acquisitionPointId
* @property audioOnlyTimecodeControl
* @property certificateMode Setting to allow self signed or verified RTMP certificates.
* @property connectionRetryInterval Number of seconds to wait before retrying connection to the flash media server if the connection is lost.
* @property destination
* @property eventId
* @property eventIdMode
* @property eventStopBehavior
* @property filecacheDuration
* @property fragmentLength
* @property inputLossAction
* @property numRetries Number of retry attempts.
* @property restartDelay Number of seconds to wait until a restart is initiated.
* @property segmentationMode
* @property sendDelayMs
* @property sparseTrackType
* @property streamManifestBehavior
* @property timestampOffset
* @property timestampOffsetMode
*/
public data class ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs(
public val acquisitionPointId: Output? = null,
public val audioOnlyTimecodeControl: Output? = null,
public val certificateMode: Output? = null,
public val connectionRetryInterval: Output? = null,
public val destination: Output,
public val eventId: Output? = null,
public val eventIdMode: Output? = null,
public val eventStopBehavior: Output? = null,
public val filecacheDuration: Output? = null,
public val fragmentLength: Output? = null,
public val inputLossAction: Output? = null,
public val numRetries: Output? = null,
public val restartDelay: Output? = null,
public val segmentationMode: Output? = null,
public val sendDelayMs: Output? = null,
public val sparseTrackType: Output? = null,
public val streamManifestBehavior: Output? = null,
public val timestampOffset: Output? = null,
public val timestampOffsetMode: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs.builder()
.acquisitionPointId(acquisitionPointId?.applyValue({ args0 -> args0 }))
.audioOnlyTimecodeControl(audioOnlyTimecodeControl?.applyValue({ args0 -> args0 }))
.certificateMode(certificateMode?.applyValue({ args0 -> args0 }))
.connectionRetryInterval(connectionRetryInterval?.applyValue({ args0 -> args0 }))
.destination(destination.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.eventId(eventId?.applyValue({ args0 -> args0 }))
.eventIdMode(eventIdMode?.applyValue({ args0 -> args0 }))
.eventStopBehavior(eventStopBehavior?.applyValue({ args0 -> args0 }))
.filecacheDuration(filecacheDuration?.applyValue({ args0 -> args0 }))
.fragmentLength(fragmentLength?.applyValue({ args0 -> args0 }))
.inputLossAction(inputLossAction?.applyValue({ args0 -> args0 }))
.numRetries(numRetries?.applyValue({ args0 -> args0 }))
.restartDelay(restartDelay?.applyValue({ args0 -> args0 }))
.segmentationMode(segmentationMode?.applyValue({ args0 -> args0 }))
.sendDelayMs(sendDelayMs?.applyValue({ args0 -> args0 }))
.sparseTrackType(sparseTrackType?.applyValue({ args0 -> args0 }))
.streamManifestBehavior(streamManifestBehavior?.applyValue({ args0 -> args0 }))
.timestampOffset(timestampOffset?.applyValue({ args0 -> args0 }))
.timestampOffsetMode(timestampOffsetMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgsBuilder
internal constructor() {
private var acquisitionPointId: Output? = null
private var audioOnlyTimecodeControl: Output? = null
private var certificateMode: Output? = null
private var connectionRetryInterval: Output? = null
private var destination:
Output? =
null
private var eventId: Output? = null
private var eventIdMode: Output? = null
private var eventStopBehavior: Output? = null
private var filecacheDuration: Output? = null
private var fragmentLength: Output? = null
private var inputLossAction: Output? = null
private var numRetries: Output? = null
private var restartDelay: Output? = null
private var segmentationMode: Output? = null
private var sendDelayMs: Output? = null
private var sparseTrackType: Output? = null
private var streamManifestBehavior: Output? = null
private var timestampOffset: Output? = null
private var timestampOffsetMode: Output? = null
/**
* @param value
*/
@JvmName("kkolcflcavvpngpu")
public suspend fun acquisitionPointId(`value`: Output) {
this.acquisitionPointId = value
}
/**
* @param value
*/
@JvmName("hcccdsnkneyipaww")
public suspend fun audioOnlyTimecodeControl(`value`: Output) {
this.audioOnlyTimecodeControl = value
}
/**
* @param value Setting to allow self signed or verified RTMP certificates.
*/
@JvmName("crwxvukfrnycwjtk")
public suspend fun certificateMode(`value`: Output) {
this.certificateMode = value
}
/**
* @param value Number of seconds to wait before retrying connection to the flash media server if the connection is lost.
*/
@JvmName("lmnawpurvmufmwyr")
public suspend fun connectionRetryInterval(`value`: Output) {
this.connectionRetryInterval = value
}
/**
* @param value
*/
@JvmName("hlkkvxyfpiwhknnf")
public suspend fun destination(`value`: Output) {
this.destination = value
}
/**
* @param value
*/
@JvmName("uuuukqkfsgobkjde")
public suspend fun eventId(`value`: Output) {
this.eventId = value
}
/**
* @param value
*/
@JvmName("yvqotierrmmlfxnl")
public suspend fun eventIdMode(`value`: Output) {
this.eventIdMode = value
}
/**
* @param value
*/
@JvmName("piavjtanrfunjiho")
public suspend fun eventStopBehavior(`value`: Output) {
this.eventStopBehavior = value
}
/**
* @param value
*/
@JvmName("qyonsdskxtqalglo")
public suspend fun filecacheDuration(`value`: Output) {
this.filecacheDuration = value
}
/**
* @param value
*/
@JvmName("eiebsfgqxucdvkhe")
public suspend fun fragmentLength(`value`: Output) {
this.fragmentLength = value
}
/**
* @param value
*/
@JvmName("umdsankfcmtiywcd")
public suspend fun inputLossAction(`value`: Output) {
this.inputLossAction = value
}
/**
* @param value Number of retry attempts.
*/
@JvmName("hscranbcoxtdrayc")
public suspend fun numRetries(`value`: Output) {
this.numRetries = value
}
/**
* @param value Number of seconds to wait until a restart is initiated.
*/
@JvmName("ayhbnfncxolsfkxj")
public suspend fun restartDelay(`value`: Output) {
this.restartDelay = value
}
/**
* @param value
*/
@JvmName("prqanrfhaebfexur")
public suspend fun segmentationMode(`value`: Output) {
this.segmentationMode = value
}
/**
* @param value
*/
@JvmName("rowbiorffcbrjyky")
public suspend fun sendDelayMs(`value`: Output) {
this.sendDelayMs = value
}
/**
* @param value
*/
@JvmName("amywysovvddqwjuu")
public suspend fun sparseTrackType(`value`: Output) {
this.sparseTrackType = value
}
/**
* @param value
*/
@JvmName("vpqddwwwmindhdsg")
public suspend fun streamManifestBehavior(`value`: Output) {
this.streamManifestBehavior = value
}
/**
* @param value
*/
@JvmName("tloyqkjjofxifnfx")
public suspend fun timestampOffset(`value`: Output) {
this.timestampOffset = value
}
/**
* @param value
*/
@JvmName("ogyubicljjtdbutj")
public suspend fun timestampOffsetMode(`value`: Output) {
this.timestampOffsetMode = value
}
/**
* @param value
*/
@JvmName("vwphbhtinxknobyn")
public suspend fun acquisitionPointId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acquisitionPointId = mapped
}
/**
* @param value
*/
@JvmName("kywhtdkllajatvpm")
public suspend fun audioOnlyTimecodeControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.audioOnlyTimecodeControl = mapped
}
/**
* @param value Setting to allow self signed or verified RTMP certificates.
*/
@JvmName("hxsnvcwbojxhppcv")
public suspend fun certificateMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.certificateMode = mapped
}
/**
* @param value Number of seconds to wait before retrying connection to the flash media server if the connection is lost.
*/
@JvmName("lyljfkofudcxhevc")
public suspend fun connectionRetryInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionRetryInterval = mapped
}
/**
* @param value
*/
@JvmName("hxmpsryjvthbbvtu")
public suspend fun destination(`value`: ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsDestinationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.destination = mapped
}
/**
* @param argument
*/
@JvmName("hqhoynkawlrtwcje")
public suspend fun destination(argument: suspend ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsDestinationArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsDestinationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.destination = mapped
}
/**
* @param value
*/
@JvmName("eiwosqejrsjgqgcx")
public suspend fun eventId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventId = mapped
}
/**
* @param value
*/
@JvmName("wqjibrsolfmppjtp")
public suspend fun eventIdMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventIdMode = mapped
}
/**
* @param value
*/
@JvmName("dasmomdyagpainuh")
public suspend fun eventStopBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventStopBehavior = mapped
}
/**
* @param value
*/
@JvmName("xgngymbcxhifyxon")
public suspend fun filecacheDuration(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filecacheDuration = mapped
}
/**
* @param value
*/
@JvmName("ighfmaypknxeqofj")
public suspend fun fragmentLength(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fragmentLength = mapped
}
/**
* @param value
*/
@JvmName("ahrnsjufultpsmhn")
public suspend fun inputLossAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputLossAction = mapped
}
/**
* @param value Number of retry attempts.
*/
@JvmName("acnkrxuqgoalvbbh")
public suspend fun numRetries(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.numRetries = mapped
}
/**
* @param value Number of seconds to wait until a restart is initiated.
*/
@JvmName("hfasbvgtyvljxkii")
public suspend fun restartDelay(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.restartDelay = mapped
}
/**
* @param value
*/
@JvmName("shvpviixliffblae")
public suspend fun segmentationMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.segmentationMode = mapped
}
/**
* @param value
*/
@JvmName("dfoyqaewetjdyqmm")
public suspend fun sendDelayMs(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sendDelayMs = mapped
}
/**
* @param value
*/
@JvmName("jimxavxkdoqgoapb")
public suspend fun sparseTrackType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sparseTrackType = mapped
}
/**
* @param value
*/
@JvmName("apytneawayayblil")
public suspend fun streamManifestBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.streamManifestBehavior = mapped
}
/**
* @param value
*/
@JvmName("uekhnpflrgobxmyk")
public suspend fun timestampOffset(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timestampOffset = mapped
}
/**
* @param value
*/
@JvmName("xtjefspnrhwguoaf")
public suspend fun timestampOffsetMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timestampOffsetMode = mapped
}
internal fun build(): ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs =
ChannelEncoderSettingsOutputGroupOutputGroupSettingsMsSmoothGroupSettingsArgs(
acquisitionPointId = acquisitionPointId,
audioOnlyTimecodeControl = audioOnlyTimecodeControl,
certificateMode = certificateMode,
connectionRetryInterval = connectionRetryInterval,
destination = destination ?: throw PulumiNullFieldException("destination"),
eventId = eventId,
eventIdMode = eventIdMode,
eventStopBehavior = eventStopBehavior,
filecacheDuration = filecacheDuration,
fragmentLength = fragmentLength,
inputLossAction = inputLossAction,
numRetries = numRetries,
restartDelay = restartDelay,
segmentationMode = segmentationMode,
sendDelayMs = sendDelayMs,
sparseTrackType = sparseTrackType,
streamManifestBehavior = streamManifestBehavior,
timestampOffset = timestampOffset,
timestampOffsetMode = timestampOffsetMode,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy