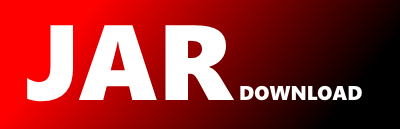
com.pulumi.aws.medialive.kotlin.inputs.ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.inputs
import com.pulumi.aws.medialive.inputs.ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property audioSilenceSettings MediaLive will perform a failover if the specified audio selector is silent for the specified period. See Audio Silence Failover Settings for more details.
* @property inputLossSettings MediaLive will perform a failover if content is not detected in this input for the specified period. See Input Loss Failover Settings for more details.
* @property videoBlackSettings MediaLive will perform a failover if content is considered black for the specified period. See Video Black Failover Settings for more details.
*/
public data class
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs(
public val audioSilenceSettings: Output? =
null,
public val inputLossSettings: Output? =
null,
public val videoBlackSettings: Output? =
null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.aws.medialive.inputs.ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs =
com.pulumi.aws.medialive.inputs.ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs.builder()
.audioSilenceSettings(
audioSilenceSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.inputLossSettings(inputLossSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.videoBlackSettings(
videoBlackSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs].
*/
@PulumiTagMarker
public class
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgsBuilder
internal constructor() {
private var audioSilenceSettings:
Output? =
null
private var inputLossSettings:
Output? =
null
private var videoBlackSettings:
Output? =
null
/**
* @param value MediaLive will perform a failover if the specified audio selector is silent for the specified period. See Audio Silence Failover Settings for more details.
*/
@JvmName("cseiveccikxyhhmt")
public suspend fun audioSilenceSettings(`value`: Output) {
this.audioSilenceSettings = value
}
/**
* @param value MediaLive will perform a failover if content is not detected in this input for the specified period. See Input Loss Failover Settings for more details.
*/
@JvmName("hgqjqmxavpmiyysl")
public suspend fun inputLossSettings(`value`: Output) {
this.inputLossSettings = value
}
/**
* @param value MediaLive will perform a failover if content is considered black for the specified period. See Video Black Failover Settings for more details.
*/
@JvmName("gceftqtsnojkmybf")
public suspend fun videoBlackSettings(`value`: Output) {
this.videoBlackSettings = value
}
/**
* @param value MediaLive will perform a failover if the specified audio selector is silent for the specified period. See Audio Silence Failover Settings for more details.
*/
@JvmName("hypddvaxrxkfalfw")
public suspend fun audioSilenceSettings(`value`: ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsAudioSilenceSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.audioSilenceSettings = mapped
}
/**
* @param argument MediaLive will perform a failover if the specified audio selector is silent for the specified period. See Audio Silence Failover Settings for more details.
*/
@JvmName("ekulekodbrbdbeed")
public suspend fun audioSilenceSettings(argument: suspend ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsAudioSilenceSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsAudioSilenceSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.audioSilenceSettings = mapped
}
/**
* @param value MediaLive will perform a failover if content is not detected in this input for the specified period. See Input Loss Failover Settings for more details.
*/
@JvmName("ufyhxejmtnogpxdn")
public suspend fun inputLossSettings(`value`: ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsInputLossSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.inputLossSettings = mapped
}
/**
* @param argument MediaLive will perform a failover if content is not detected in this input for the specified period. See Input Loss Failover Settings for more details.
*/
@JvmName("kppkynxjkffdyari")
public suspend fun inputLossSettings(argument: suspend ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsInputLossSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsInputLossSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.inputLossSettings = mapped
}
/**
* @param value MediaLive will perform a failover if content is considered black for the specified period. See Video Black Failover Settings for more details.
*/
@JvmName("rodwvrkhgrkbdfck")
public suspend fun videoBlackSettings(`value`: ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsVideoBlackSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.videoBlackSettings = mapped
}
/**
* @param argument MediaLive will perform a failover if content is considered black for the specified period. See Video Black Failover Settings for more details.
*/
@JvmName("aubnmnrjoffmtxvi")
public suspend fun videoBlackSettings(argument: suspend ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsVideoBlackSettingsArgsBuilder.() -> Unit) {
val toBeMapped =
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsVideoBlackSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.videoBlackSettings = mapped
}
internal fun build(): ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs =
ChannelInputAttachmentAutomaticInputFailoverSettingsFailoverConditionFailoverConditionSettingsArgs(
audioSilenceSettings = audioSilenceSettings,
inputLossSettings = inputLossSettings,
videoBlackSettings = videoBlackSettings,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy