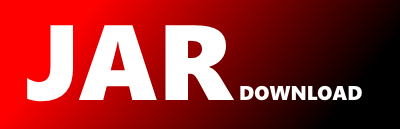
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property adMarkers The ad marker type for this output group.
* @property baseUrlContent
* @property baseUrlContent1
* @property baseUrlManifest
* @property baseUrlManifest1
* @property captionLanguageMappings
* @property captionLanguageSetting
* @property clientCache
* @property codecSpecification
* @property constantIv
* @property destination
* @property directoryStructure
* @property discontinuityTags
* @property encryptionType
* @property hlsCdnSettings
* @property hlsId3SegmentTagging
* @property iframeOnlyPlaylists
* @property incompleteSegmentBehavior
* @property indexNSegments
* @property inputLossAction
* @property ivInManifest
* @property ivSource
* @property keepSegments
* @property keyFormat
* @property keyFormatVersions
* @property keyProviderSettings
* @property manifestCompression
* @property manifestDurationFormat
* @property minSegmentLength
* @property mode
* @property outputSelection
* @property programDateTime
* @property programDateTimeClock
* @property programDateTimePeriod
* @property redundantManifest
* @property segmentLength
* @property segmentsPerSubdirectory
* @property streamInfResolution
* @property timedMetadataId3Frame Indicates ID3 frame that has the timecode.
* @property timedMetadataId3Period
* @property timestampDeltaMilliseconds
* @property tsFileMode
*/
public data class ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings(
public val adMarkers: List? = null,
public val baseUrlContent: String? = null,
public val baseUrlContent1: String? = null,
public val baseUrlManifest: String? = null,
public val baseUrlManifest1: String? = null,
public val captionLanguageMappings: List? =
null,
public val captionLanguageSetting: String? = null,
public val clientCache: String? = null,
public val codecSpecification: String? = null,
public val constantIv: String? = null,
public val destination: ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination,
public val directoryStructure: String? = null,
public val discontinuityTags: String? = null,
public val encryptionType: String? = null,
public val hlsCdnSettings: List? =
null,
public val hlsId3SegmentTagging: String? = null,
public val iframeOnlyPlaylists: String? = null,
public val incompleteSegmentBehavior: String? = null,
public val indexNSegments: Int? = null,
public val inputLossAction: String? = null,
public val ivInManifest: String? = null,
public val ivSource: String? = null,
public val keepSegments: Int? = null,
public val keyFormat: String? = null,
public val keyFormatVersions: String? = null,
public val keyProviderSettings: ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings? =
null,
public val manifestCompression: String? = null,
public val manifestDurationFormat: String? = null,
public val minSegmentLength: Int? = null,
public val mode: String? = null,
public val outputSelection: String? = null,
public val programDateTime: String? = null,
public val programDateTimeClock: String? = null,
public val programDateTimePeriod: Int? = null,
public val redundantManifest: String? = null,
public val segmentLength: Int? = null,
public val segmentsPerSubdirectory: Int? = null,
public val streamInfResolution: String? = null,
public val timedMetadataId3Frame: String? = null,
public val timedMetadataId3Period: Int? = null,
public val timestampDeltaMilliseconds: Int? = null,
public val tsFileMode: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings): ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings =
ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettings(
adMarkers = javaType.adMarkers().map({ args0 -> args0 }),
baseUrlContent = javaType.baseUrlContent().map({ args0 -> args0 }).orElse(null),
baseUrlContent1 = javaType.baseUrlContent1().map({ args0 -> args0 }).orElse(null),
baseUrlManifest = javaType.baseUrlManifest().map({ args0 -> args0 }).orElse(null),
baseUrlManifest1 = javaType.baseUrlManifest1().map({ args0 -> args0 }).orElse(null),
captionLanguageMappings = javaType.captionLanguageMappings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsCaptionLanguageMapping.Companion.toKotlin(args0)
})
}),
captionLanguageSetting = javaType.captionLanguageSetting().map({ args0 -> args0 }).orElse(null),
clientCache = javaType.clientCache().map({ args0 -> args0 }).orElse(null),
codecSpecification = javaType.codecSpecification().map({ args0 -> args0 }).orElse(null),
constantIv = javaType.constantIv().map({ args0 -> args0 }).orElse(null),
destination = javaType.destination().let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsDestination.Companion.toKotlin(args0)
}),
directoryStructure = javaType.directoryStructure().map({ args0 -> args0 }).orElse(null),
discontinuityTags = javaType.discontinuityTags().map({ args0 -> args0 }).orElse(null),
encryptionType = javaType.encryptionType().map({ args0 -> args0 }).orElse(null),
hlsCdnSettings = javaType.hlsCdnSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsHlsCdnSetting.Companion.toKotlin(args0)
})
}),
hlsId3SegmentTagging = javaType.hlsId3SegmentTagging().map({ args0 -> args0 }).orElse(null),
iframeOnlyPlaylists = javaType.iframeOnlyPlaylists().map({ args0 -> args0 }).orElse(null),
incompleteSegmentBehavior = javaType.incompleteSegmentBehavior().map({ args0 ->
args0
}).orElse(null),
indexNSegments = javaType.indexNSegments().map({ args0 -> args0 }).orElse(null),
inputLossAction = javaType.inputLossAction().map({ args0 -> args0 }).orElse(null),
ivInManifest = javaType.ivInManifest().map({ args0 -> args0 }).orElse(null),
ivSource = javaType.ivSource().map({ args0 -> args0 }).orElse(null),
keepSegments = javaType.keepSegments().map({ args0 -> args0 }).orElse(null),
keyFormat = javaType.keyFormat().map({ args0 -> args0 }).orElse(null),
keyFormatVersions = javaType.keyFormatVersions().map({ args0 -> args0 }).orElse(null),
keyProviderSettings = javaType.keyProviderSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputGroupSettingsHlsGroupSettingsKeyProviderSettings.Companion.toKotlin(args0)
})
}).orElse(null),
manifestCompression = javaType.manifestCompression().map({ args0 -> args0 }).orElse(null),
manifestDurationFormat = javaType.manifestDurationFormat().map({ args0 -> args0 }).orElse(null),
minSegmentLength = javaType.minSegmentLength().map({ args0 -> args0 }).orElse(null),
mode = javaType.mode().map({ args0 -> args0 }).orElse(null),
outputSelection = javaType.outputSelection().map({ args0 -> args0 }).orElse(null),
programDateTime = javaType.programDateTime().map({ args0 -> args0 }).orElse(null),
programDateTimeClock = javaType.programDateTimeClock().map({ args0 -> args0 }).orElse(null),
programDateTimePeriod = javaType.programDateTimePeriod().map({ args0 -> args0 }).orElse(null),
redundantManifest = javaType.redundantManifest().map({ args0 -> args0 }).orElse(null),
segmentLength = javaType.segmentLength().map({ args0 -> args0 }).orElse(null),
segmentsPerSubdirectory = javaType.segmentsPerSubdirectory().map({ args0 -> args0 }).orElse(null),
streamInfResolution = javaType.streamInfResolution().map({ args0 -> args0 }).orElse(null),
timedMetadataId3Frame = javaType.timedMetadataId3Frame().map({ args0 -> args0 }).orElse(null),
timedMetadataId3Period = javaType.timedMetadataId3Period().map({ args0 -> args0 }).orElse(null),
timestampDeltaMilliseconds = javaType.timestampDeltaMilliseconds().map({ args0 ->
args0
}).orElse(null),
tsFileMode = javaType.tsFileMode().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy