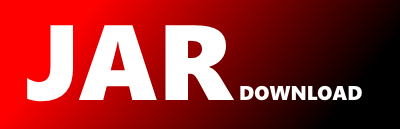
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-aws-kotlin Show documentation
Show all versions of pulumi-aws-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.aws.medialive.kotlin.outputs
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property absentInputAudioBehavior
* @property arib
* @property aribCaptionsPid
* @property aribCaptionsPidControl
* @property audioBufferModel
* @property audioFramesPerPes
* @property audioPids
* @property audioStreamType
* @property bitrate
* @property bufferModel
* @property ccDescriptor
* @property dvbNitSettings
* @property dvbSdtSettings
* @property dvbSubPids
* @property dvbTdtSettings
* @property dvbTeletextPid
* @property ebif
* @property ebpAudioInterval
* @property ebpLookaheadMs
* @property ebpPlacement
* @property ecmPid
* @property esRateInPes
* @property etvPlatformPid
* @property etvSignalPid
* @property fragmentTime
* @property klv
* @property klvDataPids
* @property nielsenId3Behavior
* @property nullPacketBitrate
* @property patInterval
* @property pcrControl
* @property pcrPeriod
* @property pcrPid
* @property pmtInterval
* @property pmtPid
* @property programNum
* @property rateMode
* @property scte27Pids
* @property scte35Control
* @property scte35Pid PID from which to read SCTE-35 messages.
* @property segmentationMarkers
* @property segmentationStyle
* @property segmentationTime
* @property timedMetadataBehavior
* @property timedMetadataPid
* @property transportStreamId
* @property videoPid
*/
public data class
ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings(
public val absentInputAudioBehavior: String? = null,
public val arib: String? = null,
public val aribCaptionsPid: String? = null,
public val aribCaptionsPidControl: String? = null,
public val audioBufferModel: String? = null,
public val audioFramesPerPes: Int? = null,
public val audioPids: String? = null,
public val audioStreamType: String? = null,
public val bitrate: Int? = null,
public val bufferModel: String? = null,
public val ccDescriptor: String? = null,
public val dvbNitSettings: ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings? =
null,
public val dvbSdtSettings: ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings? =
null,
public val dvbSubPids: String? = null,
public val dvbTdtSettings: ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings? =
null,
public val dvbTeletextPid: String? = null,
public val ebif: String? = null,
public val ebpAudioInterval: String? = null,
public val ebpLookaheadMs: Int? = null,
public val ebpPlacement: String? = null,
public val ecmPid: String? = null,
public val esRateInPes: String? = null,
public val etvPlatformPid: String? = null,
public val etvSignalPid: String? = null,
public val fragmentTime: Double? = null,
public val klv: String? = null,
public val klvDataPids: String? = null,
public val nielsenId3Behavior: String? = null,
public val nullPacketBitrate: Double? = null,
public val patInterval: Int? = null,
public val pcrControl: String? = null,
public val pcrPeriod: Int? = null,
public val pcrPid: String? = null,
public val pmtInterval: Int? = null,
public val pmtPid: String? = null,
public val programNum: Int? = null,
public val rateMode: String? = null,
public val scte27Pids: String? = null,
public val scte35Control: String? = null,
public val scte35Pid: String? = null,
public val segmentationMarkers: String? = null,
public val segmentationStyle: String? = null,
public val segmentationTime: Double? = null,
public val timedMetadataBehavior: String? = null,
public val timedMetadataPid: String? = null,
public val transportStreamId: Int? = null,
public val videoPid: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.aws.medialive.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings): ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings =
ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettings(
absentInputAudioBehavior = javaType.absentInputAudioBehavior().map({ args0 -> args0 }).orElse(null),
arib = javaType.arib().map({ args0 -> args0 }).orElse(null),
aribCaptionsPid = javaType.aribCaptionsPid().map({ args0 -> args0 }).orElse(null),
aribCaptionsPidControl = javaType.aribCaptionsPidControl().map({ args0 -> args0 }).orElse(null),
audioBufferModel = javaType.audioBufferModel().map({ args0 -> args0 }).orElse(null),
audioFramesPerPes = javaType.audioFramesPerPes().map({ args0 -> args0 }).orElse(null),
audioPids = javaType.audioPids().map({ args0 -> args0 }).orElse(null),
audioStreamType = javaType.audioStreamType().map({ args0 -> args0 }).orElse(null),
bitrate = javaType.bitrate().map({ args0 -> args0 }).orElse(null),
bufferModel = javaType.bufferModel().map({ args0 -> args0 }).orElse(null),
ccDescriptor = javaType.ccDescriptor().map({ args0 -> args0 }).orElse(null),
dvbNitSettings = javaType.dvbNitSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbNitSettings.Companion.toKotlin(args0)
})
}).orElse(null),
dvbSdtSettings = javaType.dvbSdtSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbSdtSettings.Companion.toKotlin(args0)
})
}).orElse(null),
dvbSubPids = javaType.dvbSubPids().map({ args0 -> args0 }).orElse(null),
dvbTdtSettings = javaType.dvbTdtSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.aws.medialive.kotlin.outputs.ChannelEncoderSettingsOutputGroupOutputOutputSettingsUdpOutputSettingsContainerSettingsM2tsSettingsDvbTdtSettings.Companion.toKotlin(args0)
})
}).orElse(null),
dvbTeletextPid = javaType.dvbTeletextPid().map({ args0 -> args0 }).orElse(null),
ebif = javaType.ebif().map({ args0 -> args0 }).orElse(null),
ebpAudioInterval = javaType.ebpAudioInterval().map({ args0 -> args0 }).orElse(null),
ebpLookaheadMs = javaType.ebpLookaheadMs().map({ args0 -> args0 }).orElse(null),
ebpPlacement = javaType.ebpPlacement().map({ args0 -> args0 }).orElse(null),
ecmPid = javaType.ecmPid().map({ args0 -> args0 }).orElse(null),
esRateInPes = javaType.esRateInPes().map({ args0 -> args0 }).orElse(null),
etvPlatformPid = javaType.etvPlatformPid().map({ args0 -> args0 }).orElse(null),
etvSignalPid = javaType.etvSignalPid().map({ args0 -> args0 }).orElse(null),
fragmentTime = javaType.fragmentTime().map({ args0 -> args0 }).orElse(null),
klv = javaType.klv().map({ args0 -> args0 }).orElse(null),
klvDataPids = javaType.klvDataPids().map({ args0 -> args0 }).orElse(null),
nielsenId3Behavior = javaType.nielsenId3Behavior().map({ args0 -> args0 }).orElse(null),
nullPacketBitrate = javaType.nullPacketBitrate().map({ args0 -> args0 }).orElse(null),
patInterval = javaType.patInterval().map({ args0 -> args0 }).orElse(null),
pcrControl = javaType.pcrControl().map({ args0 -> args0 }).orElse(null),
pcrPeriod = javaType.pcrPeriod().map({ args0 -> args0 }).orElse(null),
pcrPid = javaType.pcrPid().map({ args0 -> args0 }).orElse(null),
pmtInterval = javaType.pmtInterval().map({ args0 -> args0 }).orElse(null),
pmtPid = javaType.pmtPid().map({ args0 -> args0 }).orElse(null),
programNum = javaType.programNum().map({ args0 -> args0 }).orElse(null),
rateMode = javaType.rateMode().map({ args0 -> args0 }).orElse(null),
scte27Pids = javaType.scte27Pids().map({ args0 -> args0 }).orElse(null),
scte35Control = javaType.scte35Control().map({ args0 -> args0 }).orElse(null),
scte35Pid = javaType.scte35Pid().map({ args0 -> args0 }).orElse(null),
segmentationMarkers = javaType.segmentationMarkers().map({ args0 -> args0 }).orElse(null),
segmentationStyle = javaType.segmentationStyle().map({ args0 -> args0 }).orElse(null),
segmentationTime = javaType.segmentationTime().map({ args0 -> args0 }).orElse(null),
timedMetadataBehavior = javaType.timedMetadataBehavior().map({ args0 -> args0 }).orElse(null),
timedMetadataPid = javaType.timedMetadataPid().map({ args0 -> args0 }).orElse(null),
transportStreamId = javaType.transportStreamId().map({ args0 -> args0 }).orElse(null),
videoPid = javaType.videoPid().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy