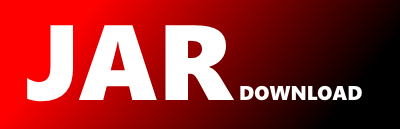
com.pulumi.aws.medialive.kotlin.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property adaptiveQuantization Enables or disables adaptive quantization.
* @property afdSignaling Indicates that AFD values will be written into the output stream.
* @property alternativeTransferFunction Whether or not EML should insert an Alternative Transfer Function SEI message.
* @property bitrate Average bitrate in bits/second.
* @property bufSize Size of buffer in bits.
* @property colorMetadata Includes color space metadata in the output.
* @property colorSpaceSettings Define the color metadata for the output. H265 Color Space Settings for more details.
* @property filterSettings Filters to apply to an encode. See H265 Filter Settings for more details.
* @property fixedAfd Four bit AFD value to write on all frames of video in the output stream.
* @property flickerAq
* @property framerateDenominator Framerate denominator.
* @property framerateNumerator Framerate numerator.
* @property gopClosedCadence Frequency of closed GOPs.
* @property gopSize GOP size in units of either frames of seconds per `gop_size_units`.
* @property gopSizeUnits Indicates if the `gop_size` is specified in frames or seconds.
* @property level H265 level.
* @property lookAheadRateControl Amount of lookahead.
* @property maxBitrate Set the maximum bitrate in order to accommodate expected spikes in the complexity of the video.
* @property minIInterval Min interval.
* @property minQp Set the minimum QP.
* @property mvOverPictureBoundaries Enables or disables motion vector over picture boundaries.
* @property mvTemporalPredictor Enables or disables the motion vector temporal predictor.
* @property parDenominator Pixel Aspect Ratio denominator.
* @property parNumerator Pixel Aspect Ratio numerator.
* @property profile H265 profile.
* @property qvbrQualityLevel Controls the target quality for the video encode.
* @property rateControlMode Rate control mode.
* @property scanType Sets the scan type of the output.
* @property sceneChangeDetect Scene change detection.
* @property slices Number of slices per picture.
* @property tier Set the H265 tier in the output.
* @property tileHeight Sets the height of tiles.
* @property tilePadding Enables or disables padding of tiles.
* @property tileWidth Sets the width of tiles.
* @property timecodeBurninSettings Apply a burned in timecode. See H265 Timecode Burnin Settings for more details.
* @property timecodeInsertion Determines how timecodes should be inserted into the video elementary stream.
* @property treeblockSize Sets the size of the treeblock.
*/
public data class ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs(
public val adaptiveQuantization: Output? = null,
public val afdSignaling: Output? = null,
public val alternativeTransferFunction: Output? = null,
public val bitrate: Output,
public val bufSize: Output? = null,
public val colorMetadata: Output? = null,
public val colorSpaceSettings: Output? =
null,
public val filterSettings: Output? =
null,
public val fixedAfd: Output? = null,
public val flickerAq: Output? = null,
public val framerateDenominator: Output,
public val framerateNumerator: Output,
public val gopClosedCadence: Output? = null,
public val gopSize: Output? = null,
public val gopSizeUnits: Output? = null,
public val level: Output? = null,
public val lookAheadRateControl: Output? = null,
public val maxBitrate: Output? = null,
public val minIInterval: Output? = null,
public val minQp: Output? = null,
public val mvOverPictureBoundaries: Output? = null,
public val mvTemporalPredictor: Output? = null,
public val parDenominator: Output? = null,
public val parNumerator: Output? = null,
public val profile: Output? = null,
public val qvbrQualityLevel: Output? = null,
public val rateControlMode: Output? = null,
public val scanType: Output? = null,
public val sceneChangeDetect: Output? = null,
public val slices: Output? = null,
public val tier: Output? = null,
public val tileHeight: Output? = null,
public val tilePadding: Output? = null,
public val tileWidth: Output? = null,
public val timecodeBurninSettings: Output? =
null,
public val timecodeInsertion: Output? = null,
public val treeblockSize: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs.builder()
.adaptiveQuantization(adaptiveQuantization?.applyValue({ args0 -> args0 }))
.afdSignaling(afdSignaling?.applyValue({ args0 -> args0 }))
.alternativeTransferFunction(alternativeTransferFunction?.applyValue({ args0 -> args0 }))
.bitrate(bitrate.applyValue({ args0 -> args0 }))
.bufSize(bufSize?.applyValue({ args0 -> args0 }))
.colorMetadata(colorMetadata?.applyValue({ args0 -> args0 }))
.colorSpaceSettings(
colorSpaceSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.filterSettings(filterSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fixedAfd(fixedAfd?.applyValue({ args0 -> args0 }))
.flickerAq(flickerAq?.applyValue({ args0 -> args0 }))
.framerateDenominator(framerateDenominator.applyValue({ args0 -> args0 }))
.framerateNumerator(framerateNumerator.applyValue({ args0 -> args0 }))
.gopClosedCadence(gopClosedCadence?.applyValue({ args0 -> args0 }))
.gopSize(gopSize?.applyValue({ args0 -> args0 }))
.gopSizeUnits(gopSizeUnits?.applyValue({ args0 -> args0 }))
.level(level?.applyValue({ args0 -> args0 }))
.lookAheadRateControl(lookAheadRateControl?.applyValue({ args0 -> args0 }))
.maxBitrate(maxBitrate?.applyValue({ args0 -> args0 }))
.minIInterval(minIInterval?.applyValue({ args0 -> args0 }))
.minQp(minQp?.applyValue({ args0 -> args0 }))
.mvOverPictureBoundaries(mvOverPictureBoundaries?.applyValue({ args0 -> args0 }))
.mvTemporalPredictor(mvTemporalPredictor?.applyValue({ args0 -> args0 }))
.parDenominator(parDenominator?.applyValue({ args0 -> args0 }))
.parNumerator(parNumerator?.applyValue({ args0 -> args0 }))
.profile(profile?.applyValue({ args0 -> args0 }))
.qvbrQualityLevel(qvbrQualityLevel?.applyValue({ args0 -> args0 }))
.rateControlMode(rateControlMode?.applyValue({ args0 -> args0 }))
.scanType(scanType?.applyValue({ args0 -> args0 }))
.sceneChangeDetect(sceneChangeDetect?.applyValue({ args0 -> args0 }))
.slices(slices?.applyValue({ args0 -> args0 }))
.tier(tier?.applyValue({ args0 -> args0 }))
.tileHeight(tileHeight?.applyValue({ args0 -> args0 }))
.tilePadding(tilePadding?.applyValue({ args0 -> args0 }))
.tileWidth(tileWidth?.applyValue({ args0 -> args0 }))
.timecodeBurninSettings(
timecodeBurninSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.timecodeInsertion(timecodeInsertion?.applyValue({ args0 -> args0 }))
.treeblockSize(treeblockSize?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs].
*/
@PulumiTagMarker
public class ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgsBuilder internal constructor() {
private var adaptiveQuantization: Output? = null
private var afdSignaling: Output? = null
private var alternativeTransferFunction: Output? = null
private var bitrate: Output? = null
private var bufSize: Output? = null
private var colorMetadata: Output? = null
private var colorSpaceSettings:
Output? =
null
private var filterSettings:
Output? =
null
private var fixedAfd: Output? = null
private var flickerAq: Output? = null
private var framerateDenominator: Output? = null
private var framerateNumerator: Output? = null
private var gopClosedCadence: Output? = null
private var gopSize: Output? = null
private var gopSizeUnits: Output? = null
private var level: Output? = null
private var lookAheadRateControl: Output? = null
private var maxBitrate: Output? = null
private var minIInterval: Output? = null
private var minQp: Output? = null
private var mvOverPictureBoundaries: Output? = null
private var mvTemporalPredictor: Output? = null
private var parDenominator: Output? = null
private var parNumerator: Output? = null
private var profile: Output? = null
private var qvbrQualityLevel: Output? = null
private var rateControlMode: Output? = null
private var scanType: Output? = null
private var sceneChangeDetect: Output? = null
private var slices: Output? = null
private var tier: Output? = null
private var tileHeight: Output? = null
private var tilePadding: Output? = null
private var tileWidth: Output? = null
private var timecodeBurninSettings:
Output? =
null
private var timecodeInsertion: Output? = null
private var treeblockSize: Output? = null
/**
* @param value Enables or disables adaptive quantization.
*/
@JvmName("exywnvxcyscorwvh")
public suspend fun adaptiveQuantization(`value`: Output) {
this.adaptiveQuantization = value
}
/**
* @param value Indicates that AFD values will be written into the output stream.
*/
@JvmName("jbvqrycwhhxrhuaw")
public suspend fun afdSignaling(`value`: Output) {
this.afdSignaling = value
}
/**
* @param value Whether or not EML should insert an Alternative Transfer Function SEI message.
*/
@JvmName("ytssvbmuibrmuxnd")
public suspend fun alternativeTransferFunction(`value`: Output) {
this.alternativeTransferFunction = value
}
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("oavfwnuewchpdgbc")
public suspend fun bitrate(`value`: Output) {
this.bitrate = value
}
/**
* @param value Size of buffer in bits.
*/
@JvmName("rpiswvbybgyrqvdg")
public suspend fun bufSize(`value`: Output) {
this.bufSize = value
}
/**
* @param value Includes color space metadata in the output.
*/
@JvmName("rpvpuerxqueqpypm")
public suspend fun colorMetadata(`value`: Output) {
this.colorMetadata = value
}
/**
* @param value Define the color metadata for the output. H265 Color Space Settings for more details.
*/
@JvmName("budvjrwdsiruhhco")
public suspend fun colorSpaceSettings(`value`: Output) {
this.colorSpaceSettings = value
}
/**
* @param value Filters to apply to an encode. See H265 Filter Settings for more details.
*/
@JvmName("fqfaqbfallvevtrc")
public suspend fun filterSettings(`value`: Output) {
this.filterSettings = value
}
/**
* @param value Four bit AFD value to write on all frames of video in the output stream.
*/
@JvmName("kagyxjpahsgofbry")
public suspend fun fixedAfd(`value`: Output) {
this.fixedAfd = value
}
/**
* @param value
*/
@JvmName("bwlpycklpurunbwe")
public suspend fun flickerAq(`value`: Output) {
this.flickerAq = value
}
/**
* @param value Framerate denominator.
*/
@JvmName("vguywkkkppcjmxfw")
public suspend fun framerateDenominator(`value`: Output) {
this.framerateDenominator = value
}
/**
* @param value Framerate numerator.
*/
@JvmName("tbrbcahgahbpnfen")
public suspend fun framerateNumerator(`value`: Output) {
this.framerateNumerator = value
}
/**
* @param value Frequency of closed GOPs.
*/
@JvmName("cqtjmfxlayehfqeb")
public suspend fun gopClosedCadence(`value`: Output) {
this.gopClosedCadence = value
}
/**
* @param value GOP size in units of either frames of seconds per `gop_size_units`.
*/
@JvmName("qvfwfbiuxambgrwt")
public suspend fun gopSize(`value`: Output) {
this.gopSize = value
}
/**
* @param value Indicates if the `gop_size` is specified in frames or seconds.
*/
@JvmName("filumvmcghqcnhcf")
public suspend fun gopSizeUnits(`value`: Output) {
this.gopSizeUnits = value
}
/**
* @param value H265 level.
*/
@JvmName("icnscdmxuaqhmmjt")
public suspend fun level(`value`: Output) {
this.level = value
}
/**
* @param value Amount of lookahead.
*/
@JvmName("uxkbuakkfrrjcxtt")
public suspend fun lookAheadRateControl(`value`: Output) {
this.lookAheadRateControl = value
}
/**
* @param value Set the maximum bitrate in order to accommodate expected spikes in the complexity of the video.
*/
@JvmName("biddniwixwdfupkk")
public suspend fun maxBitrate(`value`: Output) {
this.maxBitrate = value
}
/**
* @param value Min interval.
*/
@JvmName("yquqycrodaefqvvc")
public suspend fun minIInterval(`value`: Output) {
this.minIInterval = value
}
/**
* @param value Set the minimum QP.
*/
@JvmName("lsrtievtlmfamobh")
public suspend fun minQp(`value`: Output) {
this.minQp = value
}
/**
* @param value Enables or disables motion vector over picture boundaries.
*/
@JvmName("tfpohyfnghnwnclq")
public suspend fun mvOverPictureBoundaries(`value`: Output) {
this.mvOverPictureBoundaries = value
}
/**
* @param value Enables or disables the motion vector temporal predictor.
*/
@JvmName("btdnowyjwxsepkcs")
public suspend fun mvTemporalPredictor(`value`: Output) {
this.mvTemporalPredictor = value
}
/**
* @param value Pixel Aspect Ratio denominator.
*/
@JvmName("cinvuxtvcktbuvig")
public suspend fun parDenominator(`value`: Output) {
this.parDenominator = value
}
/**
* @param value Pixel Aspect Ratio numerator.
*/
@JvmName("leofxfaufobasrfh")
public suspend fun parNumerator(`value`: Output) {
this.parNumerator = value
}
/**
* @param value H265 profile.
*/
@JvmName("skusnwayncupvafj")
public suspend fun profile(`value`: Output) {
this.profile = value
}
/**
* @param value Controls the target quality for the video encode.
*/
@JvmName("igpopvsgolddrnnv")
public suspend fun qvbrQualityLevel(`value`: Output) {
this.qvbrQualityLevel = value
}
/**
* @param value Rate control mode.
*/
@JvmName("kfdcagfqsywddysl")
public suspend fun rateControlMode(`value`: Output) {
this.rateControlMode = value
}
/**
* @param value Sets the scan type of the output.
*/
@JvmName("neuafiqxolfkkxwo")
public suspend fun scanType(`value`: Output) {
this.scanType = value
}
/**
* @param value Scene change detection.
*/
@JvmName("lwojpjdlkbystvrk")
public suspend fun sceneChangeDetect(`value`: Output) {
this.sceneChangeDetect = value
}
/**
* @param value Number of slices per picture.
*/
@JvmName("iermkugmtagajacm")
public suspend fun slices(`value`: Output) {
this.slices = value
}
/**
* @param value Set the H265 tier in the output.
*/
@JvmName("nqnbruooufhxjcee")
public suspend fun tier(`value`: Output) {
this.tier = value
}
/**
* @param value Sets the height of tiles.
*/
@JvmName("fkpjqdxydvwmsgdv")
public suspend fun tileHeight(`value`: Output) {
this.tileHeight = value
}
/**
* @param value Enables or disables padding of tiles.
*/
@JvmName("excwnddjdiqrrdkv")
public suspend fun tilePadding(`value`: Output) {
this.tilePadding = value
}
/**
* @param value Sets the width of tiles.
*/
@JvmName("kywnlxcdjgptrysv")
public suspend fun tileWidth(`value`: Output) {
this.tileWidth = value
}
/**
* @param value Apply a burned in timecode. See H265 Timecode Burnin Settings for more details.
*/
@JvmName("mfauubkyuilngsic")
public suspend fun timecodeBurninSettings(`value`: Output) {
this.timecodeBurninSettings = value
}
/**
* @param value Determines how timecodes should be inserted into the video elementary stream.
*/
@JvmName("wqnwubvdmonsianl")
public suspend fun timecodeInsertion(`value`: Output) {
this.timecodeInsertion = value
}
/**
* @param value Sets the size of the treeblock.
*/
@JvmName("gsermwoagcmbrlje")
public suspend fun treeblockSize(`value`: Output) {
this.treeblockSize = value
}
/**
* @param value Enables or disables adaptive quantization.
*/
@JvmName("qwlodteenqptqdif")
public suspend fun adaptiveQuantization(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.adaptiveQuantization = mapped
}
/**
* @param value Indicates that AFD values will be written into the output stream.
*/
@JvmName("odqrqjbfdfdcbwlf")
public suspend fun afdSignaling(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.afdSignaling = mapped
}
/**
* @param value Whether or not EML should insert an Alternative Transfer Function SEI message.
*/
@JvmName("qiwxqblejuqtjkee")
public suspend fun alternativeTransferFunction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alternativeTransferFunction = mapped
}
/**
* @param value Average bitrate in bits/second.
*/
@JvmName("fnckstcqtrgtplat")
public suspend fun bitrate(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bitrate = mapped
}
/**
* @param value Size of buffer in bits.
*/
@JvmName("sojssixtpbljdlqr")
public suspend fun bufSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bufSize = mapped
}
/**
* @param value Includes color space metadata in the output.
*/
@JvmName("qikkihrcnjntyhna")
public suspend fun colorMetadata(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.colorMetadata = mapped
}
/**
* @param value Define the color metadata for the output. H265 Color Space Settings for more details.
*/
@JvmName("kfaysolmavqcujwt")
public suspend fun colorSpaceSettings(`value`: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsColorSpaceSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.colorSpaceSettings = mapped
}
/**
* @param argument Define the color metadata for the output. H265 Color Space Settings for more details.
*/
@JvmName("oirifxjnbppigqro")
public suspend fun colorSpaceSettings(argument: suspend ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsColorSpaceSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsColorSpaceSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.colorSpaceSettings = mapped
}
/**
* @param value Filters to apply to an encode. See H265 Filter Settings for more details.
*/
@JvmName("irpqqnooefcrckiu")
public suspend fun filterSettings(`value`: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsFilterSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filterSettings = mapped
}
/**
* @param argument Filters to apply to an encode. See H265 Filter Settings for more details.
*/
@JvmName("ylckqvvjxfuquhfd")
public suspend fun filterSettings(argument: suspend ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsFilterSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsFilterSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.filterSettings = mapped
}
/**
* @param value Four bit AFD value to write on all frames of video in the output stream.
*/
@JvmName("enqnoanracgcuxrf")
public suspend fun fixedAfd(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fixedAfd = mapped
}
/**
* @param value
*/
@JvmName("ijuvphdnlugdkchx")
public suspend fun flickerAq(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.flickerAq = mapped
}
/**
* @param value Framerate denominator.
*/
@JvmName("jlfjmsjkbgyevday")
public suspend fun framerateDenominator(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.framerateDenominator = mapped
}
/**
* @param value Framerate numerator.
*/
@JvmName("ynggbyenbqokmhkf")
public suspend fun framerateNumerator(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.framerateNumerator = mapped
}
/**
* @param value Frequency of closed GOPs.
*/
@JvmName("rtkxqaiybktarjnd")
public suspend fun gopClosedCadence(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gopClosedCadence = mapped
}
/**
* @param value GOP size in units of either frames of seconds per `gop_size_units`.
*/
@JvmName("gypgyfjkdmaspwrj")
public suspend fun gopSize(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gopSize = mapped
}
/**
* @param value Indicates if the `gop_size` is specified in frames or seconds.
*/
@JvmName("rnsegwvasrmfpymo")
public suspend fun gopSizeUnits(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gopSizeUnits = mapped
}
/**
* @param value H265 level.
*/
@JvmName("rjsjochicdyedagl")
public suspend fun level(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.level = mapped
}
/**
* @param value Amount of lookahead.
*/
@JvmName("tyvswygisbxdcwao")
public suspend fun lookAheadRateControl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lookAheadRateControl = mapped
}
/**
* @param value Set the maximum bitrate in order to accommodate expected spikes in the complexity of the video.
*/
@JvmName("qxdqhgwpdgsduroy")
public suspend fun maxBitrate(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxBitrate = mapped
}
/**
* @param value Min interval.
*/
@JvmName("vdhfcnljgdcsbxau")
public suspend fun minIInterval(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minIInterval = mapped
}
/**
* @param value Set the minimum QP.
*/
@JvmName("ovepacagchtqtugo")
public suspend fun minQp(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minQp = mapped
}
/**
* @param value Enables or disables motion vector over picture boundaries.
*/
@JvmName("iubfddboogjhitpc")
public suspend fun mvOverPictureBoundaries(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mvOverPictureBoundaries = mapped
}
/**
* @param value Enables or disables the motion vector temporal predictor.
*/
@JvmName("jhlrmjuhbfeqeepc")
public suspend fun mvTemporalPredictor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mvTemporalPredictor = mapped
}
/**
* @param value Pixel Aspect Ratio denominator.
*/
@JvmName("ckblnqtlppuoagge")
public suspend fun parDenominator(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parDenominator = mapped
}
/**
* @param value Pixel Aspect Ratio numerator.
*/
@JvmName("xuiomusykhpyfpyq")
public suspend fun parNumerator(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.parNumerator = mapped
}
/**
* @param value H265 profile.
*/
@JvmName("setwldusqkwwdeot")
public suspend fun profile(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.profile = mapped
}
/**
* @param value Controls the target quality for the video encode.
*/
@JvmName("itpjypjyrhdhuhub")
public suspend fun qvbrQualityLevel(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.qvbrQualityLevel = mapped
}
/**
* @param value Rate control mode.
*/
@JvmName("asxuthyvigwwwkqx")
public suspend fun rateControlMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rateControlMode = mapped
}
/**
* @param value Sets the scan type of the output.
*/
@JvmName("kbmcdbcnubltbotg")
public suspend fun scanType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scanType = mapped
}
/**
* @param value Scene change detection.
*/
@JvmName("ghctlgqinprojipa")
public suspend fun sceneChangeDetect(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sceneChangeDetect = mapped
}
/**
* @param value Number of slices per picture.
*/
@JvmName("drjcextkvyshrhav")
public suspend fun slices(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.slices = mapped
}
/**
* @param value Set the H265 tier in the output.
*/
@JvmName("wmixnvqtcawfaowb")
public suspend fun tier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tier = mapped
}
/**
* @param value Sets the height of tiles.
*/
@JvmName("pnljtdnrnnkkyiuw")
public suspend fun tileHeight(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tileHeight = mapped
}
/**
* @param value Enables or disables padding of tiles.
*/
@JvmName("mdggsklujxohhait")
public suspend fun tilePadding(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tilePadding = mapped
}
/**
* @param value Sets the width of tiles.
*/
@JvmName("fmmbxyydhsrqlbsm")
public suspend fun tileWidth(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tileWidth = mapped
}
/**
* @param value Apply a burned in timecode. See H265 Timecode Burnin Settings for more details.
*/
@JvmName("xdjxibofuepljdyv")
public suspend fun timecodeBurninSettings(`value`: ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsTimecodeBurninSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timecodeBurninSettings = mapped
}
/**
* @param argument Apply a burned in timecode. See H265 Timecode Burnin Settings for more details.
*/
@JvmName("gnhmiwgxrehglqiq")
public suspend fun timecodeBurninSettings(argument: suspend ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsTimecodeBurninSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsTimecodeBurninSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.timecodeBurninSettings = mapped
}
/**
* @param value Determines how timecodes should be inserted into the video elementary stream.
*/
@JvmName("nipletutwfhcjmff")
public suspend fun timecodeInsertion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timecodeInsertion = mapped
}
/**
* @param value Sets the size of the treeblock.
*/
@JvmName("ghicxjugrdxjyrpd")
public suspend fun treeblockSize(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.treeblockSize = mapped
}
internal fun build(): ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs =
ChannelEncoderSettingsVideoDescriptionCodecSettingsH265SettingsArgs(
adaptiveQuantization = adaptiveQuantization,
afdSignaling = afdSignaling,
alternativeTransferFunction = alternativeTransferFunction,
bitrate = bitrate ?: throw PulumiNullFieldException("bitrate"),
bufSize = bufSize,
colorMetadata = colorMetadata,
colorSpaceSettings = colorSpaceSettings,
filterSettings = filterSettings,
fixedAfd = fixedAfd,
flickerAq = flickerAq,
framerateDenominator = framerateDenominator ?: throw
PulumiNullFieldException("framerateDenominator"),
framerateNumerator = framerateNumerator ?: throw PulumiNullFieldException("framerateNumerator"),
gopClosedCadence = gopClosedCadence,
gopSize = gopSize,
gopSizeUnits = gopSizeUnits,
level = level,
lookAheadRateControl = lookAheadRateControl,
maxBitrate = maxBitrate,
minIInterval = minIInterval,
minQp = minQp,
mvOverPictureBoundaries = mvOverPictureBoundaries,
mvTemporalPredictor = mvTemporalPredictor,
parDenominator = parDenominator,
parNumerator = parNumerator,
profile = profile,
qvbrQualityLevel = qvbrQualityLevel,
rateControlMode = rateControlMode,
scanType = scanType,
sceneChangeDetect = sceneChangeDetect,
slices = slices,
tier = tier,
tileHeight = tileHeight,
tilePadding = tilePadding,
tileWidth = tileWidth,
timecodeBurninSettings = timecodeBurninSettings,
timecodeInsertion = timecodeInsertion,
treeblockSize = treeblockSize,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy