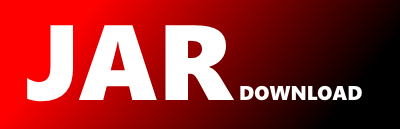
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsVideoDescriptionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property codecSettings The video codec settings. See Video Codec Settings for more details.
* @property height Output video height in pixels.
* @property name The name of the video description.
* @property respondToAfd Indicate how to respond to the AFD values that might be in the input video.
* @property scalingBehavior Behavior on how to scale.
* @property sharpness Changes the strength of the anti-alias filter used for scaling.
* @property width Output video width in pixels.
*/
public data class ChannelEncoderSettingsVideoDescriptionArgs(
public val codecSettings: Output? = null,
public val height: Output? = null,
public val name: Output,
public val respondToAfd: Output? = null,
public val scalingBehavior: Output? = null,
public val sharpness: Output? = null,
public val width: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionArgs = com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionArgs.builder()
.codecSettings(codecSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.height(height?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.respondToAfd(respondToAfd?.applyValue({ args0 -> args0 }))
.scalingBehavior(scalingBehavior?.applyValue({ args0 -> args0 }))
.sharpness(sharpness?.applyValue({ args0 -> args0 }))
.width(width?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsVideoDescriptionArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsVideoDescriptionArgsBuilder internal constructor() {
private var codecSettings: Output? = null
private var height: Output? = null
private var name: Output? = null
private var respondToAfd: Output? = null
private var scalingBehavior: Output? = null
private var sharpness: Output? = null
private var width: Output? = null
/**
* @param value The video codec settings. See Video Codec Settings for more details.
*/
@JvmName("ipsnxftebqcyjsym")
public suspend fun codecSettings(`value`: Output) {
this.codecSettings = value
}
/**
* @param value Output video height in pixels.
*/
@JvmName("exxaqxxbswqpbxds")
public suspend fun height(`value`: Output) {
this.height = value
}
/**
* @param value The name of the video description.
*/
@JvmName("abocxkaeeyeueaad")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Indicate how to respond to the AFD values that might be in the input video.
*/
@JvmName("abweuamaxvccyahw")
public suspend fun respondToAfd(`value`: Output) {
this.respondToAfd = value
}
/**
* @param value Behavior on how to scale.
*/
@JvmName("jkqkvjvbxdlefftq")
public suspend fun scalingBehavior(`value`: Output) {
this.scalingBehavior = value
}
/**
* @param value Changes the strength of the anti-alias filter used for scaling.
*/
@JvmName("xlydyiohqhwctcgf")
public suspend fun sharpness(`value`: Output) {
this.sharpness = value
}
/**
* @param value Output video width in pixels.
*/
@JvmName("lcodjoddrblfuhae")
public suspend fun width(`value`: Output) {
this.width = value
}
/**
* @param value The video codec settings. See Video Codec Settings for more details.
*/
@JvmName("xdxsvxmjutgyudgh")
public suspend fun codecSettings(`value`: ChannelEncoderSettingsVideoDescriptionCodecSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.codecSettings = mapped
}
/**
* @param argument The video codec settings. See Video Codec Settings for more details.
*/
@JvmName("fpjandghfampsnii")
public suspend fun codecSettings(argument: suspend ChannelEncoderSettingsVideoDescriptionCodecSettingsArgsBuilder.() -> Unit) {
val toBeMapped = ChannelEncoderSettingsVideoDescriptionCodecSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.codecSettings = mapped
}
/**
* @param value Output video height in pixels.
*/
@JvmName("bdsiiwhpwjorvlno")
public suspend fun height(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.height = mapped
}
/**
* @param value The name of the video description.
*/
@JvmName("mtlbrtqstdmkmnho")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Indicate how to respond to the AFD values that might be in the input video.
*/
@JvmName("eahpysnhliveottc")
public suspend fun respondToAfd(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.respondToAfd = mapped
}
/**
* @param value Behavior on how to scale.
*/
@JvmName("bfpsmaurmuyynytu")
public suspend fun scalingBehavior(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scalingBehavior = mapped
}
/**
* @param value Changes the strength of the anti-alias filter used for scaling.
*/
@JvmName("fvansfvlfgtctwct")
public suspend fun sharpness(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharpness = mapped
}
/**
* @param value Output video width in pixels.
*/
@JvmName("lxnahktdkkbfxoro")
public suspend fun width(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.width = mapped
}
internal fun build(): ChannelEncoderSettingsVideoDescriptionArgs =
ChannelEncoderSettingsVideoDescriptionArgs(
codecSettings = codecSettings,
height = height,
name = name ?: throw PulumiNullFieldException("name"),
respondToAfd = respondToAfd,
scalingBehavior = scalingBehavior,
sharpness = sharpness,
width = width,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy